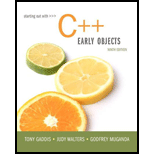
SimpleVector Modification
Modify the SimpleVector class template, presented in this chapter, to include the member functions push_back and pop_back. These functions should emulate the STL

Want to see the full answer?
Check out a sample textbook solution
Chapter 16 Solutions
Starting Out with C++: Early Objects (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Software Engineering (10th Edition)
C++ How to Program (10th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Computer Science: An Overview (12th Edition)
- C++ Program You may use the vector and iostream libraries. You are allowed to use three built-in vector member functions (and no others) but you may not have to use them all. The member functions you are allowed to use are size(), at(), and push_back() Write a function that outputs a vector of doubles with each number in the vector separated by a space then a newline after the entire vector is output. The whole vector output should be preceded by a single line saying "Current Vector Contents:". Write a function that takes a vector a doubles and reverses the order of all the elements of the vector. Write a function that fills a vector of doubles with positive numbers using the standard input stream cin, terminate the input when the user enters any negative number. A single output prompt should precede the initial input stating directions for user. Write a main function that creates an empty vector, calls functions from 2 & 3 and calls your output function before and after each…arrow_forwardTopic: pointers, dynamic array and command line arguments Write a complete C++ program named “showHelp” that accepts command line arguments. It checks whether there is a command line argument of “/help” or“/?” or “-help” followed by a topic number. It will print out “yes, topic number(<number>) if there is one. Otherwise, it prints out “no, topic number(N/A) For example, if you run this program with correct arguments as follows, it willprint out yes and its associated topic number respectivelyshowHelp /? 101showHelp /debug /help 102showHelp /print /help 103 /verboseshowHelp -verbose -debug -help And if you run the program with invalid arguments, it will print no, in all casesshowHelp -helpshowHelp 101 /?showHelp 101 102 /help /verboseshowHelp /? /help -helpNote: command line arguments are simply an array of pointers to C-string. example code: #include <iostream> using namespace std; int main(int argc, char** argv){...}arrow_forwardAbsolute Value TemplateWrite a function template that accepts an argument and returns its absolute value. The absolute value of a number is its value with no sign. For example, the absolute value of -5 is 5, and the absolute value of 2 is 2. Test the template in a simple driver program being sure to send the template short, int, double, float, and long data values.arrow_forward
- PROGRAMMING LANGUAGE: C++ Write a program which has a class named FileHandlerto read StudentName, MarksObtained from the attached picture. The class should have a function which calculates grade for a student and then adds it in a new column i.e. Grade. The grade should be calculated considering the following criteria. A: 87-100, B+: 80-86, B: 72-79, C+: 66-71, C: 60-65, D: 50-59, F: 0-49 The file should be saved once grade for all students is calculated. Write the driver program for executing the task.arrow_forwardin c++ Write a function named createTwoNames that will do the following: - Read in a word and its definition - Create the Word object for that word and definition - Read in an item’s name and its price - Create the Item object for that item - It will return an array of two pointers pointing to these newly created Word and Item objects. It will use try-catch statement to handle the exception. If the user enters an empty or all-blank word or definition or item’s name, it will not create the object(s) and return null for that object only. In another words, it may create 0, 1 or 2 objects. Whenever the error occurs, it must print out the correct error reason: empty or blank (but not both). Note that this function can and will use cin and cout to read in values from the user.arrow_forwardC++ codearrow_forward
- C++ Format Please Write a function (named "Lookup") that takes two const references to vectors. The first vector is a vector of strings. This vector is a list of words. The second parameter is a list of ints. Where each int denotes an index in the first vector. The function should return a string formed by concatenated the words (separated by a space) of the first vector in the order denoted by the second vector. Input of Test Case provided in PDF:arrow_forwardPHP Write a modeMaker function Write a function modeMaker() that forms a closure such that the function it returns can be used with your reduce() function above to find the mode of an array. The mode of an array is the value that appears the most frequently (so in the $arr above, the mode is 5). To do this, you will need an array called $seen that keeps the count of times an element has been examined At the end of the reduce function, the $seen array should look like the following for the $arr above: Array ( [10] => 1 [5] => 3 [3] => 1 [1] => 1 [2] => 1 [7] => 1 ) Note that the closure you generate should always be returning the current mode for what it has seen so far (so it will either be the current mode, or the new element passed in). The following is a start for modeMaker: function modeMaker() { $seen = array(); return function($current, $new) use (&$seen) { // your code that uses $seen goes here }; } $mode =…arrow_forwardstruct remove_from_front_of_vector { // Function takes no parameters, removes the book at the front of a vector, // and returns nothing. void operator()(const Book& unused) { (/// TO-DO (12) ||||| // // // Write the lines of code to remove the book at the front of "my_vector". // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. //// END-TO-DO (12) /||, } std::vector& my_vector; };arrow_forward
- Write a remove member function that accepts an argument for a number of units and removes that number of units of an item from inventory. If the operation is completed successfully it should return the number of units remaining in stock for that item. However, if the number of units passed to the function is less than the number of units in stock, it should not make the removal and should return –1 as an error signal.arrow_forwardC++ language Alter the code found in main.cpp Using the file, add/change the code in the file, but only where indicating you can add or change code. main.cpp #include <iostream> using namespace std; /*The function binarySearch accepts a sorted array data with no duplicates, and the range within that array to search, defined by first and last. Finally, goal is the value that is searched for within the array. If the goal can be found within the array, the function returns the index position of the goal value in the array. If the goal value does not exist in the array, the function returns -1.*/int binarySearch(int data[], int first, int last, int goal){ cout << "first: " << first << ", last: " << last << endl; // YOU CAN ONLY ADD OR CHANGE CODE BELOW THIS COMMENT return -1; // YOU CAN ONLY ADD OR CHANGE CODE ABOVE THIS COMMENT} int main(){ const int ARRAY_SIZE = 20; int searchValue; /* generates an array data that contains: 0, 10, 20,…arrow_forwardClass and Data members: Create a class called Temperature that stores temperature readings (integers) in a vector (do not use an array). The class should have data members that store the month name and year of when the temperature readings were collected. Constructor(s): The class should have a 2-argument constructor that receives the month name and year as parameters and sets the appropriate data members to these values. Member Functions: The class should have functions as follows: Member functions to set and get the month and year variables. A member function that adds a single temperature to the vector. Both negative and positive temperatures are allowed. Call this function AddTemperature. A member function to sort the vector in ascending order. Feel free to use the sort function that is available in the algorithm library for sorting vectors. Or, if you would prefer to write your own sort code, you may find this site to be helpful:…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
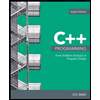