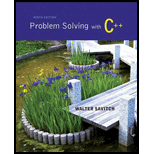
Problem Solving with C++ (9th Edition)
9th Edition
ISBN: 9780133591743
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 14, Problem 5PP
Program Plan Intro
Towers of Hanoi
Program plan:
- Include required header file.
- Declare the function for tower of Hanoi.
- Define main function.
- Declare variable for “n”.
- Initializes three post names in “char” data type.
- Create prompt statement for disks.
- Read the number of disks from user.
- Call the function “towersOfHanoi” with four parameters “n”, “post1”, “post2” and “post3”.
- Define “towersOfHanoi” function
- If the total count is equal to “1”, then display the movement of disk from given source post to destination post.
- Otherwise, recursively call the function “towersOfHanoi” with corresponding arguments.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
I need help to solve the following case, thank you
hi I would like to get help to resolve the following case
Could you help me to know features of the following concepts:
- defragmenting.
- dynamic disk.
- hardware RAID
Chapter 14 Solutions
Problem Solving with C++ (9th Edition)
Ch. 14.1 - Prob. 1STECh. 14.1 - Prob. 2STECh. 14.1 - Prob. 3STECh. 14.1 - Prob. 4STECh. 14.1 - Prob. 5STECh. 14.1 - If your program produces an error message that...Ch. 14.1 - Write an iterative version of the function cheers...Ch. 14.1 - Write an iterative version of the function defined...Ch. 14.1 - Prob. 9STECh. 14.1 - Trace the recursive solution you made to Self-Test...
Ch. 14.1 - Trace the recursive solution you made to Self-Test...Ch. 14.2 - What is the output of the following program?...Ch. 14.2 - Prob. 13STECh. 14.2 - Redefine the function power so that it also works...Ch. 14.3 - Prob. 15STECh. 14.3 - Write an iterative version of the one-argument...Ch. 14 - Prob. 1PCh. 14 - Prob. 2PCh. 14 - Write a recursive version of the search function...Ch. 14 - Prob. 4PCh. 14 - Prob. 5PCh. 14 - The formula for computing the number of ways of...Ch. 14 - Write a recursive function that has an argument...Ch. 14 - Prob. 3PPCh. 14 - Prob. 4PPCh. 14 - Prob. 5PPCh. 14 - The game of Jump It consists of a board with n...Ch. 14 - Prob. 7PPCh. 14 - Prob. 8PP
Knowledge Booster
Similar questions
- what is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forward
- Show all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forwardQuestion 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks Cole
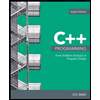
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
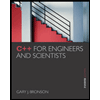
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
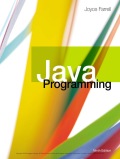
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
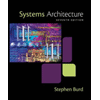
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
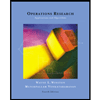
Operations Research : Applications and Algorithms
Computer Science
ISBN:9780534380588
Author:Wayne L. Winston
Publisher:Brooks Cole