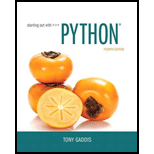
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 11, Problem 3AW
Look at the following class definition:
class Beverage:
def _ _ init _ _ (self, bev_name):
self._ _bev_name = bev_name
Write the code for a class named cola that is a subclass of the Beverage class.
The cola class’s _ _init_ _ method should call the Beverage class’s _ _init_ _ method, passing ‘cola’ as an argument.
Expert Solution & Answer

Learn your wayIncludes step-by-step video

schedule04:42
Students have asked these similar questions
Parent Class: Food
Write a parent class called Food. A food is described by a name, the number of grams of sugar (as a whole number), and the number of grams of sodium (as a whole number).
Core Class Components
For the Food class, write:
the complete class header
the instance data variables
a constructor that sets the instance data variables based on parameters
getters and setters; use validity checking on the parameters where appropriate
a toString method that returns a text representation of a Food object that includes all three characteristics of the food
Class-Specific Method
Write a method that calculates what percent of the daily recommended amount of sugar is contained in a food. The daily recommended amount might change, so the method takes in the daily allowance and then calculates the percentage.
For example, let's say a food had 6 grams of sugar. If the daily allowance was 24 grams, the percent would be 0.25. For that same food, if the daily allowance was 36 grams, the…
T/F: Instance variables are shared by all the instances of the class.
T/F: The scope of instance and static variables is the entire class. They can be declared anywhere inside a class.
T/F: To declare static variables, constants, and methods, use the static modifier.
Child Class: Vegetable
Write a child class called Vegetable. A vegetable is described by a name, the number of grams of sugar (as a whole number), the number of grams of sodium (as a whole number), and whether or not the vegetable is a starch.
Core Class Components
For the Vegetable class, write:
the complete class header
the instance data variables
a constructor that sets the instance data variables based on parameters
getters and setters; use instance data variables where appropriate
a toString method that returns a text representation of a Vegetable object that includes all four characteristics of the vegetableJava
Chapter 11 Solutions
Starting Out with Python (4th Edition)
Ch. 11.1 - In this section, we discussed superclasses and...Ch. 11.1 - Prob. 2CPCh. 11.1 - What does a subclass inherit from its superclass?Ch. 11.1 - Look at the following code, which is the first...Ch. 11.2 - Look at the following class definitions: class...Ch. 11 - In an inheritance relationship, the ___________ is...Ch. 11 - In an inheritance relationship, the _________ is...Ch. 11 - Suppose a program uses two classes: Airplane and...Ch. 11 - This characteristic of object-oriented programming...Ch. 11 - Prob. 5MC
Ch. 11 - Polymorphism allows you to write methods in a...Ch. 11 - It is not possible to call a superclasss _ _init_...Ch. 11 - A subclass can have a method with the same name as...Ch. 11 - Only the _ _init_ _method can be overridden.Ch. 11 - You cannot use the isinstance function to...Ch. 11 - What does a subclass inherit from its superclass?Ch. 11 - Look at the following class definition. What is...Ch. 11 - Prob. 3SACh. 11 - Write the first line of the definition for a...Ch. 11 - Look at the following class definitions: class...Ch. 11 - Look at the following class definition: class...Ch. 11 - Employee and ProductionWorker Classes Write an...Ch. 11 - ShiftSupervisor Class In a particular factory, a...Ch. 11 - Person and Customer Classes The Person and...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
For each of the following activities, give a PEAS description of the task environment and characterize it in te...
Artificial Intelligence: A Modern Approach
Set a breakpoint in the first line of the sendMai1 Item method in the MailClient class. Then invoke this method...
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
How do you identify the potential classes in a problem domain description?
Starting Out with C++: Early Objects
If a function doesnt return a value, the word _____ will appear as its return type.
Starting Out with C++: Early Objects (9th Edition)
Repeat Question 25 for the method deleteCurrentNode.
Java: An Introduction to Problem Solving and Programming (7th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Parking Ticket SimulatorFor this assignment you will design a set of classes that work together to simulate apolice officer issuing a parking ticket. The classes you should design are:• The Parkedcar Class: This class should simulate a parked car. The class's respon-sibilities are:To know the car's make, model, color, license number, and the number of min-utes that the car has been parkedThe BarkingMeter Class: This class should simulate a parking meter. The class'sonly responsibility is:- To know the number of minutes of parking time that has been purchased• The ParkingTicket Class: This class should simulate a parking ticket. The class'sresponsibilities areTo report the make, model, color, and license number of the illegally parked carTo report the amount of the fine, which is $2S for the first hour or part of anhour that the car is illegally parked, plus $10 for every additional hour or part ofan hour that the car is illegally parkedTo report the name and badge number of the police…arrow_forwardemployee and production worker classes write an employee class that keeps data attributes for the following pieces of information: • employee name • employee number next, write a class named productionworker that is a subclass of the employee class. the productionworker class should keep data attributes for the following information: • shift number (an integer, such as 1, 2, or 3) • hourly pay rate the workday is divided into two shifts: day and night. the shift attribute will hold an integer value representing the shift that the employee works. the day shift is shift 1 and the night shift is shift 2. write the appropriate accessor and mutator methods for each class. once you have written the classes, write a program that creates an object of the productionworker class and prompts the user to enter data for each of the object’s data attributes. store the data in the object, then use the object’s accessor methods to retrieve it and display it on the screen. satak overfallowarrow_forwardT/F A method defined in a class can access the class' instance data without needing to pass them as parameters or declare them as local variables.arrow_forward
- Dynamic Games Instructions: Write a class called Game that contains a video game’s name, genre, and difficultyLevel. Include a default constructor and destructor for the class. The constructor should print out the following message: “Creating a new game”. The destructor should print out the following message: “In the Game destructor.” Include appropriate get/set functions for the class. In main(), prompt the user to enter the number of games he or she has played in the past year. Dynamically create a built-in array based on this number (not a vector or object of the array class) to hold pointers to Game objects. Construct a loop in main() that executes once for each of the number of games that the user indicated. Within this loop, ask the user to enter the name and genre of each game. Using a random number generator, generate a difficultyLevel between 1-10 (inclusive). Seed this random number generator with 100. Next, dynamically create a Game object (remember that this…arrow_forward8. Circle ClassWrite a Circle class that has the following member variables:• radius: a double• pi: a double initialized with the value 3.14159The class should have the following member functions:• Default Constructor. A default constructor that sets radius to 0.0.• Constructor. Accepts the radius of the circle as an argument.• setRadius. A mutator function for the radius variable.• getRadius. An accessor function for the radius variable.• getArea. Returns the area of the circle, which is calculated as area = pi * radius * radius• getDiameter. Returns the diameter of the circle, which is calculated as diameter = radius * 2• getCircumference. Returns the circumference of the circle, which is calculated as circumference = 2 * pi * radiusWrite a program that demonstrates the Circle class by asking the user for the circle’s radius, creating a Circle object, and then reporting the circle’s area, diameter, and circumference.…arrow_forwardNewspaperSubscriber class- create an abstract class named NewspaperSubscriber with attributes to contain the subscriber’s street address and the subscription rate. Include get and set methods for both these attributes. The set method for the rate is abstract. The setAddress method must prompt the user to enter the subscriber’s address through the use of a dialog box. Create a constructor for the base class. Create a toString() method that concaternates and returns the subscriber’s street address and rate for display. Create 3 child classes named SevenDaySubscriber, Weekday Subscriber, and Weekend Subscriber. Each child class has an additional attribute called subType, which is a String, that will store the type of newspaper subscription. Create a setType method that will set the type of subscriber as follows: “Seven Day”, “Weekday”, or“Weekend”. Create a setRate method for each child class that sets the rate as follows: a SevenDaysubscriber pays R18.00 per week for his newspapers, a…arrow_forward
- Employee class Write a Python program employee.py that computes the cumulative salary of employees based on the length of their contract and their annual merit rate. Your program must include a class Employee with the following requirements: Class attributes: name: the name of the employee position: the position of the employee start_salary: the starting salary of the employee annual_rate: the annual merit rate on the salary contract_years: the number of years of salary Class method: get_cumulative_salary(): calculates and returns the cumulative salary of an employee based on the number of contract years. Round the cumulative salary to two digits after the decimal point. Example: If start_salary = 100000, annual_rate = 5% and contract_years = 3: Then the cumulative salary should be : 100000 + 105000 + 110250 = 315250 Outside of the class Employee, the program should have two functions: Function name Function description Function input(s) Function output(s) / return…arrow_forwardC# languageWrite a program to create a class employee, it consist of ID, name, department and address. All employees belongs to “Computer Science” department and it can never be changed by any means. Employee ID is initialized only once when Employee object is created, any further attempt to change ID should be failed. Class must have a 3 parameterized constructor to set values and two methods: print(): to display all the data of a particular employee totalObjects(): to count and print total number of objects that has been created In Main(), create atleast two objects of employee class, display their records by calling print() function and also print the total number of objects that has been created. [this question is continued on next page] Sample Main Method: static void Main(string[] args) { Employee obj1 = new Employee(1, "Zubair", "Karachi"); obj1.print(); Employee obj2 = new Employee(2, "Nabeel", "Islamabad"); obj2.print();…arrow_forwardJava:arrow_forward
- Assignment:The BankAccount class models an account of a customer. A BankAccount has the followinginstance variables: A unique account id sequentially assigned when the Bank Account is created. A balance which represents the amount of money in the account A date created which is the date on which the account is created.The following methods are defined in the BankAccount class: Withdraw – subtract money from the balance Deposit – add money to the balance Inquiry on:o Balanceo Account ido Date createdarrow_forwardWe use the _____ operator to create an instance of (object in) a particular class.arrow_forwardCar ClassWrite a class named Car that has the following fields:• yearModel. The yearModel field is an int that holds the car’s year model.• make. The make field references a String object that holds the make of the car.• speed. The speed field is an int that holds the car’s current speed. In addition, the class should have the following constructor and other methods.• Constructor. The constructor should accept the car’s year model and make as arguments. These values should be assigned to the object’s yearModel and make fields. The constructor should also assign 0 to the speed field.• Accessors. Appropriate accessor methods should get the values stored in an object’s yearModel, make, and speed fields.• accelerate. The accelerate method should add 5 to the speed field each time it is called.• brake. The brake method should subtract 5 from the speed field each time it is called.Demonstrate the class in a program that creates a Car object, and then calls the accelerate method five…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
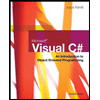
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY