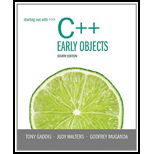
Starting Out with C++: Early Objects
8th Edition
ISBN: 9780133360929
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 12PC
Program Plan Intro
Recursive Generation of Subsets
Program Plan:
- Include the required header files
- Declare function prototypes.
- Define the “main ()” function.
- Declare the required variables.
- Get the input from the user.
- If the user input is not in the range the condition exits the program.
- Create the list and call the function “get_Subsets ()”.
- Display the output.
- Define the overloaded stream insertion operator for vector of int.
- Display the open square bracket.
- If the number is in the range, display the number inside the bracket.
- Display the close square bracket.
- Return the output to the main function.
- Define the overloaded stream insertion operator for a list of generic type.
- Display the open square bracket.
- Create a list and declare the variable name “itr” for the list.
- While condition used to check if the “itr” is not equal to last number in the same list.
- If so, display the number.
- Increment the “itr”.
- Print the numbers separated by commas.
- Display the close square bracket.
- Return the output to the main function.
- Define the “get_subsets” vector function.
- Declare the list of subsets.
- Check the “n” value is equal to 0.
- If do, start with a list of subsets of 1 to n that contains on the empty set.
- Return the sub list.
- Get the list of all subsets of 1 to n-1 and store it in a “sub_setList”.
- Temporarily used to extend the subset list by declaring the vector variables.
- While condition used to check if the “itr” is not equal to last number in the same list.
- Declare the vector variables.
- Push the value to the list.
- Increment the “itr” value.
- Return the value to the main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
3. Largest: a recursive function that computes the largest value for an integer array of positiveand negative values. For example, for the array below, the function largest should return 22,which is the largest value in the array. You can assume there are no more 20 integers in thearray. Think of how to formulate the recurrence relation in this problem yourself.
CodeW
For fun X C Solved
https://codeworkou...
臺亂
CodeWorkout
X272: Recursion Programming Exercise: Is
Reverse
For function isReverse, write the two missing base case conditions. Given two strings, this
function returns true if the two strings are identical, but are in reverse order. Otherwise it
returns false. For example, if the inputs are "tac" and "cat", then the function should return true.
Examples:
isReverse("tac", "cat") -> true
Your Answer:
1 public boolean isReverse(String s1, String s2) {
2.
if >
3.
4.
else if >
return true;
return false;
5.
6.
else {
String s1first =
String s2last
return s1first.equals (s2last) &&
51. substring(0, 1);
s2, substring(s2.length() 1);
7.
8.
6.
isReverse(s1.substring(1), s2.substring(0, s2.length() 1));
{
12}
1:11AM
50°F Clear
12/4/2021
Write a recursive function that finds the minimum value in an ArrayList.
Your function signature should be
public static int findMinimum(ArrayList<Integer>)
One way to think of finding a minimum recursively is to think “the minimum number is either the last element in the ArrayList, or the minimum value in the rest of the ArrayList”.
For example, if you have the ArrayList
[1, 3, 2, 567, 23, 45, 9],
the minimum value in this ArrayList is either 9 or the minimum value in [1, 3, 2, 567, 23, 45]
Hint:The trick is to remove the last element each time to make the ArrayList a little shorter.
import java.util.*;
public class RecursiveMin{public static void main(String[] args){Scanner input = new Scanner(System.in);ArrayList<Integer> numbers = new ArrayList<Integer>();while (true){System.out.println("Please enter numbers. Enter -1 to quit: ");int number = input.nextInt();if (number == -1){break;}else {numbers.add(number);}}
int minimum =…
Chapter 17 Solutions
Starting Out with C++: Early Objects
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Why does the insertNode function shown in this...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Write a function void printSecond(ListNode ptr}...Ch. 17 - Write a function double lastValue(ListNode ptr)...Ch. 17 - Write a function ListNode removeFirst(ListNode...Ch. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Running Back Program 17-11 makes a person run from...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a recursive function that finds the minimum value in an ArrayList. Your function signature should be public static int findMinimum(ArrayList<Integer>) One way to think of finding a minimum recursively is to think “the minimum number is either the last element in the ArrayList, or the minimum value in the rest of the ArrayList”. For example, if you have the ArrayList [1, 3, 2, 567, 23, 45, 9], the minimum value in this ArrayList is either 9 or the minimum value in [1, 3, 2, 567, 23, 45] ================================================ import java.util.*; public class RecursiveMin{public static void main(String[] args){Scanner input = new Scanner(System.in);ArrayList<Integer> numbers = new ArrayList<Integer>();while (true){System.out.println("Please enter numbers. Enter -1 to quit: ");int number = input.nextInt();if (number == -1){break;}else {numbers.add(number);}} int minimum = findMinimum(numbers);System.out.println("Minimum: " + minimum);}public static int…arrow_forwardExercise 1: The number of combinations CR represents the number of subsets of cardi- nal p of a set of cardinal n. It is defined by C = 1 if p = 0 or if p = n, and by C = C+ C in the general case. An interesting property to nxC calculate the combinations is: C : Write the recursive function to solve this problem.arrow_forwardThe Polish mathematician Wacław Sierpiński described the pattern in 1915, but it has appeared in Italian art since the 13th century. Though the Sierpinski triangle looks complex, it can be generated with a short recursive function. Your main task is to write a recursive function sierpinski() that plots a Sierpinski triangle of order n to standard drawing. Think recursively: sierpinski() should draw one filled equilateral triangle (pointed downwards) and then call itself recursively three times (with an appropriate stopping condition). It should draw 1 filled triangle for n = 1; 4 filled triangles for n = 2; and 13 filled triangles for n = 3; and so forth. API specification. When writing your program, exercise modular design by organizing it into four functions, as specified in the following API: public class Sierpinski { // Height of an equilateral triangle whose sides are of the specified length. public static double height(double length) // Draws a filled equilateral…arrow_forward
- Your main task is to write a recursive function sierpinski() that plots a Sierpinski triangle of order n to standard drawing. Think recursively: sierpinski() should draw one filled equilateral triangle (pointed downwards) and then call itself recursively three times (with an appropriate stopping condition). It should draw 1 filled triangle for n = 1; 4 filled triangles for n = 2; and 13 filled triangles for n = 3; and so forth. Sierpinski.java When writing your program, exercise modular design by organizing it into four functions, as specified in the following API: public class Sierpinski { // Height of an equilateral triangle with the specified side length. public static double height(double length) // Draws a filled equilateral triangle with the specified side length // whose bottom vertex is (x, y). public static void filledTriangle(double x, double y, double length) // Draws a Sierpinski triangle of order n, such that the largest filled //…arrow_forwardThe 4th problem mimics the situation where eagles flying in the sky can be spotted and counted.FindEagles: a recursive function that examines and counts the number of objects (eagles) in aphotograph. The data is in a two-dimensional grid of cells, each of which may be empty (value 0) orfilled (value 1 to 9). Maximum grid size is 50 x 50. The filled cells that are connected form an object(eagle). Two cells are connected if they are vertically, horizontally, or diagonally adjacent. Thefollowing figure shows 3 x 4 grids with 3 eagles. 0 0 1 21 0 0 01 0 3 1 FindEagle function takes as parameters the 2-D array and the x-y coordinates of a cell that is a part ofan eagle (non-zero value) and erases (change to 0) the image of an eagle. The function FindEagleshould return an integer value that counts how many cells has been counted as part of an eagle and havebeen erased. The following sample data has two pictures, the first one is 3 x 4, and the second one is 5 x 5 grids. Notethat your program…arrow_forwardPython Lee has discovered what he thinks is a clever recursive strategy for printing the elements in a sequence (string, tuple, or list). He reasons that he can get at the first element in a sequence using the 0 index, and he can obtain a sequence of the rest of the elements by slicing from index 1. This strategy is realized in a function that expects just the sequence as an argument. If the sequence is not empty, the first element in the sequence is printed and then a recursive call is executed. On each recursive call, the sequence argument is sliced using the range 1:. Here is Lee’s function definition: def printAll(seq): if seq: print(seq[0]) printAll(seq[1:]) Write a program that tests this function and add code to trace the argument on each call. Does this function work as expected? If so, are there any hidden costs in running it? its supposed to print something like thisarrow_forward
- For function addOdd(n) write the missing recursive call. This function should return the sum of all postive odd numbers less than or equal to n. Examples: addOdd(1) -> 1addOdd(2) -> 1addOdd(3) -> 4addOdd(7) -> 16 public int addOdd(int n) { if (n <= 0) { return 0; } if (n % 2 != 0) { // Odd value return <<Missing a Recursive call>> } else { // Even value return addOdd(n - 1); }}arrow_forwardGive a recursive definition for the set of all strings of a’s and b’s that begins with an a and ends in a b. Say, S = { ab, aab, abb, aaab, aabb, abbb, abab..} Let S be the set of all strings of a’s and b’s that begins with a and ends in a b. The recursive definition is as follows – Base:... Recursion: If u ∈ S, then... Restriction: There are no elements of S other than those obtained from the base and recursion of S.arrow_forward•rewrite calculateSum function as a recursive function. m(i) = m(i-1) + i/(i+1), where i >=1arrow_forward
- q17 use javaarrow_forwardImplement a recursive function called evens that returns an integer with only the even numbers. Note this function is returning an integer, not printing. There should be no use of cout within your function. The function declaration should look as follows: int evens(int n); (ex. evens(234567); returns 246) (ex. cout << evens(56032); prints 602)arrow_forwardCS211 Non-recursive solution for Towers of Hanoi Using the algorithm discussed in class, write an iterative program to solve the Towers of Hanoi problem. The problem: You are given three towers a, b, and c. We start with n rings on tower a and we need to transfer them to tower b subject to the following restrictions: 1. We can only move one ring at a time, and 2. We may never put a larger numbered ring on top of a smaller numbered one. There are always 3 towers. Your program will prompt the user for the number of rings. Here is the algorithm. Definition: A ring is "available" if it is on the top of one of the towers. Definition: The "candidate" is the smallest available ring that has not been moved on the most recent move. The first candidate is ring 1. The Algorithm: 1. Find the candidate. 2. Move the candidate (right or left, depending if the number of rings is odd or even) to the closest tower on which it can be placed. Move "around the circle" if necessary. 3. If not done, go back…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
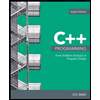
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning