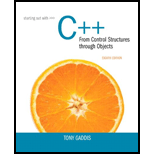
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 20, Problem 4PC
Program Plan Intro
Height of the Binary Tree
Program Plan:
- Create a template prefix and define the template class BinaryTree to perform the following functions:
- Declare the required variables.
- Declare the function prototypes.
- Define the no-argument generic constructor BinaryTree() to initialize the root value as null.
- Call the functions insertNode(), remove(), displayInOrder(), and treeHeight().
- Define the generic function insert() to insert the node in position pointed by the tree node pointer in a tree.
- Define the generic function insertNode() to create a new node and it should be passed inside the insert() function to insert a new node into the tree.
- Define the generic function remove()which calls deleteNode() to delete the node.
- Define the generic function deleteNode() which deletes the node stored in the variable num and it calls the makeDeletion() function to delete a particular node passed inside the argument.
- Define the generic function makeDeletion()which takes the reference to a pointer to delete the node and the brances of the tree corresponding below the node are reattached.
- Define the generic function displayInOrder()to display the values in the subtree pointed by the node pointer.
- Define the generic function getTreeHeight() to count the height of the tree.
- Define the generic function TreeHeight()which calls getTreeHeight() to display the height of the tree.
- In main() function,
- Create a tree with integer data type to hold the integer values.
- Call the function treeHeight() to print the initial height of the tree.
- Call the function insertNode() to insert the node in a tree.
- Call the function displayInOrder() to display the nodes inserted in the order.
- Call the function remove() to remove the nodes from tree.
- Call the function treeHeight() to print the height of the tree after deleting the nodes.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Refer to page 75 for graph-related problems.
Instructions:
• Implement a greedy graph coloring algorithm for the given graph.
• Demonstrate the steps to assign colors while minimizing the chromatic number.
•
Analyze the time complexity and limitations of the approach.
Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]
Refer to page 150 for problems on socket programming.
Instructions:
• Develop a client-server application using sockets to exchange messages.
•
Implement both TCP and UDP communication and highlight their differences.
• Test the program under different network conditions and analyze results.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]
Refer to page 80 for problems on white-box testing.
Instructions:
•
Perform control flow testing for the given program, drawing the control flow graph (CFG).
• Design test cases to achieve statement, branch, and path coverage.
• Justify the adequacy of your test cases using the CFG.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]
Chapter 20 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 20.1 - Prob. 21.1CPCh. 20.1 - Prob. 21.2CPCh. 20.1 - Prob. 21.3CPCh. 20.1 - Prob. 21.4CPCh. 20.1 - Prob. 21.5CPCh. 20.1 - Prob. 21.6CPCh. 20.2 - Prob. 21.7CPCh. 20.2 - Prob. 21.8CPCh. 20.2 - Prob. 21.9CPCh. 20.2 - Prob. 21.10CP
Ch. 20.2 - Prob. 21.11CPCh. 20.2 - Prob. 21.12CPCh. 20 - Prob. 1RQECh. 20 - Prob. 2RQECh. 20 - Prob. 3RQECh. 20 - Prob. 4RQECh. 20 - Prob. 5RQECh. 20 - Prob. 6RQECh. 20 - Prob. 7RQECh. 20 - Prob. 8RQECh. 20 - Prob. 9RQECh. 20 - Prob. 10RQECh. 20 - Prob. 11RQECh. 20 - Prob. 12RQECh. 20 - Prob. 13RQECh. 20 - Prob. 14RQECh. 20 - Prob. 15RQECh. 20 - Prob. 16RQECh. 20 - Prob. 17RQECh. 20 - Prob. 18RQECh. 20 - Prob. 19RQECh. 20 - Prob. 20RQECh. 20 - Prob. 21RQECh. 20 - Prob. 22RQECh. 20 - Prob. 23RQECh. 20 - Prob. 24RQECh. 20 - Prob. 25RQECh. 20 - Prob. 1PCCh. 20 - Prob. 2PCCh. 20 - Prob. 3PCCh. 20 - Prob. 4PCCh. 20 - Prob. 5PCCh. 20 - Prob. 6PCCh. 20 - Prob. 7PCCh. 20 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Refer to page 10 for problems on parsing. Instructions: • Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)). • Compute the FIRST and FOLLOW sets and construct the parsing table if applicable. • Parse a sample input string and explain the derivation step-by-step. