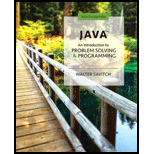
Concept explainers
Explanation of Solution
Complete program:
//Import required packages
import java.util.*;
//Definition of class Sum
public class Sum
{
//Definition of class main
public static void main(String[] args)
{
//Declare array "a"
int a[] = new int[7];
int value, key;
//Create an object for scanner class
Scanner sc = new Scanner(System.in);
//Get the array values
System.out.println("Enter the array values:");
//For loop to get the values from the user
for (int i = 0; i<a.length; i++)
{
//Get the values
value = sc.nextInt();
//Assign the values to the array
a[i] = value;
}
//Get the key value from the user
System.out.println("Enter key values to be search index:");
key = sc.nextInt();
//Call the method "trinarySearch()"
int result = ternarySearch(a, key, 0, a.length - 1);
System.out.println("Value found at position: " + result);
}
//Definition of method "trinarySearch()"
public static int ternarySearch(int data[], int target,
int front, int end)
{
//Declare the variable "res"
int res;
//Check whether the front is greater than end
if (front > end)
//Assign "-1" to "res"
res = -1;
else {
/*Divide the first half of the array by "3"*/
int firstHalf = (2 * front + end) / 3;
/*Divide the second half of the array by "3"*/
int secondHalf = (front + 2 * end) / 3;
/*Check whether the given number in the first half of the array*/
if (target == data[firstHalf])
;&#x...

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
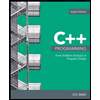
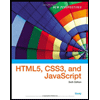
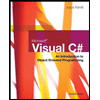
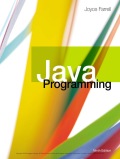
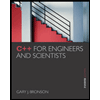