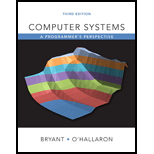
Compile and run the sample code that uses show_bytes (file show-bytes. c) on different machines to which you have access. Determine the byte orderings used by these machines.

Byte ordering:
- Some machines decide to store the objects in memory ordered from least significant byte to most, while other machines store them from most to least.
- The byte ordering are made by the two ways:
- Little Endian
- In little Endian, the least significant byte comes first.
- Big Endian
- In big Endian, the most significant byte comes first.
- Little Endian
Example:
The example for find the little-endian and big-endian for hexadecimal value is shown below:
Here assume that the hexadecimal value is “0x13244860”. Then address range for given ordering byte is “0x200” through “0x203”.
Big Endian for given hexadecimal value is
0x200 | 0x201 | 0x202 | 0x203 |
13 | 24 | 48 | 60 |
Little Endian for given hexadecimal value is
0x200 | 0x201 | 0x202 | 0x203 |
60 | 48 | 24 | 13 |
Explanation of Solution
Corresponding code from given question:
#include <stdio.h>
//Define variable "byte_pointer" in char datatype.
typedef unsigned char* byte_pointer;
//Function definition for show_bytes.
void show_bytes(byte_pointer start, size_t len)
{
//Declare variable "i" in int data type.
int i;
/* "For" loop to determine byte representation in hexadecimal */
for (i = 0; i < len; i++)
//Display each bytes in "2" digits hexadecimal value.
printf(" %.2x", start[i]);
printf("\n");
}
//Function to determine byte for "int" number.
void show_int(int x)
{
//Call show_bytes function with integer value.
show_bytes((byte_pointer) &x, sizeof(int));
}
//Function to determine byte for "float" number.
void show_float(float x)
{
//Call show_bytes function float value.
show_bytes((byte_pointer) &x, sizeof(float));
}
//Function to determine byte for "pointer" number.
void show_pointer(void *x)
{
//Call show_bytes function with pointer value.
show_bytes((byte_pointer) &x, sizeof(void *));
}
//Test all show bytes.
void test_show_bytes(int val)
{
//Define variables.
int ival = val;
float fval = (float) ival;
int *pval = &ival;
//Call function.
show_int(ival);
show_float(fval);
show_pointer(pval);
}
//Main function.
int main(int argc, char* argv[])
{
//Define the sample number.
int sampleNumber = 682402;
//Call test_show_bytes function.
test_show_bytes(sampleNumber);
return 0;
}
The given program is used to display the byte representation of different program objects by using the casting.
- Define “byte_pointer” using “typedef”.
- It is used to define data type as a pointer to an object of type “unsigned char”.
- The function “show_bytes” is used to display the address of a byte sequence by using the argument that is byte pointer and a byte count.
- Each byte is displayed by “2” digit.
- The function “show_int” is to display the byte representations of object of “int” data type.
- The function “show_float” is to display the byte representations of object of “float” data type.
- The function “show_pointer” is to display the byte representations of object of “void *” data type.
- Test all the data type values by using function “test_show_bytes”.
- Finally, assign the sample number in main function and call the “test_show_bytes” with argument “sampleNumber”.
Byte ordering used by the given machines:
After compiling and running the above code, the following output will be appear
a2 69 0a 00
20 9a 26 49
3c cc e9 18 ff 7f 00 00
From the above output,
- The byte representation for “int” data type is “a2 69 0a 00”.
- The byte representation for “float” data type is “20 9a 26 49”.
- The byte representation for “int *”data type is “3c cc e9 18 ff 7f 00 00”.
The byte ordering used by these machines is “big-endian”.
- Reason:
- Consider, the byte representation of “int” value is “a2 69 0a 00”.
- From this, the value is ordered from most significant byte to least significant byte. Hence, it is referred as big-endian.
Want to see more full solutions like this?
Chapter 2 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Additional Engineering Textbook Solutions
Introduction To Programming Using Visual Basic (11th Edition)
Degarmo's Materials And Processes In Manufacturing
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
SURVEY OF OPERATING SYSTEMS
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out With Visual Basic (8th Edition)
- (1 point) By dragging statements from the left column to the right column below, give a proof by induction of the following statement: an = = 9" - 1 is a solution to the recurrence relation an = 9an-18 with ao = : 0. The correct proof will use 8 of the statements below. Statements to choose from: Note that a₁ = 9a0 + 8. Now assume that P(n) is true for all n ≥ 0. Your Proof: Put chosen statements in order in this column and press the Submit Answers button. Let P(n) be the predicate, "a = 9″ – 1". απ = 90 − 1 = Note that Let P(n) be the predicate, "an 9" - 1 is a solution to the recurrence relation an = 9an-1 +8 with ao = 0." - Now assume that P(k + 1) is true. Thus P(k) is true for all k. Thus P(k+1) is true. Then ak+1 = 9ak +8, so P(k + 1) is true. = 1 − 1 = 0, as required. Then = 9k — 1. ak Now assume that P(k) is true for an arbitrary integer k ≥ 1. By the recurrence relation, we have ak+1 = ak+1 = = 9ak + 8 = 9(9k − 1) + 8 This simplifies to 9k+19+8 = 9k+1 − 1 Then 9k+1 − 1 = 9(9*…arrow_forwardGiven the following request to an AI chatbot, which response is more accurate? (You should use Google to verify that the factual claims in each response are accurate.) Prompt Is this true? The first recorded use of the phrase "when pigs fly" in print is in a 1616 play by Ben Jonson called "The Devil is an Ass," in which a character says, "When pigs fly, good-night!!" Response Response A Response B Yes, that's true! The phrase "when pigs fly" indeed appears in Ben Jonson's 1616 play The Devil is an Ass and was said during the second act in a conversation between Fitzdottrel and Pug. The phrase was first used to express skepticism or disbelief, suggesting that something is as unlikely to happen as the fanciful idea of pigs flying. This usage is often cited as one of the earliest instances of the phrase in print. The claim that the phrase "when pigs fly" was first recorded in Ben Jonson's 1616 play "The Devil is an Ass" is not accurate. There is no evidence from the…arrow_forwardThis is for my Computer Organization & Assembly Language Classarrow_forward
- Please answer the homework scenario below and make a JAVA OOP code. You have been hired by GMU to create and manage their course registration portal. Your first task is to develop a program that will create and track different courses in the portal. Each course has the following properties: • a course number ex. IT 106, IT 206, • A course description, ex. Intro to Programming • Total credit hour ex. 3.0, and • current enrollment ex. 30 Each course must have at least a course number and credit hours. The maximum enrollment for each course is 40 students. The current enrollment should be no greater than the maximum enrollment. A course can have a maximum of 4 credit hour. The DDC should calculate the number of seats remaining for the course. Design an object-oriented solution to create a data definition class for the course object. The course class must define all the constructors, mutators with proper validation, accessors, and special purpose methods. The DDC should calculate the…arrow_forwardFor this case study, students will analyze the ethical considerations surrounding artificial intelligence and big data in healthcare, as explored in the case study found in the textbook (pages 34-36) and in the extended version available here There will also be additional articles in this weeks learning module to show both sides of the coin. https://www.delftdesignforvalues.nl/wp-content/uploads/2018/03/Saving-the-life-of-medical-ethics-in-the-age-of-AI-and-Big-Data.pdf Students should refer to the syllabus for specific guidelines regarding length, format, and content requirements. Reflection Questions to Consider: What are the key ethical dilemmas presented in the case? How does AI challenge traditional medical ethics principles such as autonomy, beneficence, and confidentiality? In what ways can responsible innovation help address moral overload in healthcare decision-making? What are the potential risks and benefits of integrating AI-driven decision-making into patient care?…arrow_forwardCan you please solve this. Thanksarrow_forward
- can you solve this pleasearrow_forwardIn the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forwardIn the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forward
- Send me the lexer and parserarrow_forwardHere is my code please draw a transition diagram and nfa on paper public class Lexer { private static final char EOF = 0; private static final int BUFFER_SIZE = 10; private Parser yyparser; // parent parser object private java.io.Reader reader; // input stream public int lineno; // line number public int column; // column // Double buffering implementation private char[] buffer1; private char[] buffer2; private boolean usingBuffer1; private int currentPos; private int bufferLength; private boolean endReached; // Keywords private static final String[] keywords = { "int", "print", "if", "else", "while", "void" }; public Lexer(java.io.Reader reader, Parser yyparser) throws Exception { this.reader = reader; this.yyparser = yyparser; this.lineno = 1; this.column = 0; // Initialize double buffering buffer1 = new char[BUFFER_SIZE]; buffer2 = new char[BUFFER_SIZE]; usingBuffer1 = true; currentPos = 0; bufferLength = 0; endReached = false; // Initial buffer fill fillBuffer(); } private…arrow_forwardIf integer x is divisible by 3, can you prove that ceil(x/2) + floor(x/6) = floor(x/2) + ceil(x/6)arrow_forward
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- COMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
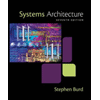
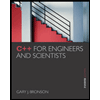
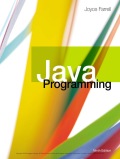
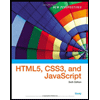