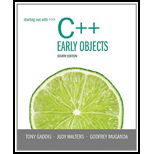
Explanation of Solution
Appending a node:
It is the process of adding a new node at the end of a linked list.
Inserting a node:
It is the process of adding a new node at a specific location in a list.
Member function for appending a node:
The following member function is used to append the received value into a list at end:
//Definition of appendNode() function
void IntList::appendNode(int num)
{
//Declare a structure pointer variables
ListNode *newNode, *dataPtr = nullptr;
//Assign a new node
newNode = new ListNode;
//Store the argument value into "newNode"
newNode->value = num;
//Assign the next pointer to be "NULL"
newNode->next = nullptr;
//Condition to check whether the list is empty or not
if (!head)
//If true, make newNode as the first node
head = newNode;
//else part
else
{
//Store head value into dataPtr
dataPtr = head;
//Loop to find the last node in the list
while (dataPtr->next)
//Assign last node to the structure variable
dataPtr = dataPtr->next;
//Insert newNode as the last node
dataPtr->next = newNode;
}
}
Explanation:
Consider the function named “appendNode()” to insert the received value “num” at end of the list.
- Make a new node and assign a received value “num” into the node.
- Using “if…else” condition check whether the list is empty or not.
- If the “head” node is empty, assign a new node into “head” pointer.
- Otherwise, make a loop to find a last node in the list and insert the value at end of the list.
Member function for inserting a node:
The following member function is used to insert the received value into a list at specific location:
//Definition of insertNode() function
void IntList::insertNode(int num)
{
/*Declare a structure pointer variables*/
ListNode *newNode, *dataPtr, *prev = nullptr;
//Assign a new node
newNode = new ListNode;
//Store the argument value into "newNode"
newNode->value ...

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Starting Out with C++: Early Objects
- Index Numbers: Why do we use index numbers?arrow_forwardPYTHON CODE Reza Enterprises sells tickets for buses, tours, and other travel services. Because Reza frequently mistypes long ticket numbers, Reza Enterprises has asked his students to write an application that shows if a ticket is invalid. Your application/program tells the ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you lose the last digit of the number, then divide by 7, the remainder of the division is exactly the same to the last dropped digit. This process is shown below: Step 1: Enter the ticket number; for example 123454 Step 2: Remove the last digit, leaving 12345 Step 3: Determine the remainder when the ticket number from step 2 is divided by 7. In this case, 12345 divided by 7 leaves a remainder of 4. Step 4: Display a message to the ticket agent indicating whether the ticket number is valid or not. If the ticket number is valid, save the number to a .txt file called “tickets.txt” and continue to loop your program asking…arrow_forwardWhat is displayed as a result of executing the code segment?arrow_forward
- What is one possible consequence of leaving the mouse pointer on an irregular section of code for an extended period of time?arrow_forwardEmm is it possible to slightly change the code a bit, so it gets multiple data from multiple variables in a struct, Much appreciatedarrow_forwardSteps for the code Let's say you have a 5-digit number, 23456. You are supposed to write a code that prints numbers in the ones, tens, hundreds, thousands, and ten thousands places of this number. In the text box below, write the steps you will follow to write the code. Share it on the discussion forum. Here, you only need to write the approach in a step-by-step manner, just like you did with algorithm design in the case of computational thinking.arrow_forward
- PYTHON PROGRAMMING Reza Enterprises sells tickets for buses, tours, and other travel services. Because Reza frequently mistypes long ticket numbers, Reza Enterprises has asked his students to write an application that shows if a ticket is invalid. Your application/program tells the ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you lose the last digit of the number, then divide by 7, the remainder of the division is exactly the same to the last dropped digit. This process is shown below: Step 1: Enter the ticket number; for example 123454 Step 2: Remove the last digit, leaving 12345 Step 3: Determine the remainder when the ticket number from step 2 is divided by 7. In this case, 12345 divided by 7 leaves a remainder of 4. Step 4: Display a message to the ticket agent indicating whether the ticket number is valid or not. If the ticket number is valid, save the number to a .txt file called “tickets.txt” and continue to loop your program…arrow_forward#arrow_forwardJava - Golf Scoresarrow_forward
- 99 question Reza Enterprises sells tickets for buses, tours, and other travel services. Because Reza frequently mistypes long ticket numbers, Reza Enterprises has asked his students to write an application that shows if a ticket is invalid. Your application/program tells the ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you lose the last digit of the number, then divide by 7, the remainder of the division is exactly the same to the last dropped digit. This process is shown below: Step 1: Enter the ticket number; for example 123454 Step 2: Remove the last digit, leaving 12345 Step 3: Determine the remainder when the ticket number from step 2 is divided by 7. In this case, 12345 divided by 7 leaves a remainder of 4. Step 4: Display a message to the ticket agent indicating whether the ticket number is valid or not. If the ticket number is valid, save the number to a .txt file called “tickets.txt” and.arrow_forwardpython multiple choice Code Example 4-1 def get_username(first, last): s = first + "." + last return s.lower() def main(): first_name = input("Enter your first name: ") last_name = input("Enter your last name: ") username = get_username(first_name, last_name) print("Your username is: " + username) main() 5. Refer to Code Example 4-1: What is the scope of the variable named s ?a. globalb. localc. global in main() but local in get_username()d. local in main() but global in get_username()arrow_forwardUsing only the programming techniques you learned in this chapter, write an application that calculates the squares and cubes of the numbers from 0 to 10 and prints the resulting values in table format, as shown below. number square cube 0 0 0 1 1 1 2 4 8 3 9 27 4 16 64 5 25 125 6 36 216 7 49 343 8 64 512 9 81 729 10 100 1000arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
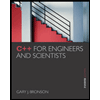
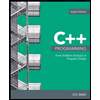
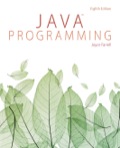
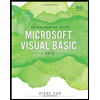