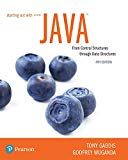
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 20, Problem 2AW
Program Plan Intro
Stack:
- A stack is a linear data structure.
- It follows the order FILO (first in last out) LIFO or (last-in-first-out) for operations on it.
- The two main operations performed on a stack are,
- Push() – Add item to stack.
- Pop() – Remove item from stack.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Java Programming
Define a class CollectionBooks. This class has a data member list of type Book using the ArrayList collection.
Define method add. This method add the any object to list.
Define printAll. This method display all the added object in the list.
Define printAll. This method display all the added object in the list. · Define int count. This method returns the number of objects added in the list.
Define Book search(Object e). This method returns the object being search if not found return null.
Define void remove(int index). This method remove the object in a list
Add a main method with the following menu:1 – Add 2 – Count 3 – Print4 – Search 5 – Delete 6 - Exit
in java
JAVA programming question
i will attach images of the ourQueue code and the main
I have to do the following:
*create an ourQueue object, add the following sequence:
*2,-3,4,-6,7,8,-9,-11
*create and ourStack object
*write a program/ one loop that would result into the stack containing all of the negative number at the bottom and the positive numbers at the top.
*outStack at the end should contain the following
*[-3,-6,-9,-11,2,4,7,8]
Chapter 20 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 20.3 - Prob. 20.1CPCh. 20.3 - Prob. 20.2CPCh. 20.3 - Prob. 20.4CPCh. 20.3 - Prob. 20.5CPCh. 20.6 - Prob. 20.6CPCh. 20.6 - Prob. 20.7CPCh. 20.6 - Prob. 20.8CPCh. 20.6 - Prob. 20.9CPCh. 20 - Prob. 1MCCh. 20 - Prob. 2MC
Ch. 20 - Prob. 3MCCh. 20 - The concept of seniority, which some employers use...Ch. 20 - Prob. 5MCCh. 20 - Prob. 6MCCh. 20 - Prob. 8TFCh. 20 - Prob. 9TFCh. 20 - Prob. 10TFCh. 20 - Prob. 1FTECh. 20 - Prob. 2FTECh. 20 - Prob. 3FTECh. 20 - Prob. 4FTECh. 20 - Prob. 5FTECh. 20 - Prob. 1AWCh. 20 - Prob. 2AWCh. 20 - Suppose that you have two stacks but no queues....Ch. 20 - Prob. 1SACh. 20 - Prob. 2SACh. 20 - Prob. 3SACh. 20 - Prob. 4SACh. 20 - Prob. 5SACh. 20 - Prob. 6SA
Knowledge Booster
Similar questions
- You will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forwardURGENT URGENT URGENT !!!! Write a void method swapStackwithQueue that takes MyStack and MyQueue Objects as parameters and exchanges the elements. a) The top element of the old stack becomes the rear element of the new queue. b b) and the rear element of the old queue becomes the top element of the new stack. The output should be similar to the image.arrow_forwardThe implementation of a queue in an array, as given in this chapter, uses the variable count to determine whether the queue is empty or full. You can also use the variable count to return the number of elements in the queue. On the other hand, class linkedQueueType does not use such a variable to keep track of the number of elements in the queue. Redefine the class linkedQueueType by adding the variable count to keep track of the number of elements in the queue. Modify the definitions of the functions addQueue and deleteQueue as necessary. Add the function queueCount to return the number of elements in the queue. Also, write a program to test various operations of the class you defined.arrow_forward
- Create an interface called Stack with methods Push (), Pop () and property Length.Create a class that implements this interface. Use an array to implement stack datastructure. language C#arrow_forwardC# Create class IntegerSet. Each IntegerSet object can hold integers in the range 0–100. The set is represented by an array of bools. Array element a[i] is true if integer i is in the set. Array element a[j] is false if integer j is not in the set. The parameterless constructor initializes the array to the “empty set” (i.e., a set whose array representation contains all false values.) • Provide the following methods: a) Method Union creates a third set that’s the set-theoretic union of two existing sets (i.e., an element of the third set’s array is set to true if that element is true in either or both of the existing sets—otherwise, the element of the third set is set to false). b) Method Intersection creates a third set which is the set-theoretic intersection of two existing sets (i.e., an element of the third set’s array is set to false if that element is false in either or both of the existing sets—otherwise, the element of the third set is set to true). c) Method…arrow_forwardC# Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function. This would occur in the NumberStack class, not the main class. These are the provided variables: private int [] stack;private int size; Create a method that will reverse the stack when put into the main class.arrow_forward
- Assume s1,s2 are two stacks and we write s1=s2 , if the class stack based on array then إختر أحد الخيارات: a. This statement is good to use when s1 has small number of items b. This statement is good to use when s2 has large number of items c. This statement is good to use when s2 has small number of items d. This statement is good to use when s1 has large number of items أخلِ اختياريarrow_forwardStack: Stacks are a type of container with LIFO (Last In First Out) type of working, where a new element is added at one end and (top) an element is removed from that end only. Your Stack should not be of the fixed sized. It should be able to grow itself. So using the class made in task 1, make a class named as Stack, having following additional functionalities: bool empty() : Returns whether the Stack is empty or not. Time Complexity should be: O(1) bool full() : Returns whether the Stack is full or not. Time Complexity should be: O(1)int size() : Returns the current size of the Stack. Time Complexity should be: O(1)Type top () : Returns the last element of the Stack. Time Complexity should be: O(1) void push(Type) : Adds the element of type Type at the top of the stack. Time Complexity should be: O(1) Type pop() : Deletes the top most element of the stack and returns it. Time Complexity should be: O(1) Write non-parameterized constructor for the above class. Write Copy…arrow_forward6. Given a Stack of holding 7 objects. Show the final contents of the array after the following code is executed: for (int k = 1; k <= 7; k++) S.Push(k); for (int k = 1; k<= 4; k++) { S.Push(S.Pop()); S.Pop(); }arrow_forward
- If the elements “A”, “B”, “C” and “D” are placed in a stack and are removed one at a time, in what order will they be removed?arrow_forwardOCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly and provide the code with the screenshot of the output. The code must have a function that takes a pair of strings (tuple) and returns a unit. You must avoid this error that is attached as an image. Make sure to have the following methods below: -Push integers, strings, and names on the stack -Push booleans -Pushing an error literal or unit literal will push :error: or :unit:onto the stack, respectively -Command pop removes the top value from the stack -The command add refers to integer addition. Since this is a binary operator, it consumes the toptwo values in the stack, calculates the sum and pushes the result back to the stack - Command sub refers to integer subtraction -Command mul refers to integer multiplication -Command div refers to integer division -Command rem refers to the remainder of integer…arrow_forwardDesign and implement an application that reads a sentence fromthe user and prints the sentence with the characters of each wordbackwards. Use a stack to reverse the characters of each word.8. Pop the infix expression off the stack and print it.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
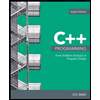
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning