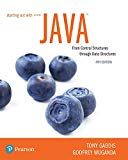
Concept explainers
Explanation of Solution
The given program is used to implement queue using linked list.
Syntax error:
An error that occurs on the source code of a program is referred as a “syntax error”, because the computer programs strictly follow the syntax rules. If the code fails to prove its language syntax format, then the compiler will throw an error.
Error in the code:
The operator “++” cannot be applied to a reference. Use front = front.next;
Correct statement:
/*removing the element from front by moving front to the next node.*/
front= front.next;
Corrected code:
//A linked implementation of a queue
int dequeue()
{
//checks whether the queue is empty
if(empty())
/*throws exception EmptyQueueException indicating the queue is empty*/
throw new EmptyQueueException();
/*if the queue is not empty then,
front element is reserved to a variable*/
int value= front...

Want to see the full answer?
Check out a sample textbook solution
Chapter 20 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- If N represents the number of elements in the queue, then the dequeue method of the LinkedQueue class is O(N). true or falsearrow_forwardThe QueueInterface interface is a contract between a Queue ADT's implementer and the programmer who uses it.is it true or falsearrow_forwardpackage queuedemo; class Queue { int front, rear, size; int capacity; int array[]; public Queue(int cap) { capacity = cap; front=size=0; rear=capacity-1; array = new int[capacity]; } // Method to add an item to the queue. void enqueue(int item) { if(isFull()) { System.out.println("Queue overflow"); return; } rear=(rear+1)%capacity; array[rear]=item; size=size+1; } // Queue is empty when size is 0 boolean isEmpty() { return (size == 0); } // Queue is full when size becomes equal to the capacity boolean isFull() { return (size == capacity); } // Method to remove an item from queue. int dequeue() { if (isEmpty()) System.out.println("Queue Underflow"); return; } int item = array[front]; front = (front + 1)% this.capacity;…arrow_forward
- By using this Queue class: public class Queue {private int rear, front;private Object[] elements;Queue(int capacity){elements = new Object[capacity];rear = -1;front = 0;}void enqueue(Object data){if(isFull()){System.out.println("Queue overflow");}else {rear = (rear +1) % elements.length;elements[rear] = data;}}Object dequeue(){if(isEmpty()){System.out.println("Queue empty");return null;}else {Object retData = elements[front];elements[front]= null;front = (front+1) % elements.length;return retData;}}Object peek(){if (isEmpty()){System.out.println("Queue is empty");return null;}else {return elements[front];}}boolean isEmpty(){return elements[front] == null;}boolean isFull(){return (front == (rear +1)% elements.length &&elements[front] != null&& elements[rear] != null);}int size(){if (rear >= front){return rear - front +1;}else if (elements[front] != null) {return elements.length - (front - rear) + 1;}else {return 0;}}}arrow_forwardNote : addqueue works like Enqueue and deleteQueue works like Dequeue Consider the following statements: (8, 9) queueType queue; int num; Show what is output by the following segment of code num = 7; queue.addQueue (6); queue.addQueue (num); num = queue.front (); queue.deleteQueue(); queue.addQueue (num + 5); queue.addQueue (14); queue.addQueue (num queue.addQueue (25); queue.deleteQueue (); 2); cout <« "Queue elements: "; while (!queue.isEmptyQueue ()) { cout <« queue.front () << " "; queue.deleteQueue(); } cout <« endl; Queue elements: 14 14 4 25 Queue elements: 11 14 4 4 Queue elements: 11 14 4 25 Queue elements: 11 14 25 25arrow_forwardIf N represents the number of elements in the queue, then the enqueue method of the ArrayBoundedQueue class is O(N). true or falsearrow_forward
- In a queue implementation using array of size 5, the array index starts with 0 where head and tail values are 3 and 4 respectively. Determine the array index at which the insertion of the next element will take place. 0 5 1 2arrow_forwardclass Queue { private static int front, rear, capacity; private static int queue[]; Queue(int size) { front = rear = 0; capacity = size; queue = new int[capacity]; } // insert an element into the queue static void queueEnqueue(int item) { // check if the queue is full if (capacity == rear) { System.out.printf("\nQueue is full\n"); return; } // insert element at the rear else { queue[rear] = item; rear++; } return; } //remove an element from the queue static void queueDequeue() { // check if queue is empty if (front == rear) { System.out.printf("\nQueue is empty\n"); return; } // shift elements to the right by one place uptil rear else {…arrow_forwardThe QueueInterface interface represents a contract between the implementer of a Queue ADT and the programmer who uses the ADT. true or falsearrow_forward
- A(n) array can be used in an array implementation of a queue to avoid an overflow error at the rear of the queue when the queue is not full.arrow_forwardThe essential condition which is checked before deletion in a linked queue is? a) Underflow b) Overflow c) Front value d) Rear valuearrow_forwardThe QueueInterface interface is an agreement between the implementer of a Queue ADT and the developer using that ADT. Thus, the question is: true or false?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
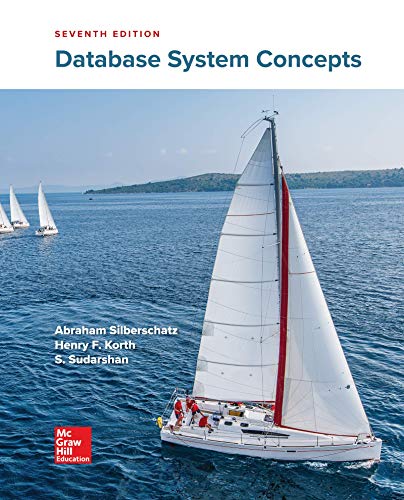
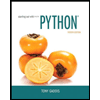
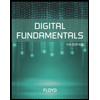
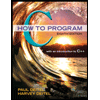
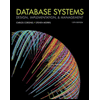
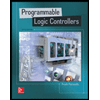