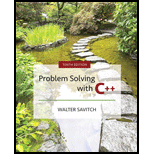
Concept explainers
Write a
A member variable of type string that contains the administrator’s title (such as Director or Vice President).
A member variable of type string that contains the company area of responsibility (such as Production, Accounting, or Personnel).
A member variable of type string that contains the name of this administrator’s immediate supervisor.
A protected: member variable of type double that holds the administrator’s annual salary. It is possible for you to use the existing salary member if you did the change recommended earlier.
A member function called setSupervisor, which changes the supervisor name.
A member function for reading in an administrator’s data from the keyboard.
A member function called print, which outputs the object’s data to the screen.
An overloading of the member function printCheck() with appropriate notations on the check.

Salaried Employee
Program Plan:
administrator.h:
- Include required header files.
- Create a namespace.
- Declare a class “Administrator”.
- Inside the “protected” access specifier,
- Declare a variable to hold the salary amount.
- Inside the “public” access specifier,
- Declare the constructors.
- Declare the member functions.
- Inside the “private” access specifier,
- Declare the variables to store the title, responsibility, and name of the supervisor.
- Inside the “protected” access specifier,
- Declare a class “Administrator”.
administrator.cpp:
- Include required header files.
- Create a namespace.
- Declare constructors.
- Set the supervisor name.
- Give function definition for “readData ()”.
- Get the title, responsibility and supervisor name from the user.
- Give function definition for “print ()”.
- Print the details like name, responsibility and supervisor name.
- Give function definition for “printCheck ()”.
- Call the function “setNetPay ()” to set the amount.
- Print the name using the function “getName ()”.
- Print the amount using the function “getNetPay ()”.
- Print the employee number using the function “getSSN ()”.
salariedemployee.h:
- Include required header files.
- Create a namespace.
- Declare a class “SalariedEmployee”.
- Inside “public” access specifier,
- Declare default and parameterized constructor.
- Declare the function “getSalary ()”, and “setSalary ()”.
- Inside “protected” access specifier,
- Declare a variable “salary”.
- Inside “public” access specifier,
- Declare a class “SalariedEmployee”.
salariedemployee.cpp:
- Include required header files.
- Create a namespace.
- Instantiate the constructors.
- Give mutator and accessor functions to set and get the salary amount respectively.
employee.h:
- Include required header files.
- Create a namespace.
- Inside the “public” access specifier.
- Declare the default and parameterized constructor.
- Declare the functions.
- Inside the “private” access specifier.
- Declare required variables like “name”, “ssn”, and “netPay”.
- Inside the “public” access specifier.
employee.cpp:
- Include required header files.
- Create a namespace.
- Instantiate the constructors.
- Give mutator and accessor functions to set and get the name, employee number, and net pay.
- Give function to print the check.
main.cpp:
- Include required header files.
- Declare the “main ()” function.
- Create an object for “Administrator” class.
- Call the function “readData ()”, “print ()”, and “printCheck ()” using the object.
The below program demonstrates the creation of “Administrator” class with the required given constraints.
