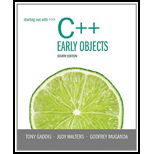
Concept explainers
A)
Explanation of Solution
Purpose of the given code:
The given code is trying to print the members of a linked list by traversing through the entire list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::printList()//Line 1
{//Line 2
//loop
//Error line3
while(head)//Line 3
{//Line 4
/*Print the data value of the node while traversing through the list*/
cout<<head->value; //Line5
/*the pointer is moved one position ahead till end of list */
head= head->next;//Line6
}//Line 7
}//Line 8
Error in the given code:
- In “line 3”, use of the head pointer to walk down the list destroys the list.
- This should be written as an “auxiliary pointer”. So, correct code is given below:
ListNode *nodePtr = head
- This should be written as an “auxiliary pointer”. So, correct code is given below:
while (nodePtr != null)
;&#x...
B)
Explanation of Solution
Purpose of the given code:
The given code is trying to print the members of a linked list by traversing through the entire list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::PrintList()//Line 1
{//Line 2
//Declaration of structure pointer variables
//Line3
ListNode *p =head; /*the start or head of the list is stored in p */
//loop
//Error Line4
while (p->next) //Line4
{//Line 5
/*Print the data value of node p while traversing through the linklist */
//Line6
cout<<p->value; /*print the individual data values of each node */
//Line7
p=p->next; //the pointer is moved one position ahead till end of list
}//Line8
}//Line9
Error in the given code:
- In “line 4”, eventually the pointer p becomes “NULL”, at which time the attempt to access p->NULL will result in an error.
- This should be written by replacing the text p->next in the while loop with p.
while (p) //Line4
/*Here the loop will traverse till p exists or till p is not NULL */
- The function fails to declare a return type of void...
C)
Explanation of Solution
Purpose of the given code:
The given code is trying to print the members of a linked list by traversing through the entire list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::PrintList()//Line 1
{//Line 2
//Declaration of structure pointer variables
//Line3
ListNode *p =head; /*the start or head of the list is stored in p */
//loop
// Line4
while (p) //Line4
{//Line 5
/*Print the data value of node p while traversing through the linklist */
//Line6
cout<<p->value; /*print the individual data values of each node */
//Error Line7
//Line7
p++//the pointer is incremented by one position
}//Line8
}//Line9
Error in the given code:
- In “line 7”, the function uses p++ erroneously in place of p=p->next when attempting to move to the next node in the list. This is not possible as increment operator can work only on variables containing data values but here p is a node of a link list which contains an address value pointer pointing to the next list along with a data value.
- This should be written by replacing the text p++ in the while loop body with p=p->next...
D)
Explanation of Solution
Purpose of the given code:
The given code is trying to destroy the members of a linked list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::~NumberList()//Line 1
{//Line 2
//Declaration of structure pointer variables
ListNode *nodePtr, *nextNode;//Line 3
//Storing "head" pointer into "nodePtr"
nodePtr = head;//Line 4
//loop
while (nodePtr != nullptr)//Line 5
{//Line 6
//Assign address of next into "nextNode"
nextNode = nodePtr->next;//Line 7
//Error
nodePtr->next=nullptr;//Line 8
//Assign nextNode into "nodePtr"
nodePtr = nextNode;//Line 9
}//Line 10
}//Line 11
Error in the given code:
In “line 8”, the address of “next” in “nodePtr” is assigned as “nullptr”.
- This should be written as “delete nodePtr” to delete the value of node from the list, because, “delete” operator is used to free the memory space allocated by the list...

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Starting Out with C++: Early Objects
- C++ ProgrammingActivity: Queue Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. #include "queue.h" #include "linkedlist.h" class SLLQueue : public Queue { LinkedList* list; public: SLLQueue() { list = new LinkedList(); } void enqueue(int e) { list->addTail(e); return; } int dequeue() { int elem; elem = list->removeHead(); return elem; } int first() { int elem; elem = list->get(1); return elem;; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } int collect(int max) { int sum = 0; while(first() != 0) { if(sum + first() <= max) { sum += first(); dequeue(); } else {…arrow_forwarddata structures-java language quickly plsarrow_forwardC++ ProgrammingActivity: Queue Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. SEE ATTACHED PHOTO FOR THE PROBLEM #include "queue.h" #include "linkedlist.h" class SLLQueue : public Queue { LinkedList* list; public: SLLQueue() { list = new LinkedList(); } void enqueue(int e) { list->addTail(e); return; } int dequeue() { int elem; elem = list->removeHead(); return elem; } int first() { int elem; elem = list->get(1); return elem;; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } int collect(int max) { int sum = 0; while(first() != 0) { if(sum + first() <= max) { sum += first();…arrow_forward
- data structures in javaarrow_forwardProblem Description: Q1) Write a method public static void downsize (LinkedList employeeNames, int n) that removes every nth employee from a linked list. Q2) Write a method public static void reverse (LinkedList strings) that reverses the entries in a linked list.arrow_forwardMultiple choice in data structures void doo(node<int>*root){ if(root !=0) { node<int>*p=root; while(root->next!=0) root=root->next; p->data=root->data; } What is this code do? a. swap the first item with the last item in the linked list b. set the first item in the linked list as the last item c. doesn't do anything because the root parameter is passed by value d. change the root item in the binary tree with the farthest leaf itemarrow_forward
- In C++ Write the DLList member function DLLNode<T>* DLLidt<T>::get_at(int index) function that return the node at specific index in the list.arrow_forwardIn C++ Write the DLList member function void DLList <T>:: RemoveAllDuplicatesOf (T& x) function, which delete all the occurrences of x (but one) in a sorted DLL. Example: If the current list is [5, 7, 7, 7, 10, 15, 25, 25]then calling:RemoveAllDuplicatesOf(7); will change the current list to [5, 7, 10, 15, 25, 25}arrow_forwardIn C++ Write the DLList member function bool DLLidt<T>::insert_before(int index, T& x) that is going to insert a node with the value of x before the node at position index in the list. Example: If the current list is [5, 6, 7, 8] insert_before(2,0); will change the current list to [5,6,0,7,8] **You need to use get_at function, and use it only one time to optimize the call of your code. ** Don’t forget to fix the next, and prev pointers for each node. ** for inserting before 0 index, you may use add_to_head function.arrow_forward
- C++ ProgrammingActivity: Deque Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. #include "deque.h" #include "linkedlist.h" #include <iostream> using namespace std; class DLLDeque : public Deque { DoublyLinkedList* list; public: DLLDeque() { list = new DoublyLinkedList(); } void addFirst(int e) { list->addAt(e,1); } void addLast(int e) { list->addAt(e,size()+1); } int removeFirst() { return list->removeAt(1); } int removeLast() { return list->removeAt(size()); } int size(){ return list->size(); } bool isEmpty() { return list->isEmpty(); } // OPTIONAL: a helper method to help you debug void print() {…arrow_forward6. Suppose that we have defined a singly linked list class that contains a list of unique integers in ascending order. Create a method that merges the integers into a new list. Note the additional requirements listed below. Notes: ● . Neither this list nor other list should change. The input lists will contain id's in sorted order. However, they may contain duplicate values. For example, other list might contain id's . You should not create duplicate id's in the list. Important: this list may contain duplicate id's, and other list may also contain duplicate id's. You must ensure that the resulting list does not contain duplicates, even if the input lists do contain duplicates.arrow_forwardReference-based Linked Lists: Select all of the following statements that are true. As a singly linked list's node references both its predecessor and its successor, it is easily possible to traverse such a list in both directions. According to the terminology introduced in class, the head reference variable in a singly linked list object references the list's first node. According to the terminology introduced in class, in a doubly linked list, each node references both the head and tail node. In a double-ended singly linked list, the tail reference variable provides access to the entire list. In a circular linked list, the last node references the first node.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
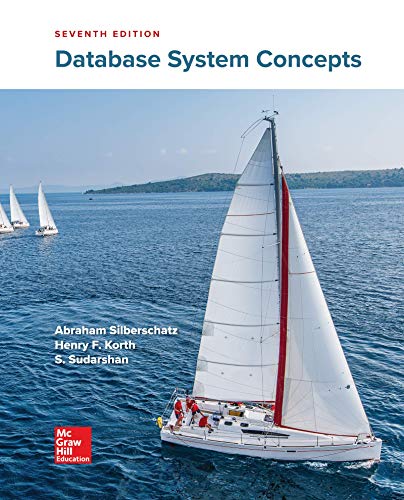
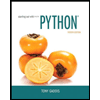
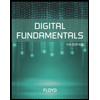
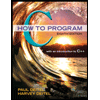
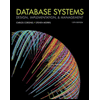
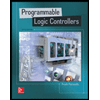