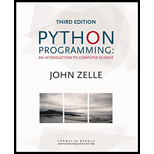
Concept explainers
Modified gpasort program
Program plan:
- Import the necessary modules in “grade_sort.py” file.
- Define the “make_Student()” function,
- Returns student record values to the caller.
- Define the “read_Students()” function,
- Returns the list of student record to the caller.
- Define the “write_Students()” function,
- Write the student record.
- Define the “main()” function,
- Get the input file.
- Read the students record for the input file.
- Make a “while” loop for “True”.
- Get the type of input to sort.
- Check whether the type is “GPA” using “if”.
- If it is true, sort the data based on the “GPA”.
- Use “break” to exit.
- Check whether the type is “name” using “elif”.
- If it is true, sort the data based on the “name”.
- Use “break” to exit.
- Check whether the type is “credits” using “if”.
- If it is true, sort the data based on the “credits”.
- Use “break” to exit.
-
- Write the data into the output file.
- Check whether the type is “GPA” using “if”.
- Get the type of input to sort.
- Create a class Student in “gpa.py” file,
- Define the “_init_()” method.
- Assign name hours and GPoints.
-
-
- Define the “get_Name()” method.
- Return the name.
- Define the “get_Hours()” method.
- Return hours.
- Define the “getQ_Points()” method.
- Return GPoints.
- Define the “gpa()” method.
- Return gpa
- Define the “make_Student()” method.
- Return name, hours, and grade points.
- Define the main() function.
- Define the “get_Name()” method.
-
-
- Assign name hours and GPoints.
- Define the “_init_()” method.
- Call the “main()” function.

Explanation of Solution
Program:
File name: “gpasort.py”
#Import required module
from gpa import Student
#Deine the function make_Student()
def make_Student(info_Str):
#Make multiple assignment
Name, Hours, Gpoints = info_Str.split("\t")
#Return constructor
return Student(Name, Hours, Gpoints)
#Define the function read_Students()
def read_Students(file_name):
#Openthe input file for reading
in_file = open(file_name, 'r')
#Create an empty list
Students = []
#Create ffor loop to iterate over all lines in a file
for line in in_file:
#Append the line in a list
Students.append(make_Student(line))
#Close the input file
in_file.close()
#Return the list
return Students
#Define the function write_Students()
def write_Students(Students, file_name):
#Open output file to write
out_file = open(file_name, 'w')
#Create a for loop to iterate over list
for s in Students:
#Print output
print("{0}\t{1}\t{2}".format(s.get_Name(), s.get_Hours(), s.getQ_Points()), file = out_file)
#Close the output file
out_file.close()
#Define the main() function
def main():
#Print the string
print("This program sorts student grade information by GPA, name, or credits.")
#Get the input file
file_name = 'gpa1.txt'
#Assign the data return from read_Students()
data = read_Students(file_name)
#Create "while" loop
while True:
#Get the type
x = (input('Type "GPA", "name", or "credits" >>> '))
#Check whether the type is "GPA"
if x == 'GPA':
#Sort the data based on the gpa
data.sort(key=Student.gpa)
s = "_(GPA)"
#Use break to exit
break
#Check whether the type is "name"
elif x == 'name':
#Sort the data based on the name
data.sort(key=Student.get_Name)
s = "_(name)"
#Use break to exit
break
#Check whether the type is "credits"
elif x == 'credits':
#Sort the data based on the credit points
data.sort(key=Student.getQ_Points)
s = "_(credits)"
#Use break to exit
break
#Otherwise
else:
#Print the string
print("Please try again.")
#Assign the output file
file_name = "GPA2" + s + ".py"
#Writ the data into output file
write_Students(data, file_name)
#Print the output file
print("The data has been written to", file_name)
#Call main() function
if __name__ == '__main__': main()
File name: “gpa.py”
#Create a class Student
class Student:
#Define _init_() method
def __init__(self, Name, Hours, Gpoints):
self.Name = Name
self.Hours = float(Hours)
self.Gpoints = float(Gpoints)
#Define get_Name() method
def get_Name(self):
#Return the name
return self.Name
#Define get_Hours()
def get_Hours(self):
#return hours
return self.Hours
#Define getQ_Points()
def getQ_Points(self):
#return grade points
return self.Gpoints
#Define the function gpa()
def gpa(self):
#return the value
return self.Gpoints / self.Hours
#Define the function make_Student()
def make_Student(info_Str):
#Make multiple assignment
Name, Hours, Gpoints = info_Str.split("\t")
#Return the constructor
return Student(Name, Hours, Gpoints)
#Define the main() function
def main():
#Open the input file for reading
file_name = input("Enter the name of the grade file: ")
in_file = open(file_name, 'r')
#Set best to the record for the first student in the file
best = make_Student(in_file.readline())
#Process lines of the file using "for" loop
for line in in_file:
#Make the line of file into a student record
s = make_Student(line)
#Check whether the student is best so far
if s.gpa() > best.gpa():
#Assign the best student record
best = s
#Close the input file
in_file.close()
#Print information about the best student
print("The best student is:", best.get_Name())
print("Hours:", best.get_Hours())
print("GPA:", best.gpa())
if __name__ == '__main__':
#Call the main() function
main()
Contents of “gpa1.txt”
Adams, Henry 127 228
Computewell, Susan 100 400
DibbleBit, Denny 18 41.5
Jones, Jim 48.5 155
Smith, Frank 37 125.33
Screenshot of output file “GPA2.py” before execution:
Output:
This program sorts student grade information by GPA, name, or credits.
Type "GPA", "name", or "credits" >>> name
The data has been written to GPA2_(name).py
>>>
Screenshot of output file “GPA2_(name).py after execution:
Additional output:
This program sorts student grade information by GPA, name, or credits.
Type "GPA", "name", or "credits" >>> GPA
The data has been written to GPA2_(GPA).py
>>>
Screenshot of output file “GPA2_(gpa).py after execution:
Want to see more full solutions like this?
Chapter 11 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- solve this questions for me .arrow_forwarda) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?arrow_forwardConsider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as thearrow_forward
- whats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forwardWhat is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forward
- Consider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
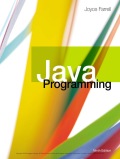
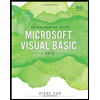
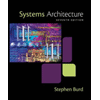
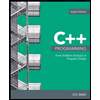
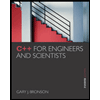