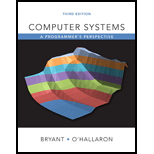
Write code to implement the following function:
/*
* Generate mask indicating leftmost 1 in x. Assume w=32.
* For example, 0xFF00 -> 0x8000, and 0x6600 — > 0x4000.
* If x = 0, then return 0.
*/
int leftmost_one(unsigned x);
Your function should follow the bit-level integer coding rules (page 128), except that you may assume that data type int has w = 32 bits.
Your code should contain a total of at most 15 arithmetic, bitwise, and logical operations.
Hint: First transform x into a bit

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Additional Engineering Textbook Solutions
SURVEY OF OPERATING SYSTEMS
Management Information Systems: Managing The Digital Firm (16th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Mechanics of Materials (10th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
- 3. Consider the following code segment: X = 0 for i in range(3): for j in range(3): х3х+ 1 X = x*2 What is the value of x after the code segment has run? (a) 90 (b) 42 (с) 18 (d) 6arrow_forwardQ11/ A function f(t) is said to be even if: Oa) f(t) = f(-t) Ob) f(t) = -f(-t) Oc) f(t) # f(-t) %3D Od) None of thesearrow_forwardLanguage :- Python Problem Statement:Given an integer N, your task is to find an NxN layout of X's and O's such that noaxis-aligned square (2x2 or larger) within the grid has the same symbol at each ofits four corners.arrow_forward
- .CLO1.1:Simplify this function F(A,B,C,D)= E (0,2,4,6), given that d(A,B,C,D)= E (8,10,12,14) 'D A O 'B .B O C'D' + CD .C O 'C.D Oarrow_forwardIn c++ Now write a program for a double floating pointtype.•What are the number of significant bits that the double’s mantissa can hold, excluding the sign bit.•What is the largest number that a double’s mantissa can hold without roundoff error?•Repeat the program shown on the previous page but set to show where the double’s mantissa starts to exhibit roundoff errors.arrow_forwardC++ only ExampleInput:215 100123456789 9876543 Output:70102768568246676arrow_forward
- in c please comment on code Write a program to read a real number, r, and solve sin(r) =r² – x² using Bracketing and Bisection. You can use the C function sin(x) from math.h. Example output: Please enter r: 4 A root is near 4.101086 where sin(4.101086) = -0.818901 and r 2-x 2 = -0.818902arrow_forwardProgramming Language :- Carrow_forwardin cpp language pleasearrow_forward
- Java Functions with 1D Array Write a Function that accepts two integers X and Y and prints the binary representation of the numbers starting from X to Y. Note: X would always be lesser than Y. Input 1. Integer X 2. Integer Y 3. Integer X 4. Integer Y Output: Enter X: 5 Enter Y: 10 101 110 111 1000 1001 1010arrow_forwardUrgent C++ Code ExampleInput:215 100123456789 9876543 Output:70102768568246676arrow_forwardQ. use set and test or swap #include <stdio.h>#include <stdlib.h>#include <pthread.h> long long sum=0,num=2000000; pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; void *mySumFun(void *vargp){int step=*(int *) vargp; for(int i=0;i<num;i++){ //pthread_mutex_lock(&mutex);sum+=step;// pthread_mutex_unlock(&mutex);}return NULL;}int main(){pthread_t tid1,tid2;int step1=1, step2=-1;pthread_create(&tid1, NULL, mySumFun, &step1); pthread_create(&tid2, NULL, mySumFun, &step2);pthread_join(tid2, NULL);pthread_join(tid1, NULL);printf("The sum is = %lld \n", sum);exit(0);}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
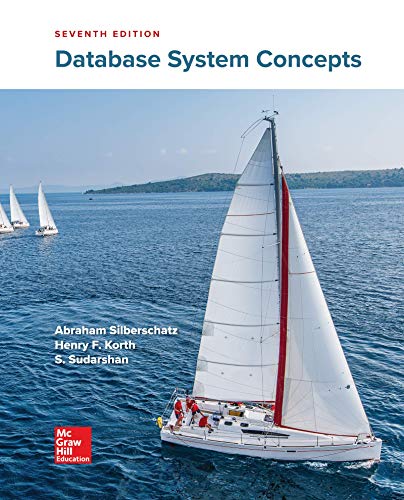
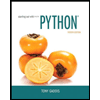
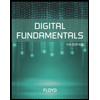
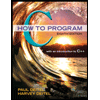
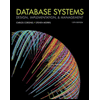
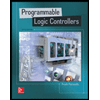