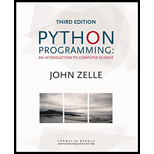
Modified gpasort program
Program plan:
- Import the necessary modules in “grade_sort.py” file.
- Define the “make_Student()” function,
- Returns student record values to the caller.
- Define the “read_Students()” function,
- Returns the list of student record to the caller.
- Define the “write_Students()” function,
- Write the student record.
- Define the “main()” function,
- Get the input file.
- Read the students record for the input file.
- Make a “while” loop for “True”.
- Get the type of input to sort.
- Get the type of ordering from the user.
- Check whether the type is “GPA” using “if”.
- If it is true, sort the data based on the “GPA”.
- Rename the file name.
- Check whether the ordering is "D"
-
- If it is true, reverse the data.
- Use “break” to exit.
- Check whether the type is “name” using “elif”.
- If it is true, sort the data based on the “name”.
- Rename the file name.
- Check whether the ordering is "D"
-
- If it is true, reverse the data.
- Use “break” to exit.
- Check whether the type is “credits” using “if”.
- If it is true, sort the data based on the “credits”.
- Rename the file name.
- Check whether the ordering is "D"
-
- If it is true, reverse the data.
- Use “break” to exit.
-
-
- Write the data into the output file.
-
- Check whether the type is “GPA” using “if”.
- Create a class Student in “gpa.py” file,
- Define the “_init_()” method.
- Assign name hours and GPoints.
-
-
-
- Define the “get_Name()” method.
- Return the name.
- Define the “get_Hours()” method.
- Return hours.
- Define the “getQ_Points()” method.
- Return GPoints.
- Define the “gpa()” method.
- Return gpa
- Define the “make_Student()” method.
- Return name, hours, and grade points.
- Define the “main()” function.
- Define the “get_Name()” method.
-
-
-
- Assign name hours and GPoints.
- Define the “_init_()” method.
- Call the “main()” function.

Explanation of Solution
Program:
File name: “gpasort.py”
#Import required module
from gpa import Student
#Define the function make_Student()
def make_Student(info_Str):
#Make multiple assignment
Name, Hours, Gpoints = info_Str.split("\t")
#Return constructor
return Student(Name, Hours, Gpoints)
#Define the function read_Students()
def read_Students(file_name):
#Open the input file for reading
in_file = open(file_name, 'r')
#Create an empty list
Students = []
#Create for loop to iterate over all lines in a file
for line in in_file:
#Append the line in a list
Students.append(make_Student(line))
#Close the input file
in_file.close()
#Return the list
return Students
#Define the function write_Students()
def write_Students(Students, file_name):
#Open output file to write
out_file = open(file_name, 'w')
#Create a for loop to iterate over list
for s in Students:
#Print output
print("{0}\t{1}\t{2}".format(s.get_Name(), s.get_Hours(), s.getQ_Points()), file = out_file)
#Close the output file
out_file.close()
#Define the main() function
def main():
#Print the string
print("This program sorts student grade information by GPA, name, or credits.")
#Get the input file
file_name = 'gpa1.txt'
#Assign the data return from read_Students()
data = read_Students(file_name)
#Create "while" loop
while True:
#Get the type
x = (input('Type "GPA", "name", or "credits" >>> '))
#Get the type of ordering
m = (input('Type "A" for ascending, "D" for descending.'))
#Check whether the type is "GPA"
if x == 'GPA':
#Sort the data based on the gpa
data.sort(key=Student.gpa)
#Rename the file name
s = "_(GPA)"
#Check whether the ordering is "D"
if m == "D":
#Reverse the data
data.reverse()
#Use break to exit
break
#Check whether the type is "name"
elif x == 'name':
#Sort the data based on the name
data.sort(key=Student.get_Name)
#Rename the file name
s = "_(name)"
#Check whether the ordering is "D"
if m == "D":
#Reverse the data
data.reverse()
#Use break to exit
break
#Check whether the type is "credits"
elif x == 'credits':
#Sort the data based on the credits
data.sort(key=Student.getQ_Points)
#Rename the file name
s = "_(credits)"
#Check whether the ordering is "D"
if m == "D":
#Reverse the data
data.reverse()
#Use break to exit
break
#Otherwise
else:
#Print the string
print("Please try again.")
#Assign the output file
filename = "GPA2" + s + ".py"
#Write the data into output file
write_Students(data, filename)
#Print output file
print("The data has been written to", filename)
#Call the main function
if __name__ == '__main__': main()
File name: “gpa.py”
#Create a class Student
class Student:
#Define _init_() method
def __init__(self, Name, Hours, Gpoints):
self.Name = Name
self.Hours = float(Hours)
self.Gpoints = float(Gpoints)
#Define get_Name() method
def get_Name(self):
#Return the name
return self.Name
#Define get_Hours()
def get_Hours(self):
#return hours
return self.Hours
#Define getQ_Points()
def getQ_Points(self):
#return grade points
return self.Gpoints
#Define the funcition gpa()
def gpa(self):
#return the value
return self.Gpoints / self.Hours
#Define the function make_Student()
def make_Student(info_Str):
#Make multiple assignment
Name, Hours, Gpoints = info_Str.split("\t")
#Return the constructor
return Student(Name, Hours, Gpoints)
#Define the main() function
def main():
#Open the input file for reading
file_name = input("Enter the name of the grade file: ")
in_file = open(file_name, 'r')
#Set best to the record for the first student in the file
best = make_Student(in_file.readline())
#Process lines of the file using "for" loop
for line in in_file:
#Make the line of file into a student record
s = make_Student(line)
#Checck whether the student is best so far
if s.gpa() > best.gpa():
#Assign the best student record
best = s
#Close the input file
in_file.close()
#Print information about the best student
print("The best student is:", best.get_Name())
print("Hours:", best.get_Hours())
print("GPA:", best.gpa())
if __name__ == '__main__':
#Call the main() function
main()
Contents of “gpa1.txt”
Adams, Henry 127 228
Computewell, Susan 100 400
DibbleBit, Denny 18 41.5
Jones, Jim 48.5 155
Smith, Frank 37 125.33
Screenshot of output file “GPA2.py” before execution:
Output:
This program sorts student grade information by GPA, name, or credits.
Type "GPA", "name", or "credits" >>> name
Type "A" for ascending, "D" for descending.D
The data has been written to GPA2_(name).py
>>>
Screenshot of output file “GPA2_(name).py after execution:
Additional output:
This program sorts student grade information by GPA, name, or credits.
Type "GPA", "name", or "credits" >>> GPA
Type "A" for ascending, "D" for descending.D
The data has been written to GPA2_(GPA).py
>>>
Screenshot of output file “GPA2_(gpa).py after execution:
Want to see more full solutions like this?
Chapter 11 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- This week we will be building a regression model conceptually for our discussion assignment. Consider your current workplace (or previous/future workplace if not currently working) and answer the following set of questions. Expand where needed to help others understand your thinking: What is the most important factor (variable) that needs to be predicted accurately at work? Why? Justify its selection as your dependent variable.arrow_forwardAccording to best practices, you should always make a copy of a config file and add a date to filename before editing? Explain why this should be done and what could be the pitfalls of not doing it.arrow_forwardIn completing this report, you may be required to rely heavily on principles relevant, for example, the Work System, Managerial and Functional Levels, Information and International Systems, and Security. apply Problem Solving Techniques (Think Outside The Box) when completing. should reflect relevance, clarity, and organisation based on research. Your research must be demonstrated by Details from the scenario to support your analysis, Theories from your readings, Three or more scholarly references are required from books, UWIlinc, etc, in-text or narrated citations of at least four (4) references. “Implementation of an Integrated Inventory Management System at Green Fields Manufacturing” Green Fields Manufacturing is a mid-sized company specialising in eco-friendly home and garden products. In recent years, growing demand has exposed the limitations of their fragmented processes and outdated systems. Different departments manage production schedules, raw material requirements, and…arrow_forward
- 1. Create a Book record that implements the Comparable interface, comparing the Book objects by year - title: String > - author: String - year: int Book + compareTo(other Book: Book): int + toString(): String Submit your source code on Canvas (Copy your code to text box or upload.java file) > Comparable 2. Create a main method in Book record. 1) In the main method, create an array of 2 objects of Book with your choice of title, author, and year. 2) Sort the array by year 3) Print the object. Override the toString in Book to match the example output: @Javadoc Declaration Console X Properties Book [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspo [Book: year=1901, Book: year=2010]arrow_forwardQ5-The efficiency of a 200 KVA, single phase transformer is 98% when operating at full load 0.8 lagging p.f. the iron losses in the transformer is 2000 watt. Calculate the i) Full load copper losses ii) half load copper losses and efficiency at half load. Ans: 1265.306 watt, 97.186%arrow_forward2. Consider the following pseudocode for partition: function partition (A,L,R) pivotkey = A [R] t = L for i L to R-1 inclusive: if A[i] A[i] t = t + 1 end if end for A [t] A[R] return t end function Suppose we call partition (A,0,5) on A=[10,1,9,2,8,5]. Show the state of the list at the indicated instances. Initial A After i=0 ends After 1 ends After i 2 ends After i = 3 ends After i = 4 ends After final swap 10 19 285 [12 pts]arrow_forward
- .NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardusing r languagearrow_forward8. Cash RegisterThis exercise assumes you have created the RetailItem class for Programming Exercise 5. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods: A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list. A method named get_total that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list. A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list. A method named clear that should clear the CashRegister object’s internal list. Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the…arrow_forward
- 5. RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95Item #3 Shirt 20 24.95arrow_forwardFind the Error: class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forwardFind the Error: class Pet def __init__(self, name, animal_type, age) self.__name = name; self.__animal_type = animal_type self.__age = age def set_name(self, name) self.__name = name def set_animal_type(self, animal_type) self.__animal_type = animal_typearrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
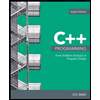
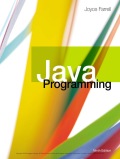
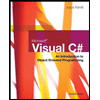
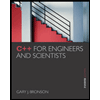
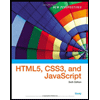