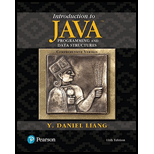
Program to display maximum consecutive increasingly ordered substring
Program Plan:
- Create the class “Exercise22_01”.
- In the main() function,
- Read the input object to read the input from user.
- Call the function maxConsecutivesSortedSubstring()to print the maximum ordered substring.
- In the maxConsecutivesSortedSubstring(),
- Initially assign the maximum length of the substring.
- Use for() loop to execute the whole length of the string.
- Check the position of character at “i” and “i-1” and compare the values.
- If it is lesser, assign the “i” to “current”.
- If the condition not satisfies, then increment the length of maximum consecutive length.
- Use for() loop to iterate the string values.
- Check whether the current length of string is less than the largest string.
- If yes, then assign the current element length to largest subsequence element.
- Construct the character sequence using while() loop at the end of the string.
- Return the string.
- Check the position of character at “i” and “i-1” and compare the values.
- In the maxConsecutivesSortedSubstring1(),
- Use the for loop to iterate the whole length of string.
- Check the position of character at “i” and “i-1” and compare the values.
- Return the sorted substring.
- Use the for loop to iterate the whole length of string.
This program reads the input from user and displays the maximum consecutive increasingly ordered substring.
Program:
//Declare the class Exercise22_01
public class Exercise22_01 {
//Declare the main function
public static void main(String[] args) {
//Create the input object
java.util.Scanner input = new
java.util.Scanner(System.in);
//Read the string
System.out.print("Enter a string: ");
String s = input.nextLine();
/*Call the function maxConsecutiveSortedSubstring() to print the maximum consecutive sorted substring*/
System.out.println("Maximum consecutive substring
is " + maxConsecutiveSortedSubstring(s));
}
/*Define the function maxConsecutiveSortedSubstring()*/
public static String
maxConsecutiveSortedSubstring(String s) {
//Declare the array and assign the length of array
int[] maxConsecutiveLength = new int[s.length()];
//Assign the variable as 0
int current = 0;
//Execute the for loop until the condition fails
for (int i = 1; i < s.length(); i++) {
/*Check whether the character is smaller than
the current character stored in string*/
if (s.charAt(i) <= s.charAt(i - 1)) {
//Assign the “i” value to current variable
current = i;
} else {
/*Execute the for loop until the condition fails*/
for (int j = i - 1; j >= current; j--)
//Increment the sequence of length
maxConsecutiveLength[j]++;
}
}
//Assign the length of sequence at index “i”
int currentMaxLength = maxConsecutiveLength[0];
int index = 0;
//Execute the for loop until the length of string
for (int i = 0; i < s.length(); i++) {
//Check whether the condition is true
if (maxConsecutiveLength[i] > currentMaxLength)
{
/*Assign the maximum sequence length to current length */
currentMaxLength = maxConsecutiveLength[i];
//Assign the index position
index = i;
}
}
//Return the substring
return s.substring(index, index + currentMaxLength + 1);
}
//Define the function for the sorted substring
public static String
maxConsecutiveSortedSubstring1(String s) {
//Assign the current length
int currentMaxLength = 1;
//Assign the last index of sorted substring
int lastIndexOfMaxConsecutiveSortedSubstring = 0;
//Assign the possible length
int possibleMaxLength = 1;
//Execute the for loop until it fails
for (int i = 1; i < s.length(); i++) {
//Check the position of the string
if (s.charAt(i) > s.charAt(i - 1)) {
//Check the condition
if
(lastIndexOfMaxConsecutiveSortedSubstri
ng == i - 1) {
/* Add the max consecutive substring*/
currentMaxLength++;
/*Add the index of max consecutive substring*/
lastIndexOfMaxConsecutiveSortedSub
string++;
//If condition not satisfies
} else {
//Increment the length
possibleMaxLength++;
//Check the condition
if (possibleMaxLength >
currentMaxLength) {
/*Assign the possible length to current maximum length*/
currentMaxLength =
possibleMaxLength;
/*Assign the index of the string*/
lastIndexOfMaxConsecutiveSort
edSubstring = i;
possibleMaxLength = 1;
}
}
}
}
//Return the sorted substring
return
s.substring(lastIndexOfMaxConsecutiveSortedSubstr
ing - currentMaxLength + 1,lastIndexOfMaxConsecutiveSortedSubstring + 1);
}
}
Running time complexity:
The above program executes in
Sample Output:
Enter a string: abcabcdgabmnsxy
Maximum consecutive substring is abmnsxy

Program to display maximum consecutive increasingly ordered substring
Program Plan:
- Create the class “Exercise22_01”.
- In the main() function,
- Read the input object to read the input from user.
- Call the function maxConsecutivesSortedSubstring()to print the maximum ordered substring.
- In the maxConsecutivesSortedSubstring(),
- Initially assign the maximum length of the substring.
- Use for() loop to execute the whole length of the string.
- Check the position of character at “i” and “i-1” and compare the values.
- If it is lesser, assign the “i” to “current”.
- If the condition not satisfies, then increment the length of maximum consecutive length.
- Use for() loop to iterate the string values.
- Check whether the current length of string is less than the largest string.
- If yes, then assign the current element length to largest subsequence element.
- Construct the character sequence using while() loop at the end of the string.
- Return the string.
- Check the position of character at “i” and “i-1” and compare the values.
- In the maxConsecutivesSortedSubstring1(),
- Use the for loop to iterate the whole length of string.
- Check the position of character at “i” and “i-1” and compare the values.
- Return the sorted substring.
- Use the for loop to iterate the whole length of string.
This program reads the input from user and displays the maximum consecutive increasingly ordered substring.
Explanation of Solution
Program:
//Declare the class Exercise22_01
public class Exercise22_01 {
//Declare the main function
public static void main(String[] args) {
//Create the input object
java.util.Scanner input = new
java.util.Scanner(System.in);
//Read the string
System.out.print("Enter a string: ");
String s = input.nextLine();
/*Call the function maxConsecutiveSortedSubstring() to print the maximum consecutive sorted substring*/
System.out.println("Maximum consecutive substring
is " + maxConsecutiveSortedSubstring(s));
}
/*Define the function maxConsecutiveSortedSubstring()*/
public static String
maxConsecutiveSortedSubstring(String s) {
//Declare the array and assign the length of array
int[] maxConsecutiveLength = new int[s.length()];
//Assign the variable as 0
int current = 0;
//Execute the for loop until the condition fails
for (int i = 1; i < s.length(); i++) {
/*Check whether the character is smaller than
the current character stored in string*/
if (s.charAt(i) <= s.charAt(i - 1)) {
//Assign the “i” value to current variable
current = i;
} else {
/*Execute the for loop until the condition fails*/
for (int j = i - 1; j >= current; j--)
//Increment the sequence of length
maxConsecutiveLength[j]++;
}
}
//Assign the length of sequence at index “i”
int currentMaxLength = maxConsecutiveLength[0];
int index = 0;
//Execute the for loop until the length of string
for (int i = 0; i < s.length(); i++) {
//Check whether the condition is true
if (maxConsecutiveLength[i] > currentMaxLength)
{
/*Assign the maximum sequence length to current length */
currentMaxLength = maxConsecutiveLength[i];
//Assign the index position
index = i;
}
}
//Return the substring
return s.substring(index, index + currentMaxLength + 1);
}
//Define the function for the sorted substring
public static String
maxConsecutiveSortedSubstring1(String s) {
//Assign the current length
int currentMaxLength = 1;
//Assign the last index of sorted substring
int lastIndexOfMaxConsecutiveSortedSubstring = 0;
//Assign the possible length
int possibleMaxLength = 1;
//Execute the for loop until it fails
for (int i = 1; i < s.length(); i++) {
//Check the position of the string
if (s.charAt(i) > s.charAt(i - 1)) {
//Check the condition
if
(lastIndexOfMaxConsecutiveSortedSubstri
ng == i - 1) {
/* Add the max consecutive substring*/
currentMaxLength++;
/*Add the index of max consecutive substring*/
lastIndexOfMaxConsecutiveSortedSub
string++;
//If condition not satisfies
} else {
//Increment the length
possibleMaxLength++;
//Check the condition
if (possibleMaxLength >
currentMaxLength) {
/*Assign the possible length to current maximum length*/
currentMaxLength =
possibleMaxLength;
/*Assign the index of the string*/
lastIndexOfMaxConsecutiveSort
edSubstring = i;
possibleMaxLength = 1;
}
}
}
}
//Return the sorted substring
return
s.substring(lastIndexOfMaxConsecutiveSortedSubstr
ing - currentMaxLength + 1,lastIndexOfMaxConsecutiveSortedSubstring + 1);
}
}
Running time complexity:
The above program executes in
is
Enter a string: abcabcdgabmnsxy
Maximum consecutive substring is abmnsxy
Want to see more full solutions like this?
Chapter 22 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
SURVEY OF OPERATING SYSTEMS
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
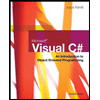
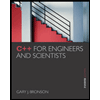
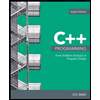
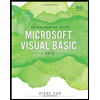
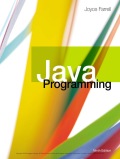