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 20 for problems related to finite automata. Instructions: • Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the given language. • Minimize the DFA and show all steps, including state merging. • Verify that the automaton accepts the correct language by testing with sample strings. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forwardRefer to page 60 for solving the Knapsack problem using dynamic programming. Instructions: • Implement the dynamic programming approach for the 0/1 Knapsack problem. Clearly define the recurrence relation and show the construction of the DP table. Verify your solution by tracing the selected items for a given weight limit. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 70 for problems related to process synchronization. Instructions: • • Solve a synchronization problem using semaphores or monitors (e.g., Producer-Consumer, Readers-Writers). Write pseudocode for the solution and explain the critical section management. • Ensure the solution avoids deadlock and starvation. Test with an example scenario. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward15 points Save ARS Consider the following scenario in which host 10.0.0.1 is communicating with an external SMTP mail server at IP address 128.119.40.186. NAT translation table WAN side addr LAN side addr (c), 5051 (d), 3031 S: (e),5051 SMTP B D (f.(g) 10.0.0.4 server 138.76.29.7 128.119.40.186 (a) is the source IP address at A, and its value. S: (a),3031 D: (b), 25 10.0.0.1 A 10.0.0.2. 1. 138.76.29.7 10.0.0.3arrow_forward6.3A-3. Multiple Access protocols (3). Consider the figure below, which shows the arrival of 6 messages for transmission at different multiple access wireless nodes at times t=0.1, 1.4, 1.8, 3.2, 3.3, 4.1. Each transmission requires exactly one time unit. 1 t=0.0 2 3 45 t=1.0 t-2.0 t-3.0 6 t=4.0 t-5.0 For the CSMA protocol (without collision detection), indicate which packets are successfully transmitted. You should assume that it takes .2 time units for a signal to propagate from one node to each of the other nodes. You can assume that if a packet experiences a collision or senses the channel busy, then that node will not attempt a retransmission of that packet until sometime after t=5. Hint: consider propagation times carefully here. (Note: You can find more examples of problems similar to this here B.] ☐ U ப 5 - 3 1 4 6 2arrow_forward
- Just wanted to know, if you had a scene graph, how do you get multiple components from a specific scene node within a scene graph? Like if I wanted to get a component from wheel from the scene graph, does that require traversing still? Like if a physics component requires a transform component and these two component are part of the same scene node. How does the physics component knows how to get the scene object's transform it is attached to, this being in a scene graph?arrow_forwardHow to develop a C program that receives the message sent by the provided program and displays the name and email included in the message on the screen?Here is the code of the program that sends the message for reference: typedef struct { long tipo; struct { char nome[50]; char email[40]; } dados;} MsgStruct; int main() { int msg_id, status; msg_id = msgget(1000, 0600 | IPC_CREAT); exit_on_error(msg_id, "Creation/Connection"); MsgStruct msg; msg.tipo = 5; strcpy(msg.dados.nome, "Pedro Silva"); strcpy(msg.dados.email, "pedro@sapo.pt"); status = msgsnd(msg_id, &msg, sizeof(msg.dados), 0); exit_on_error(status, "Send"); printf("Message sent!\n");}arrow_forward9. Let L₁=L(ab*aa), L₂=L(a*bba*). Find a regular expression for (L₁ UL2)*L2. 10. Show that the language is not regular. L= {a":n≥1} 11. Show a derivation tree for the string aabbbb with the grammar S→ABλ, A→aB, B→Sb. Give a verbal description of the language generated by this grammar.arrow_forward
- 14. Show that the language L= {wna (w) < Nь (w) < Nc (w)} is not context free.arrow_forward7. What language is accepted by the following generalized transition graph? a+b a+b* a a+b+c a+b 8. Construct a right-linear grammar for the language L ((aaab*ab)*).arrow_forward5. Find an nfa with three states that accepts the language L = {a^ : n≥1} U {b³a* : m≥0, k≥0}. 6. Find a regular expression for L = {vwv: v, wЄ {a, b}*, |v|≤4}.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
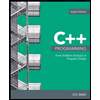
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning