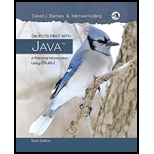
Add a further field, refNumber, to the Book class. This field can store a reference number for a library, for example. It should be of type String and initialized to the zero length string ("") in the constructor, as its initial value is not passed in a parameter to the constructor. Instead, define a mutator for it with the following header:
public void setRefNumber (String ref)
The body of this method should assign the value of the parameter to the refNumber field. Add a corresponding getRefNumber accessor to help you check that the mutator works correctly.

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Concepts Of Programming Languages
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- Implement the class Character The role should be “primary” or “secondary” all other values are not acceptable. Considercapital and small letters variance. The gender should be “m” or “M” for male or “f” or “F” for female. The age should be a positive value. The giveRaise method increase the salary of a character by a given percentage with amaximum salary of 10000. The toString method returns a string that describes the character. For example: [Elisabeth Kane, female, 35, primary,4500$] 2) Implement the class Play A Play can have at most 20 characters. The real number of characters is registered in nbOfCharacters and is updated automaticallywhen a new character is added. AddCharacter method adds a new character to the characters array and updates thenbOfCharacters value. The attribute nbPlay keeps track of the number of Plays created. The toString method returns a string description of the play including name, genre and thenames of all the characters.Example: [The Opera…arrow_forwardChallenge exercise The following object creation will result in the constructor of the Date class being called. Can you write the constructor’s header? new Date("March", 23, 1861) Try to give meaningful names to the parametersarrow_forwardDynamic Apex: Write test class for this apex code(Not required the output) You are working on the project in which you have to create the apex code in which your task is to define the objects dynamically by using the method argument which is api name in string and a map of string, string as a key value pair. Class Code: public static void modifySobjectData(String str , Map mapofString) { //Retrive the type of Sobject by using the getGlobalDescribe Schema.SobjectType targetType = Schema.getGlobalDescribe().get(str); //Initalize the Object Sobject newobject = targetType.newSobject(); for (String s :mapofString.keySet() { newobject.put(s, mapofString.get(s)); } System.debug('newObject' + newobject); //Perform the DML if(targetType != null) insert newobject; } }arrow_forward
- Exercise 2. Create a Book class where: Each book contains the following information: book title book Author name, barcode (as fong integers), and book topic. o Implement an appropriate constructor(s) and all necessary get/set methods. Test Book class: o Create diffcrent book objects (at least 5 hooks) and store them in a LinkedList sorted by book barcode value Generate a unique random integer value for the barcode. Iterate through the LinkedList and print out the books' details O Create a second LinkedList object containing a copy of the above LinkedList, but in reverse order.arrow_forwardA for construct is a loop that goes over a list of objects. Consequently, it runs indefinitely if there are items to process. What do you think about this?arrow_forwardWrite Junit test class that Constructs a new quarter. public Quarter(int quarter, int year) Parameters:year - the year (1900 to 9999).quarter - the quarter (1 to 4).arrow_forward
- Design a class named CustomerRecord that holds a customer number, name, and address. Include separate methods to 'set' the value for each data field using the parameter being passed. Include separate methods to 'get' the value for each data field, i.e. "return" the field's value. Pseudocode:arrow_forwardComplete the convert() method that casts the parameter from a double to an integer and returns the res Note that the main() method prints out the returned value of the convert() method. Ex: If the double value is 19.9, then the output is: 19 Ex: If the double value is 3.1, then the output is: 3 512334.3517088.qx3zqy7 LAB ACTIVITY 1 public class LabProgram { INM + ∞ 2 25.20.1: LAB: Write convert() method to cast double to int 3 public static int convert (double d) { /* Type your code here */ 4 5 6 7 } 8 public static void main(String[] args) { 9 System.out.println (convert (19.9)); 10 11 12} } System.out.println(convert(3.1)); LabProgram.javaarrow_forwardModify the student class presented in this chapter as follows. Each student object should also contain the scores for three tests. Provide a constructor that sets all instance values based on parameter values. Overload the constructor such that each test score is assumed to be initially zero. Provide a method called setTestScore that accepts two parameters: the test number (1 through 3) and the score. Also provide a method called getTestScore that accepts the test number and returns the appropriate score Provide a method called average that computes and returns the average test score for this student. Modify the toString method such that the test scores and average are included in the description of the student. Modify the driver class main method to exercise the new Student methods. (Java)arrow_forward
- Modify the student class presented in this chapter as follows. Each student object should also contain the scores for three tests. Provide a constructor that sets all instance values based on parameter values. Overload the constructor such that each test score is assumed to be initially zero. Provide a method called setTestScore that accepts two parameters: the test number (1 through 3) and the score. Also provide a method called getTestScore that accepts the test number and returns the appropriate score Provide a method called average that computes and returns the average test score for this student. Modify the toString method such that the test scores and average are included in the description of the student. Modify the driver class main method to exercise the new Student methods. (c++ language)arrow_forwardWrite the definitions of the member functions of the classes arrayListType and unorderedArrayListType that are not given in this chapter. The specific methods that need to be implemented are listed below. Implement the following methods in arrayListType.h: isEmpty isFull listSize maxListSize clearList Copy constructor Implement the following method in unorderedArrayListType.h insertAt Also, write a program (in main.cpp) to test your function.arrow_forwardDescription is " A MathVector object will be passed to your method. Return its contents as a String. If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector object as a String. This makes it useful for displaying to the user. You might have noticed that there's an @override term there. That's because many objects already have a "toString()" method associated with them... because Java was designed to include them by default. Here, the override tells Java "I know, I know. You already have a toString() that you'd assign here. But it's not good enough. Here's a better one for this particular kind of object." It's a little bit like saying "Most humans have two legs. So, by default, I'll give everyone two legs. But sometimes we override that and give no legs, or just one leg to a person. And sometimes we give them four so that they can be a centaur!" To use this in a println() method, just name your object. The toString()…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
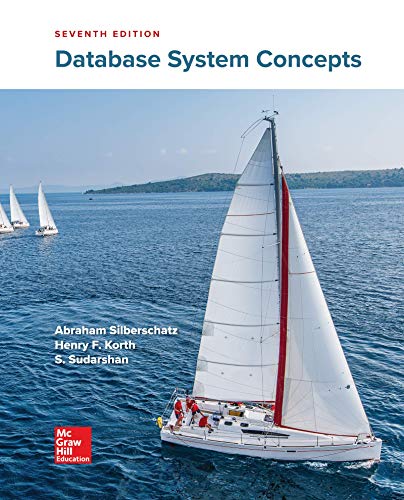
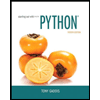
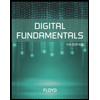
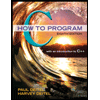
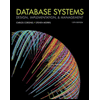
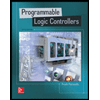