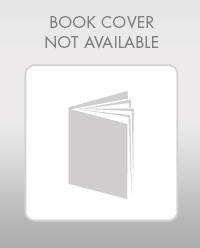
A class that cannot be instantiated is a(n) _____ .

The classes which cannot be instantiated are referred as “abstract base class”.
Explanation of Solution
Inheritance:
Inheritance is the concept of inheriting the members and properties of a base class from the derived class. The main advantages of inheritance are,
- Code reusability and fast implementation.
- Reduces the program code.
Abstract base class:
Abstract base class is a class which contains at least one pure virtual function.
- Compiler will not permit the user to instantiate an abstract class.
- Normally, in function the base class contains the implementation and the derived class overrides this implementation with its own implementation.
- But, the class which inherits an abstract base class must provide definition to the pure virtual function.
- The pure virtual functions implementation is not provided, and the function can be made pure virtual by adding (= 0) at the end of the function.
- The pure virtual function is mostly implemented in the derived class and not in a base class.
Example for abstract base class with virtual function:
/*Abstract base class as it uses virtual function*/
class Base
{
/*Access specifier*/
public:
/*virtual function*/
virtual void disp() = 0
};
/*Virtual function definition*/
void Base::disp()
{
//statement;
}
Explanation:
In the above example,
- Define the abstract base class with the name of “Base”.
- Declare the pure virtual function with the name of “disp()” to make the class as the abstract class.
- The implementation of pure virtual function “disp()” is not provided in base class. But, it is given outside of the class definition.
- Hence, the abstract base class “Base” cannot be instantiated.
Want to see more full solutions like this?
Chapter 15 Solutions
Starting Out With C++: Early Objects (10th Edition)
Additional Engineering Textbook Solutions
Degarmo's Materials And Processes In Manufacturing
Web Development and Design Foundations with HTML5 (8th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (8th Edition)
SURVEY OF OPERATING SYSTEMS
Management Information Systems: Managing The Digital Firm (16th Edition)
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
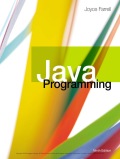
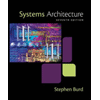
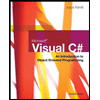
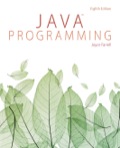
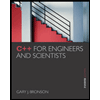