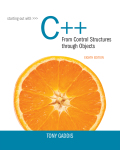
EBK STARTING OUT WITH C++
8th Edition
ISBN: 8220100794438
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16.3, Problem 16.8CP
Program Plan Intro
Function template:
In C++, a function template is referred as a “generic” function, which can work with different data types.
- While writing a function template, a programmer can specify the “type parameter” instead of using the actual data type.
- The compiler generates the code, when it encounters a function call to a function template. This code will handle the particular data type which is used in the function call.
Example:
For example consider the below function template used to find the cube of given value for any types:
//template function
template <class T>
//function definition of "cube"
T cube(T x)
{
//return the cube of the value
return x*x*x ;
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 16 Solutions
EBK STARTING OUT WITH C++
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.3 - Prob. 16.6CPCh. 16.3 - The following function accepts an i nt argument...Ch. 16.3 - Prob. 16.8CPCh. 16.3 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - Prob. 16.11CPCh. 16 - Prob. 1RQECh. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - What is unwinding the stack?Ch. 16 - What happens if an exception is thrown by a classs...Ch. 16 - How do you prevent a program from halting when the...Ch. 16 - Why is it more convenient to write a function...Ch. 16 - Why must you be careful when writing a function...Ch. 16 - Prob. 10RQECh. 16 - Prob. 11RQECh. 16 - Prob. 12RQECh. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 14RQECh. 16 - Prob. 15RQECh. 16 - Prob. 16RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 18RQECh. 16 - Prob. 19RQECh. 16 - Prob. 20RQECh. 16 - Prob. 21RQECh. 16 - _____________ are pointer-like objects used to...Ch. 16 - Prob. 23RQECh. 16 - Write a function that searches a numeric array for...Ch. 16 - Write a function that dynamically allocates a...Ch. 16 - Make the function you wrote in Question 17 a...Ch. 16 - Write a template for a function that displays the...Ch. 16 - Prob. 28RQECh. 16 - Prob. 29RQECh. 16 - Prob. 30RQECh. 16 - Prob. 31RQECh. 16 - Prob. 32RQECh. 16 - Prob. 33RQECh. 16 - Prob. 34RQECh. 16 - T F All type parameters defined in a function...Ch. 16 - Prob. 36RQECh. 16 - T F A class object passed to a function template...Ch. 16 - Prob. 38RQECh. 16 - Prob. 39RQECh. 16 - Prob. 40RQECh. 16 - Prob. 41RQECh. 16 - T F A class template may not be derived from...Ch. 16 - T F A class template may not be used as a base...Ch. 16 - Prob. 44RQECh. 16 - Prob. 45RQECh. 16 - Prob. 46RQECh. 16 - Prob. 47RQECh. 16 - try { quotient = divide(num1, num2); } cout The...Ch. 16 - template class T T square(T number) { return T T;...Ch. 16 - template class T int square(int number) { return...Ch. 16 - Prob. 51RQECh. 16 - Assume the following definition appears in a...Ch. 16 - Assume the following statement appears in a...Ch. 16 - Prob. 1PCCh. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Prob. 4PCCh. 16 - Prob. 5PCCh. 16 - IntArray Class Exception Chapter 14 presented an...Ch. 16 - TestScores Class Write a class named TestScores....Ch. 16 - Prob. 9PCCh. 16 - SortableVector Class Template Write a class...Ch. 16 - Inheritance Modification Assuming you have...Ch. 16 - Prob. 12PCCh. 16 - Prob. 13PCCh. 16 - 14. Test Scores vector
Modify Programming...Ch. 16 - Prob. 15PCCh. 16 - Prob. 16PCCh. 16 - Prob. 17PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
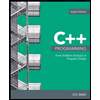
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
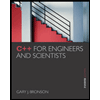
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
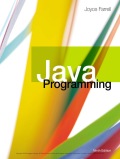
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
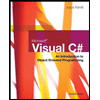
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
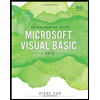
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning