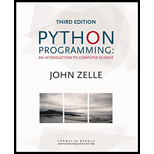
Modified gpasort program
Program plan:
- Import the necessary modules in “grade_sort.py” file.
- Define the “make_Student()” function,
- Returns student record values to the caller.
- Define the “read_Students()” function,
- Returns the list of student record to the caller.
- Define the “write_Students()” function,
- Write the student record.
- Define the “sort()” function,
- Create empty list.
- Create “for” loop,
- Assign the data from the file.
- Append the data to the new list using append() method.
- Call the function “sort()” to sort the data.
- Call the function “reverse()” to reverse the data.
- Return new list.
- Define the “main()” function,
- Assign the input file.
- Read the students record from the input file.
- Sort the data by calling “sort()” function.
- Assign the output file.
- Call the function “write_Students()”.
- Call the “main()” function.
- Create a class Student in “gpa.py” file,
- Define the “_init_()” method.
- Assign name hours and GPoints.
- Define the “get_Name()” method.
- Return the name.
- Define the “get_Hours()” method.
- Return hours.
- Define the “getQ_Points()” method.
- Return GPoints.
- Define the “gpa()” method.
- Return gpa
- Define the “make_Student()” method.
- Return name, hours, and grade points.
- Define the “main()” function.
- Define the “get_Name()” method.
- Assign name hours and GPoints.
- Define the “_init_()” method.
- Call the “main()” function.

Explanation of Solution
Program:
File name: “gpa_sort.py”
#Import required module
from gpa import Student
#Define the function make_Student()
def make_Student(info_Str):
#Make multiple assignment
Name, Hours, Gpoints = info_Str.split("\t")
#Return constructor
return Student(Name, Hours, Gpoints)
#Define the function read_Students()
def read_Students(file_name):
#Open the input file for reading
in_file = open(file_name, 'r')
#Create an empty list
Students = []
#Create for loop to iterate over all lines in a file
for line in in_file:
#Append the line in a list
Students.append(make_Student(line))
#Close the input file
in_file.close()
#Return the list
return Students
#Define the function write_Students()
def write_Students(Students, file_name):
#Open output file to write
out_file = open(file_name, 'w')
#Create a for loop to iterate over list
for s in Students:
#Print output
print("{0}\t{1}\t{2}".format(s[1],
s[2], s[3]), file = out_file)
#Close the output file
out_file.close()
#Define the function
def sort(Students):
#Create empty list
new_Data = []
#Create for loop
for i in range(len(Students)):
#Assign tuple
x = (Students[i].gpa(), Students[i].get_Name(),
Students[i].get_Hours(), Students[i].getQ_Points())
#Append the data at the end of new list
new_Data.append(x)
#Call the function "sort()" to sort the data
new_Data.sort()
#Call the function "reverse()" to reverse the data
new_Data.reverse()
#Return new list
return new_Data
#Define main() function
def main():
#Print the string
print("This program sorts student grade information by GPA")
#Assign the file name
file_name = "gpa1.txt"
#Read the data from a file
data = read_Students(file_name)
#Sort the data
data = sort(data)
#Assign the file name
file_name = "gpa_(sort1).txt"
#Call the function
write_Students(data, file_name)
if __name__ == '__main__':
#Call the main() function
main()
File name: “gpa.py”
#Create a class Student
class Student:
#Define _init_() method
def __init__(self, Name, Hours, Gpoints):
self.Name = Name
self.Hours = float(Hours)
self.Gpoints = float(Gpoints)
#Define get_Name() method
def get_Name(self):
#Return the name
return self.Name
#Define get_Hours()
def get_Hours(self):
#return hours
return self.Hours
#Define getQ_Points()
def getQ_Points(self):
#return grade points
return self.Gpoints
#Define the function gpa()
def gpa(self):
#return the value
return self.Gpoints / self.Hours
#Define the function make_Student()
def make_Student(info_Str):
#Make multiple assignment
Name, Hours, Gpoints = info_Str.split("\t")
#Return the constructor
return Student(Name, Hours, Gpoints)
#Define the main() function
def main():
#Open the input file for reading
file_name = input("Enter the name of the grade file: ")
in_file = open(file_name, 'r')
#Set best to the record for the first student in the file
best = make_Student(in_file.readline())
#Process lines of the file using "for" loop
for line in in_file:
#Make the line of file into a student record
s = make_Student(line)
#Checck whether the student is best so far
if s.gpa() > best.gpa():
#Assign the best student record
best = s
#Close the input file
in_file.close()
#Print information about the best student
print("The best student is:", best.get_Name())
print("Hours:", best.get_Hours())
print("GPA:", best.gpa())
if __name__ == '__main__':
#Call the main() function
main()
Contents of “gpa1.txt”
Adams, Henry 127 228
Computewell, Susan 100 400
DibbleBit, Denny 18 41.5
Jones, Jim 48.5 155
Smith, Frank 37 125.33
Output:
This program sorts student grade information by GPA
>>>
Screenshot of output file “gps_(sort1).txt after execution:
Want to see more full solutions like this?
Chapter 11 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- What is a functional decomposition diagram? what is a good example of a high level task being broken down into tasks in at least two lower levels (three levels in all).arrow_forwardWhat are the advantages to using a Sytems Analysis and Design model like the SDLC vs. other approaches?arrow_forward3. Problem Description: Define the Circle2D class that contains: Two double data fields named x and y that specify the center of the circle with get methods. • A data field radius with a get method. • A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius. • A constructor that creates a circle with the specified x, y, and radius. • A method getArea() that returns the area of the circle. • A method getPerimeter() that returns the perimeter of the circle. • • • A method contains(double x, double y) that returns true if the specified point (x, y) is inside this circle. See Figure (a). A method contains(Circle2D circle) that returns true if the specified circle is inside this circle. See Figure (b). A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this circle. See the figure below. р O со (a) (b) (c)< Figure (a) A point is inside the circle. (b) A circle is inside another circle. (c) A circle overlaps another…arrow_forward
- 1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.arrow_forwardSecurity in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.arrow_forwardPlease include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forward
- Object-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forwardWhen using functions in python, it allows us tto create procedural abstractioons in our programs. What are 5 major benefits of using a procedural abstraction in python?arrow_forward
- Find the error, assume data is a string and all variables have been declared. for ch in data: if ch.isupper: num_upper = num_upper + 1 if ch.islower: num_lower = num_lower + 1 if ch.isdigit: num_digits = num_digits + 1 if ch.isspace: num_space = num_space + 1arrow_forwardFind the Error: date_string = input('Enter a date in the format mm/dd/yyyy: ') date_list = date_string.split('-') month_num = int(date_list[0]) day = date_list[1] year = date_list[2] month_name = month_list[month_num - 1] long_date = month_name + ' ' + day + ', ' + year print(long_date)arrow_forwardFind the Error: full_name = input ('Enter your full name: ') name = split(full_name) for string in name: print(string[0].upper(), sep='', end='') print('.', sep=' ', end='')arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
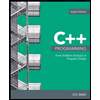
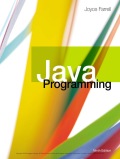
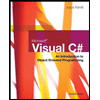
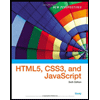
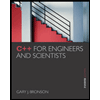