Explanation of Solution
Program:
administrator.h:
//Include required header files
#ifndef ADMINISTRATOR_H
#define ADMINISTRATOR_H
#include <string>
#include "salariedemployee.h"
using namespace std;
//Create a namespace
namespace SEmployees
{
//Declare a class
class Administrator : public SalariedEmployee
{
//Access specifier
protected:
//Declare a variable
double theAnnualSalary;
//Access specifier
public:
//Constructors
Administrator();
Administrator(const string& theName, const string& theSsn, double theAnnualSalary);
//Declare the member functions
void setSupervisor(const string& newSupervisorName);
void readData();
void print();
void printCheck();
//Access specifier
private:
//Declare required variables
string adminTitle;
string areaOfResponsibility;
string supervisorName;
};
}
#endif
administrator.cpp:
//Include required header files
#include <string>
#include <iostream>
#include "administrator.h"
using namespace std;
//Create a namespace
namespace SEmployees
{
//Constructor
Administrator::Administrator() : SalariedEmployee(), adminTitle("No title yet"),areaOfResponsibility("No responsibility yet"), supervisorName("No supervisor yet"){}
//Constructor
Administrator::Administrator(const string& theName, const string& theSsn,double theAnnualSalary): SalariedEmployee(theName, theSsn, theAnnualSalary), adminTitle("No title yet"), areaOfResponsibility("No responsibility yet"), supervisorName("No supervisor yet"){}
//Function to set supervisor name
void Administrator::setSupervisor(const string& newSupervisorName)
{
//Set the name
supervisorName = newSupervisorName;
}
//Function to get the information
void Administrator::readData()
{
//Print the statement
cout << "Enter the details of the administrator " << getName() << endl;
//Ge the title
cout << " Enter the administrator's title: ";
getline(cin, adminTitle);
//Get the area of responsibility
cout << " Enter the company area of responsibility: ";
getline(cin, areaOfResponsibility);
//Get the name of supervisor
cout << " Enter the name of this administrator's immediate supervisor: ";
getline(cin, supervisorName);
}
//Function to print information
void Administrator::print()
{
//Print the statement
cout << "\nDetails of the administrator..." << endl;
//Print the name
cout << "Administrator's name: " << getName() << endl;
//Print the title
cout << "Administrator's title: " << adminTitle << endl;
//Print the responsibility
cout << "Area of responsibility: " << areaOfResponsibility << endl;
//Print the supervisor name
cout << "Immediate supervisor's name: " << supervisorName << endl;
}
//Function to print the check
void Administrator::printCheck()
{
//Print the statement
cout << "\nPay check..." << endl;
//Call the function
setNetPay(salary);
//Print the statements
cout << "\n_______________________________________________\n";
//Print the name
cout << "Pay to the order of " << getName() << endl;
//Print the amount
cout << "The sum of $" << getNetPay();
cout << "\n_______________________________________________\n";
cout << "Check Stub NOT NEGOTIABLE \n";
//Print the employee number
cout << "Employee Number: " << getSSN() << endl;
//Print the salary
cout << "Salaried Employee (Administrator). Regular Pay: $" << salary;
cout << "\n_______________________________________________\n";
}
}
salariedemployee.h:
//Include required header files
#ifndef SALARIEDEMPLOYEE_H
#define SALARIEDEMPLOYEE_H
#include <string>
#include "employee.h"
using namespace std;
//Create a namespace
namespace SEmployees
{
//Declare a class
class SalariedEmployee : public Employee
{
//Access specifier
public:
//Default constructor
SalariedEmployee( );
//Parameterized constructor
SalariedEmployee (string theName, string theSSN,double theWeeklySalary);
//Function declarations
double getSalary( ) const;
void setSalary(double newSalary);
//Access specifier
protected:
//Declare a variable
double salary;
};
}
#endif
salariedemployee.cpp:
//Include required header files
#include <iostream>
#include <string>
#include "salariedemployee.h"
using namespace std;
//Create a namespace
namespace SEmployees
{
//Constructors
SalariedEmployee::SalariedEmployee( ) : Employee( ), salary(0){}
SalariedEmployee::SalariedEmployee(string theName, string theNumber,double theWeeklySalary): Employee(theName, theNumber), salary(theWeeklySalary){}
//Accessor function to get the salary
double SalariedEmployee::getSalary( ) const
{
//Return the amount
return salary;
}
//Mutator function to set the salary
void SalariedEmployee::setSalary(double newSalary)
{
//Set the amount
salary = newSalary;
}
}
employee.h:
//Include required header files
#ifndef EMPLOYEE_H
#define EMPLOYEE_H
#include <string>
using namespace std;
//Create a namespace
namespace SEmployees
{
//Declare a class
class Employee
{
//Access specifier
public:
//Declare a default constructor
Employee( );
//Declare the parameterized constructor
Employee(string theName, string theSSN);
//Declare the functions
string getName( ) const;
string getSSN( ) const;
double getNetPay( ) const;
void setName(string newName);
void setSSN(string newSSN);
void setNetPay(double newNetPay);
void printCheck( ) const;
//Access specifier
private:
//Declare required variables
string name;
string ssn;
double netPay;
};
}
#endif
employee.cpp:
//Include required header files
#include <string>
#include <cstdlib>
#include <iostream>
#include "employee.h"
using namespace std;
//Create a namespace
namespace SEmployees
{
//Constructors
Employee::Employee( ) : name("No name yet"), ssn("No number yet"), netPay(0){}
Employee::Employee(string theName, string theNumber): name(theName), ssn(theNumber), netPay(0){}
//Accessor function to get a name
string Employee::getName( ) const
{
//Return the name
return name;
}
//Accessor function to get the number
string Employee::getSSN( ) const
{
//Return the number
return ssn;
}
//Accessor function to get the pay
double Employee::getNetPay( ) const
{
//Return the pay
return netPay;
}
//Mutator function to set the name
void Employee::setName(string newName)
{
//Set the name
name = newName;
}
//Mutator function to set the number
void Employee::setSSN(string newSSN)
{
//Set the number
ssn = newSSN;
}
//Mutator function to set the pay
void Employee::setNetPay (double newNetPay)
{
//Set the pay
netPay = newNetPay;
}
//Function to print the check
void Employee::printCheck() const
{
//Print the statements.
cout << "\nERROR: printCheck FUNCTION CALLED FOR AN \n"<< "UNDIFFERENTIATED EMPLOYEE. Aborting the program.\n"<< "Check with the author of the program about this bug.\n";
exit(1);
}
}
main.cpp:
//Include required header files
#include <iostream>
#include "administrator.h"
//Create namespace
using SEmployees::Administrator;
//Main function
int main()
{
//Add details
Administrator admin("Mr. John Smith", "963-85-2741", 10000.00);
//Call the function to read information
admin.readData();
//Call the function to print
admin.print();
//Call the function to print the check
admin.printCheck();
//Return the statement
return 0;
}
Output:
Enter the details of the administrator Mr. John Smith
Enter the administrator's title: Director
Enter the company area of responsibility: Personnel
Enter the name of this administrator's immediate supervisor: Mr. Adams
Details of the administrator...
Administrator's name: Mr. John Smith
Administrator's title: Director
Area of responsibility: Personnel
Immediate supervisor's name: Mr. Adams
Pay check...
_______________________________________________
Pay to the order of Mr. John Smith
The sum of $10000
_______________________________________________
Check Stub NOT NEGOTIABLE
Employee Number: 963-85-2741
Salaried Employee (Administrator). Regular Pay: $10000
_______________________________________________
Want to see more full solutions like this?
Chapter 15 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
SURVEY OF OPERATING SYSTEMS
Starting Out With Visual Basic (8th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Database Concepts (8th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- Python - need help creating a python program that will sum the digits of a number entered by the user. For example if the user inputs the value 243 the program will output 9 because 2 + 4 + 3 = 9. The program should ask for a single integer from the user, it should then calculate the sum of all the digits of that number and output the result.arrow_forwardI need help with this in Python (with flowchart): Im creating a reverse guessing game. Then to choose a random number from 1 to 100 and the computer program will attempt to guess it, displaying the directions calculated or not. The guess will be displayed and the user will answer if it was correct or not. If correct, the game ends, if not then the computer asks if the guess was too high or too low. Finally inputting an answer and the computer generates a new guess within the proper range. Oh and to make sure the program doesnt guess outside of the ranges produced by the inputs of “too high” and “too low”. The program ending when the user guesses correctly or after the program takes 6 guesses. HELP ASAP!arrow_forwardI need help with this in Python (with flowchart): Im creating a reverse guessing game. Then to choose a random number from 1 to 100 and the computer program will attempt to guess it, displaying the directions calculated or not. The guess will be displayed and the user will answer if it was correct or not. If correct, the game ends, if not then the computer asks if the guess was too high or too low. Finally inputting an answer and the computer generates a new guess within the proper range. Oh and to make sure the program doesnt guess outside of the ranges produced by the inputs of “too high” and “too low”. The program ending when the user guesses correctly or after the program takes 6 guesses. HELP ASAP!arrow_forward
- Need help finding errors in my pseudocode (Two)! Declare Boolean finished = False Declare Integer value, cube While NOT finished Display "Enter a value to be cubed." Input value; Set cube = value^3 Display value, " cubed is ", cube End While Next, I intended the following pseudocode to display the numbers 1 through 60, and then display the message "Time’s up!". Doesnt work and has error. Declare Integer counter = 1 Const Integer TIME_LIMIT = 60 While counter < TIME_LIMIT Display counter Set counter = counter + 1 End While Display "Time's up!"arrow_forwardHaving error in pseudcode; wanting to get five sets of two numbers each, calculate the sum of each set, and calculate the sum of all the numbers entered. Not functioning as intended and can't find the error.Code: // This program calculates the sum of five sets of two numbers. Declare Integer number, sum, total Declare Integer sets, numbers Constant Integer MAX_SETS = 5 Constant Integer MAX_NUMBERS = 2 Set sum = 0; Set total = 0; For sets = 1 To MAX_NUMBERS For numbers = 1 To MAX_SETS Display "Enter number ", numbers, " of set ", sets, "." Input number; Set sum = sum + number End For Display "The sum of set ", sets, " is ", sum "." Set total = total + sum Set sum = 0 End For Display "The total of all the sets is ", total, "."arrow_forwardNeed help converting loops!1. Convert the following While loop to a For loop: Declare Integer count = 0 While count < 50 Display "The count is ", count Set count = count + 1 End While _________________________________________________ 2. Convert the following For loop to a While loop: Declare Integer count For count = 1 To 50 Display count End Forarrow_forward
- Need help making this!1.Design a nested loop that displays 10 rows of # characters. There should be 15 # characters in each row. 2. Design a nested set of for loops that displays the following arrangements of ‘X’ characters X XX XXX XXXX XXXXX XXXXXXarrow_forwardI need help to resolve the case, thank youarrow_forwardIn 32-bit MSAM, You were given the following negative array. write a program that converts each array element to its positive representation. Then add all these array elements and assign them to the dl register. .data myarr sbyte -5, -6, -7, -4.code ; Write the rest of the program and paste the fully working code in the space below. the dl register should have the value 22 after summing up all elements in the array.arrow_forward
- Microprocessor 8085 Lab Experiment Experiment No. 3 Logical Instructions Write programs with effects 1. B=(2Dh XOR D/2) - (E AND 2Eh+1) when E=53, D=1Dh 2. HL= (BC+HL) XOR DE (use register pair when necessary), when BC=247, HL 516, DE 12Ach 3. Reset bits 1,4,6 of A and set bits 3,5 when A=03BH Write all as table (address line.hexacode,opcede,operant.comment with flags)arrow_forwardIn 32-bit MASM, Assume your grocery store sells three types of fruits. Apples, Oranges, and Mangos. Following are the sale numbers for the week (7 days).dataapples dword 42, 47, 52, 63, 74, 34, 73oranges dword 78, 53, 86, 26, 46, 51, 60mangos dword 30, 39, 41, 70, 75, 84, 29Using a single LOOP instruction, write a program to add elements in all these three arrays. Then assign the total result into the eax register. The eax register should have the value 1153 after a successful execution.arrow_forwardYou were given the following negative array. write a program that converts each array element to its positive representation. Then add all these array elements and assign them to the dl register. .data myarr sbyte -5, -6, -7, -4.code ; Write the rest of the program and paste the fully working code in the space below. The dl register should have the value 22 after summing up all elements in the array. Your answer must be in 32-bit MSAM.arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
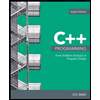
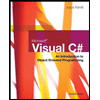
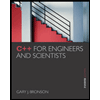
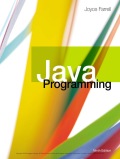