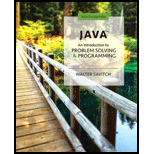
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 11, Problem 4PP
Program Plan Intro
Possible cuts in a bar
Program plan:
- Import required packages
- Define the class “Sequence”.
- Define the class “main”
- Create an object for scanner class
- Get the value from the user
- Call the method “c(number)”.
- Call the method “d(number)”.
- Create an object for scanner class
- Define the method “c()”.
- Declare required variables.
- Check whether “k” to “0”.
- Assign “1” to “res”.
- Otherwise, call the method “c(k-1)”.
- Return the value “res”.
- Define the method “d()”.
- Declare required variables.
- Check whether “k” to “0”.
- Assign “1” to “res”.
- Otherwise, assign “0” to “res”.
- Iterate till “i” is less than “k”.
- Call the method “d(i-1)*d(k-1)”.
- Return the value “res”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
We are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7).
A code breaker manages to factories 247 = 13 x 19
Determine Alice's secret key.
To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.
Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.
Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnn
Chapter 11 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 11.1 - What output will be produced by the following...Ch. 11.1 - What is the output produced by the following code?Ch. 11.1 - Write a recursive definition for the following...Ch. 11.1 - What is the output of the following code? public...Ch. 11.1 - Prob. 5STQCh. 11.1 - Complete the definition of the following method....Ch. 11.2 - Revise the method getCount in Listing 11.5 so that...Ch. 11.2 - Prob. 8STQCh. 11.2 - Prob. 9STQCh. 11.2 - Suppose you want me class ArraySearcher to work...
Ch. 11.2 - What Java statement will sort the following array,...Ch. 11.2 - How would you change the class MergeSort so that...Ch. 11.2 - How would you change the class MergeSort so that...Ch. 11.2 - If a value in an array of base type int occurs...Ch. 11.3 - Convert the following event handler to use the...Ch. 11 - What output will be produced by the following...Ch. 11 - What output will be produced by the following...Ch. 11 - Write a recursive method that will compute the...Ch. 11 - Write a recursive method that will compute the sum...Ch. 11 - Complete a recursive definition of the following...Ch. 11 - Write a recursive method that will compute the sum...Ch. 11 - Write a recursive method that will find and return...Ch. 11 - Prob. 8ECh. 11 - Write a recursive method that will compute...Ch. 11 - Suppose we want to compute the amount of money in...Ch. 11 - Prob. 11ECh. 11 - Write a recursive method that will count the...Ch. 11 - Write a recursive method that will remove all the...Ch. 11 - Write a recursive method that will duplicate each...Ch. 11 - Write a recursive method that will reverse the...Ch. 11 - Write a static recursive method that returns the...Ch. 11 - Write a static recursive method that returns the...Ch. 11 - One of the most common examples of recursion is an...Ch. 11 - A common example of a recursive formula is one to...Ch. 11 - A palindrome is a string that reads the same...Ch. 11 - A geometric progression is defined as the product...Ch. 11 - The Fibonacci sequence occurs frequently in nature...Ch. 11 - Prob. 4PPCh. 11 - Once upon a time in a kingdom far away, the king...Ch. 11 - There are n people in a room, where n is an...Ch. 11 - Prob. 7PPCh. 11 - Prob. 10PPCh. 11 - Prob. 12PP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
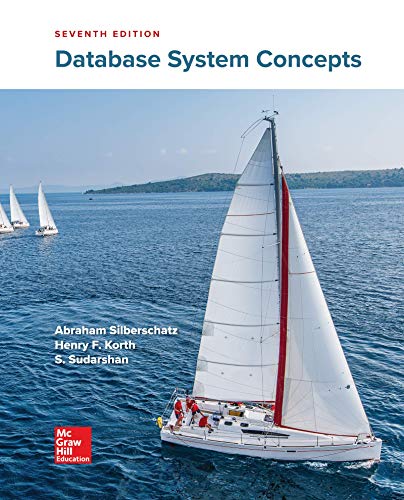
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
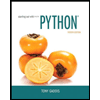
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
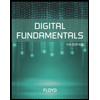
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
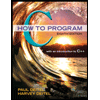
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
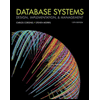
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
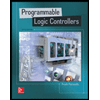
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Binary Numbers and Base Systems as Fast as Possible; Author: Techquikie;https://www.youtube.com/watch?v=LpuPe81bc2w;License: Standard YouTube License, CC-BY
Binary Number System; Author: Neso Academy;https://www.youtube.com/watch?v=w7ZLvYAi6pY;License: Standard Youtube License