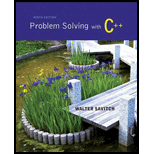
Problem Solving with C++ (9th Edition)
9th Edition
ISBN: 9780133591743
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 14.3, Problem 15STE
Explanation of Solution
Recursive function definition for “squares” function:
The recursive function definition for “squares” function is shown below:
//Function definition for "squares" function
int squares(int n)
{
/* If "n" is less than or equal to "1" */
if (n <= 1)
//Returns "1"
return 1;
//Otherwise
else
/* Recursively call the "squares" function with subtracting the value of "n" by "1" and then add and multiplied by "n" */
return (squares(n-1) + n * n);
}
Explanation:
The above function is used to compute the sum of the squares of numbers from “1” to “n”.
- In this function, first check the value of “n”. If the value of “n” is less than or equal to “1”, then returns “1”.
- Otherwise, recursively call the “squares” function with subtracting the value of “n” by “1” and then add and multiplied by “n”...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a recursive function definition for the following function: int squares(int n); //Precondition: n >= 1 //Returns the sum of the squares of numbers 1 through n. For example, squares(3) returns 14 because 12 + 22 + 32 is 14.
Write a recursive function that computes the sum of the digits in an integer. Use the following function header:
def sumDigits(n):For example, sumDigits(234) returns Write a test program that prompts the user to enter an integer and displays its sum.
Problem: Recursive Power Method
Design a python function that uses recursion to raise a number to a power. The function should accept two arguments: the number to be raised, and the exponent. Assume the exponent is a nonnegative integer.
Write the main() function to input the required parameters as shown in thesample input/output.
Sample Output:Average number of words per line: 26.0Enter a number: 2Enter a positive whole number between 1 and 100: 102.0 raised to the power of 10 is 1,024.00
Chapter 14 Solutions
Problem Solving with C++ (9th Edition)
Ch. 14.1 - Prob. 1STECh. 14.1 - Prob. 2STECh. 14.1 - Prob. 3STECh. 14.1 - Prob. 4STECh. 14.1 - Prob. 5STECh. 14.1 - If your program produces an error message that...Ch. 14.1 - Write an iterative version of the function cheers...Ch. 14.1 - Write an iterative version of the function defined...Ch. 14.1 - Prob. 9STECh. 14.1 - Trace the recursive solution you made to Self-Test...
Ch. 14.1 - Trace the recursive solution you made to Self-Test...Ch. 14.2 - What is the output of the following program?...Ch. 14.2 - Prob. 13STECh. 14.2 - Redefine the function power so that it also works...Ch. 14.3 - Prob. 15STECh. 14.3 - Write an iterative version of the one-argument...Ch. 14 - Prob. 1PCh. 14 - Prob. 2PCh. 14 - Write a recursive version of the search function...Ch. 14 - Prob. 4PCh. 14 - Prob. 5PCh. 14 - The formula for computing the number of ways of...Ch. 14 - Write a recursive function that has an argument...Ch. 14 - Prob. 3PPCh. 14 - Prob. 4PPCh. 14 - Prob. 5PPCh. 14 - The game of Jump It consists of a board with n...Ch. 14 - Prob. 7PPCh. 14 - Prob. 8PP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- (Numerical) Write a program that tests the effectiveness of the rand() library function. Start by initializing 10 counters to 0, and then generate a large number of pseudorandom integers between 0 and 9. Each time a 0 occurs, increment the variable you have designated as the zero counter; when a 1 occurs, increment the counter variable that’s keeping count of the 1s that occur; and so on. Finally, display the number of 0s, 1s, 2s, and so on that occurred and the percentage of the time they occurred.arrow_forwardWrite a recursive function that converts a decimal number into a hex number as a string. The function header is: string decimalToHex(int value) Write a test program that prompts the user to enter a decimal number and displays its hex equivalent.arrow_forwardExponent y Catherine Arellano mplement a recursive function that returns he exponent given the base and the result. for example, if the base is 2 and the result is 3, then the output should be 3 because the exponent needed for 2 to become 8 is 3 (i.e. 23 = 8) nstructions: 1. In the code editor, you are provided with a main() function that asks the user for two integer inputs: 1. The first integer is the base 2. The second integer is the result 2. Furthermore, you are provided with the getExponent() function. The details of this function are the following: 1. Return type - int 2. Name - getExponent 3. Parameters 1. int - base 2. int - result 4. Description - this recursive function returns the exponent 5. Your task is to add the base case and the general case so it will work Score: 0/5 Overview 1080 main.c exponent.h 1 #include 2 #include "exponent.h" 3 int main(void) { 4 int base, result; 5 6 printf("Enter the base: "); scanf("%d", &base); 7 8 9 printf("Enter the result: ");…arrow_forward
- Write a recursive function that parses a hex number as a string into a decimal integer. The function header is: int hexToDecimal(const string& hexString) Write a test program that prompts the user to enter a hex string and displays its decimal equivalent.arrow_forward5. Given an integer n, you need to find the digital root of that integer using a recursive function. If n has only 1 digit, then its digital root is n. Otherwise, the digital root is equal to the digital root of the sum of the digits of n. The process continues until a single-digit number is reached. For example, digital_root of 576 is calculated as: digital_root (576) = 5+7+ 6 = 18 digital_root (18) = 1 +8=9 digital_root (9) = 9 Programming Language :- C So, the digital root of 576 is 9arrow_forwardType in Carrow_forward
- A recursive function in programming is a function that calls itself during its execution. The following two functions are examples of recursive functions: def function1(length): if length > 0: print(length) function1(length - 1) def function2(length): while length > 0: print(length) function2(length - 1) What can you say about the output of function1(3) and function2(3)? The two functions produce the same output 3 2 1. function1 produces the output: 3 2 1 and function2 runs infinitely. 3. function1 produces the output: 3 2 1 and function2 runs infinitely. 4. The two programs produce the same output: 1 2 3arrow_forwardWrite a recursive function that displays the number of even and odd digits in an integer using the following header: void evenAndOddCount(int value) Write a test program that prompts the user to enter an integer and displays the number of even and odd digits in it.arrow_forwardComputer Science need help plzarrow_forward
- For glass box testing of a recursive function, you should test cases where: a) the function returns without a recursive call, ie using a base case b) the function makes exactly one recursive call c) the function makes more than one recursive call d) all of thesearrow_forwardWrite a recursive function that converts a decimal number into a binary number as a string. The function header is: string decimalToBinary(int value) Write a test program that prompts the user to enter a decimal number and dis- plays its binary equivalent.arrow_forwardProblem Statement for Recursive Sum of Numbers Program Here is a simple recursive problem: Design a function that accepts a positive integer >= 1 and returns the sum of all the integers from 1 up to the number passed as an argument. For example, if 10 is passed as an argument, the function will return 55. Use recursion to calculate the sum. Write a second function which asks the user for the integer and displays the result of calling the function. Part 1. Understand the Problem To design a recursive function, you need to determine at least one base case (the base case returns a solution) and a general case (the general case calls the function again but passes a smaller version of the data as a parameter). To make this problem recursive think about it like this: If the integer is 1, the function will return 1. If the integer is 2, the function will return 1 + 2 = 3. If the integer is 3, the function will return 1 + 2 + 3 = 6. . . . For this problem, we will be sending in the "last"…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
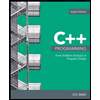
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
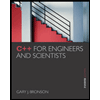
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr