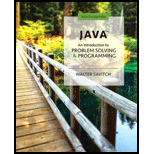
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 11, Problem 1P
Write a static recursive method that returns the number of digits in the integer passed to it as an argument of type int. Allow for both positive and negative arguments. For example, −120 has three digits. Do not count leading zeros. Embed the method in a
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Specifications: Part-1Part-1: DescriptionIn this part of the lab you will build a single operation ALU. This ALU will implement a bitwise left rotation. Forthis lab assignment you are not allowed to use Digital's Arithmetic components.IF YOU ARE FOUND USING THEM, YOU WILL RECEIVE A ZERO FOR LAB2!The ALU you will be implementing consists of two 4-bit inputs (named inA and inB) and one 4-bit output (named
out). Your ALU must rotate the bits in inA by the amount given by inB (i.e. 0-15).Part-1: User InterfaceYou are provided an interface file lab2_part1.dig; start Part-1 from this file.NOTE: You are not permitted to edit the content inside the dotted lines rectangle.Part-1: ExampleIn the figure above, the input values that we have selected to test are inA = {inA_3, inA_2, inA_1, inA_0} = {0, 1, 0,0} and inB = {inB_3, inB_2, inB_1, inB_0} = {0, 0, 1, 0}. Therefore, we must rotate the bus 0100 bitwise left by00102, or 2 in base 10, to get {0, 0, 0, 1}. Please note that a rotation left is…
How can I perform Laplace Transformation when using integration based on this? Where we convert time-based domain to frequency domain
what would be the best way I can explain the bevhoirs of Laplace and Inverse Transofrmation In MATLAB.
Chapter 11 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 11.1 - What output will be produced by the following...Ch. 11.1 - What is the output produced by the following code?Ch. 11.1 - Write a recursive definition for the following...Ch. 11.1 - What is the output of the following code? public...Ch. 11.1 - Prob. 5STQCh. 11.1 - Complete the definition of the following method....Ch. 11.2 - Revise the method getCount in Listing 11.5 so that...Ch. 11.2 - Prob. 8STQCh. 11.2 - Prob. 9STQCh. 11.2 - Suppose you want me class ArraySearcher to work...
Ch. 11.2 - What Java statement will sort the following array,...Ch. 11.2 - How would you change the class MergeSort so that...Ch. 11.2 - How would you change the class MergeSort so that...Ch. 11.2 - If a value in an array of base type int occurs...Ch. 11.3 - Convert the following event handler to use the...Ch. 11 - What output will be produced by the following...Ch. 11 - What output will be produced by the following...Ch. 11 - Write a recursive method that will compute the...Ch. 11 - Write a recursive method that will compute the sum...Ch. 11 - Complete a recursive definition of the following...Ch. 11 - Write a recursive method that will compute the sum...Ch. 11 - Write a recursive method that will find and return...Ch. 11 - Prob. 8ECh. 11 - Write a recursive method that will compute...Ch. 11 - Suppose we want to compute the amount of money in...Ch. 11 - Prob. 11ECh. 11 - Write a recursive method that will count the...Ch. 11 - Write a recursive method that will remove all the...Ch. 11 - Write a recursive method that will duplicate each...Ch. 11 - Write a recursive method that will reverse the...Ch. 11 - Write a static recursive method that returns the...Ch. 11 - Write a static recursive method that returns the...Ch. 11 - One of the most common examples of recursion is an...Ch. 11 - A common example of a recursive formula is one to...Ch. 11 - A palindrome is a string that reads the same...Ch. 11 - A geometric progression is defined as the product...Ch. 11 - The Fibonacci sequence occurs frequently in nature...Ch. 11 - Prob. 4PPCh. 11 - Once upon a time in a kingdom far away, the king...Ch. 11 - There are n people in a room, where n is an...Ch. 11 - Prob. 7PPCh. 11 - Prob. 10PPCh. 11 - Prob. 12PP
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
During the process of erasure, when the compiler encounters a class, interface, or method with a hound type par...
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
If the coefficient of static friction at contact points A and B is, s = 0.3, determine the maximum force P that...
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Write an SQL statement to display the breed, type, and DOB for all pets having the type Dog and the breed Std. ...
Database Concepts (8th Edition)
Determine the maximum tensile and compressive bending stress in the beam if it is subjected to a moment of M = ...
Mechanics of Materials (10th Edition)
What makes Python an interpreted programming language?
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- What IETF protocol is NetFlow associated with? Group of answer choices IPX/SPX IPIX HTTPS SSHarrow_forwardHow can I perform Laplace Transformation when using integration based on this?arrow_forwardWrite an example of a personal reflection of your course. - What you liked about the course. - What you didn’t like about the course. - Suggestions for improvement. Course: Information and Decision Sciences (IDS) The Reflection Paper should be 1 or 2 pages in length.arrow_forward
- using r languagearrow_forwardI need help in explaining how I can demonstrate how the Laplace & Inverse transformations behaves in MATLAB transformation (ex: LIke in graph or something else)arrow_forwardYou have made the Web solution with Node.js. please let me know what problems and benefits I would experience while making the Web solution here, as compared to any other Web solution you have developed in the past. what problems and benefits/things to keep in mind as someone just learningarrow_forward
- PHP is the server-side scripting language. MySQL is used with PHP to store all the data. EXPLAIN in details how to install and run the PHP/MySQL on your computer. List the issues and challenges I may encounter while making this set-up? why I asked: I currently have issues logging into http://localhost/phpmyadmin/ and I tried using the command prompt in administrator to reset the password but I got the error LOCALHOST PORT not found.arrow_forwardHTML defines content, CSS defines layout, and JavaScript adds logic to the website on the client side. EXPLAIN IN DETAIL USING an example.arrow_forwardusing r languangearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
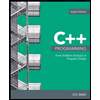
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
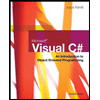
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
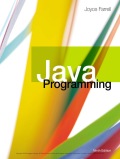
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
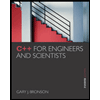
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
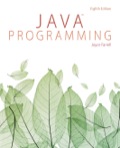
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Introduction to Big O Notation and Time Complexity (Data Structures & Algorithms #7); Author: CS Dojo;https://www.youtube.com/watch?v=D6xkbGLQesk;License: Standard YouTube License, CC-BY