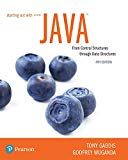
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 20, Problem 2MC
Program Description Answer
A stack is a collection of items which are accessed in last-in-first-out fashion.
Hence, the correct answer is option “A”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
TOPICS: LIST/STACK/QUEUE
Write a complete Java program about Appointment
schedule(anything). Your program must implements the
linked list The program should have the following basic
operations of list, which are:
a) Add first, in between and last
b) Delete first, in between and last
c) Display all data
The program should be an interactive program that allow
user to choose type of operation.
URGENT URGENT URGENT !!!!
Write a void method swapStackwithQueue that takes MyStack and MyQueue Objects as parameters and exchanges the elements.
a) The top element of the old stack becomes the rear element of the new queue. b
b) and the rear element of the old queue becomes the top element of the new stack.
The output should be similar to the image.
class Node: def __init__(self, e, n): self.element = e self.next = n
class LinkedList: def __init__(self, a): # Design the constructor based on data type of a. If 'a' is built in python list then # Creates a linked list using the values from the given array. head will refer # to the Node that contains the element from a[0] # Else Sets the value of head. head will refer # to the given LinkedList
# Hint: Use the type() function to determine the data type of a self.head = None # To Do # Count the number of nodes in the list def countNode(self): # To Do # Print elements in the list def printList(self): # To Do
# returns the reference of the Node at the given index. For invalid index return None. def nodeAt(self, idx): # To Do
Chapter 20 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 20.3 - Prob. 20.1CPCh. 20.3 - Prob. 20.2CPCh. 20.3 - Prob. 20.4CPCh. 20.3 - Prob. 20.5CPCh. 20.6 - Prob. 20.6CPCh. 20.6 - Prob. 20.7CPCh. 20.6 - Prob. 20.8CPCh. 20.6 - Prob. 20.9CPCh. 20 - Prob. 1MCCh. 20 - Prob. 2MC
Ch. 20 - Prob. 3MCCh. 20 - The concept of seniority, which some employers use...Ch. 20 - Prob. 5MCCh. 20 - Prob. 6MCCh. 20 - Prob. 8TFCh. 20 - Prob. 9TFCh. 20 - Prob. 10TFCh. 20 - Prob. 1FTECh. 20 - Prob. 2FTECh. 20 - Prob. 3FTECh. 20 - Prob. 4FTECh. 20 - Prob. 5FTECh. 20 - Prob. 1AWCh. 20 - Prob. 2AWCh. 20 - Suppose that you have two stacks but no queues....Ch. 20 - Prob. 1SACh. 20 - Prob. 2SACh. 20 - Prob. 3SACh. 20 - Prob. 4SACh. 20 - Prob. 5SACh. 20 - Prob. 6SA
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Description The aim of this project is to develop a cyclic doubly linked list. You will implement two classes: 1. Cyclic doubly linked lists: CyclicDoubleList, and 2. Doubly linked nodes: DoubleNode. A cyclic doubly linked list with three nodes is shown in Figure 1. The empty cyclic doubly linked list is shown in Figure 2. head. Figure 1. A cyclic doubly linked list and three nodes. head- Figure 2. An empty cyclic doubly linked list. The class CyclicDoubleList stores a finite list of n (zero or more) elements stored in doubly linked nodes. If there are zero elements in the list, the list is said to be empty. Each element is stored in an instance of the DoubleNode class. If the list is empty, the head pointer points to null. Otherwise, the head pointer points to the first node, the next pointer of the ith node (1 referred to as the head pointer, and An integer referred to as the list size which equals the number of elements in the list. Member Functions Constructors CyclicDoubleList ()…arrow_forwardDon't copy from anywhere... please fast... typed answer Assignment: Linked List of Students You have been tasked with implementing a program in Java that uses a linked list to store and manage a list of students in a class. Each student should have a name and a grade. Your program should include the following classes: Student: Represents a student in the class. Each student should have a name and a grade. Node: Represents a node in the linked list. Each node should store a reference to a student and a reference to the next node in the list. LinkedList: Represents the linked list itself. Each linked list should have a reference to the first node in the list. Your task is to implement these classes using a linked list and demonstrate their functionality by creating a console-based interface for users to interact with the system. Your program should allow users to: Add a new student to the class at the end of the list. View information about a student, including their name and grade.…arrow_forwardlinked list is an object that creates, references and manipulates node objects. In this assignment, you are asked to write a Python program to create a linked list and do a set of operations as follows:1. Create an empty linked list2. Create and insert a new node at the front of the linked list3. Insert a new node at the back of the linked list4. Insert a new node at a specified position in the linked list5. Get a copy of the data in the node at the front of the linked list6. Get a copy of the data in the node at a specified position in the linked list7. Remove the node at the front of the linked list8. Remove the node at the back of the linked list9. Remove the node at a specified position in the linked list10.Traverse the list to display all the data in the nodes of the linked list11.Check whether the linked list is empty12.Check whether the linked list is full13.Find a node of the linked list that contains a specified data itemThese operations can be implemented as methods in a…arrow_forward
- Please code in C language. Please use the starter code to help you solve the deleted node and the reverse list. Here is the starter code: #include <stdio.h> #include <ctype.h> #include <stdlib.h> #include <string.h> #include "linkedlist.h" // print an error message by an error number, and return // the function does not exit from the program // the function does not return a value void error_message(enum ErrorNumber errno) { char *messages[] = { "OK", "Memory allocaton failed.", "Deleting a node is not supported.", "The number is not on the list.", "Sorting is not supported.", "Reversing is not supported.", "Token is too long.", "A number should be specified after character d, a, or p.", "Token is not recognized.", "Invalid error number."}; if (errno < 0 || errno > ERR_END) errno = ERR_END; printf("linkedlist: %s\n", messages[errno]); } node *new_node(int v) { node *p =…arrow_forward6. Suppose that we have defined a singly linked list class that contains a list of unique integers in ascending order. Create a method that merges the integers into a new list. Note the additional requirements listed below. Notes: ● . Neither this list nor other list should change. The input lists will contain id's in sorted order. However, they may contain duplicate values. For example, other list might contain id's . You should not create duplicate id's in the list. Important: this list may contain duplicate id's, and other list may also contain duplicate id's. You must ensure that the resulting list does not contain duplicates, even if the input lists do contain duplicates.arrow_forwardC# Assume you have a LinkedList of Node objects. Both classes have all the normal operations shown below. Your job is to program the DeleteTail method of the LinkedList class. This method locates and deletes the last element of the linked list. You may not change its signature line. Keep your code clean, but no documentation is necessary. A good solution will be between 5 and 10 lines of code, not counting whitespace.arrow_forward
- It is a to the next node in the linked list, which is the property after it in the Node class.arrow_forwardStack can be implemented using _________ and ________ ?(java) a. Array and Binary Tree b. Linked List and Graph c. Queue and Linked List d. Array and Linked Listarrow_forward9 T OR F Depending on the circumstances, the dequeue method of our LinkedQueue class sometimes throws the QueueUnderflowException.arrow_forward
- In Javaarrow_forwardThe tail of a linked list is distinguished from other nodes because its next pointer is:A. void B. empty C. NULL D. None of the above.arrow_forwardWrite a program that creates a linked list to represent details of students. • SID (for Student ID) • Name • Address • Age • Gender Your program must also display a menu to perform the following tasks: • Create a linked list. The method to create a linked list makes use of the method insertFirst() or insertLast() or insertOrdered() • Insert new nodes to the linked list. • Search for details pertaining to a particular student. • Delete details of a particular student. • Find number of nodes in the linked list. • Display information pertaining to all students. The menu options should be as follows: 1. Create linked list 2. Add new student 3. Search for a student 4. Delete a student 5. Find number of students 6. Print student details 7. Exitarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
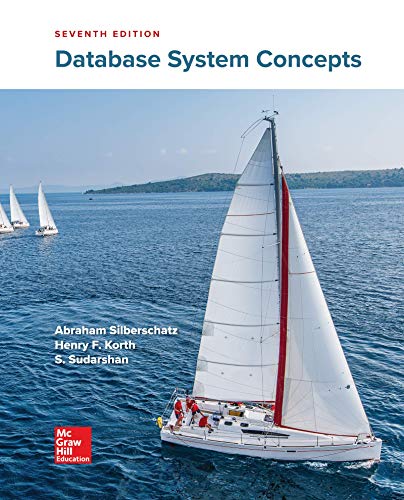
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
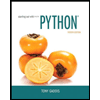
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
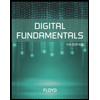
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
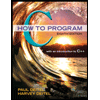
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
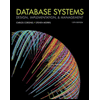
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
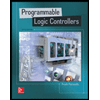
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education