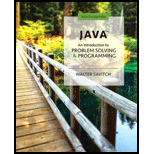
Explanation of Solution
Modified
The below highlighted parts are the modified codes in the server and client programs.
Server program:
//import required header files
import java.util.Scanner;
import java.io.InputStreamReader;
import java.io.DataOutputStream;
import java.io.PrintWriter;
import java.net.Socket;
import java.net.ServerSocket;
//definition of "SocketServer" class
public class SocketServer
{
//definition of main method
public static void main(String[] args)
{
//declare the objects for the classes
Scanner inputStream = null;
PrintWriter outputStream = null;
ServerSocket serverSocket = null;
//try block
try
{
// wait for connection on port 6789
System.out.println("Waiting for a connection.");
serverSocket = new ServerSocket(6789);
Socket socket = serverSocket.accept();
// connection made, set up streams
inputStream = new Scanner(new InputStreamReader(socket.getInputStream()));
outputStream = new PrintWriter(new DataOutputStream(socket.getOutputStream()));
//get the input from the client
int i = inputStream.nextInt();
int j = inputStream.nextInt();
//send the output to the client
outputStream.println(i + j);
//flush the stream
outputStream.flush();
//display the statement
System.out.println("The connection is closed ");
//close the connections
inputStream.close();
outputStream.close();
}
//catch block
catch (Exception e)
{
// display the exception
System.out.println("Error " + e);
}
}
}
Client program:
//import required header files
import java...

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- using r languagearrow_forwardDescribe a business example where referential integrity avoids data problems. specifying in the description of what the problems are and how they can be avoided. thaksarrow_forwardHow do the concepts of balancing and leveling affect the process of creating a data model of a system? thanksarrow_forward
- what is the relationship between a Context Diagram and Diagram 0 in the DFD process. I need to Use an examplearrow_forwardWord Processing The assignment is a newsletter for your friends and family to let them know what’s going on in your life. Your document cannot contain profanity or obscene material—this is a business assignment. The minimum requirements for your newsletter are listed below. It should contain: 2 – 4 pages Your name A title using WordArt with one or more effects applied Articles with formatted titles using a font and color different than that of the article text A section of at least 2 columns Headers and page numbers on all pages except the first page. A bulleted or numbered list A relevant picture or clip art A formatted table Tabs with leaders going to the tabbed items At least one Sidebar Your name as the document author Boldface, italicized, and underlined text A paragraph with justified margins that is shaded and has a border A paragraph with different line spacing than the rest of the document A left, right, or both indented paragraph NOTE: If providing information from outside…arrow_forwardYou are designing a set of firewall rules for server subnet. You have a Web server that constantly gets high volume of traffic from both internal and public clients, a file server that gets moderate use during regular business hours, a VPN appliance used by sales team when they have occasional travels, and an application server for custom apps served on internal network. Describe the firewall rules you would create and the order in which you would place them. Explain why.arrow_forward
- Please original work In the progression from raw data to actionable knowledge, business analysts play a crucial role in transforming and interpreting data to support strategic decision-making. What do you think are the most important skills a business analyst needs to effectively navigate the transition from data to information and then to knowledge? How can organizations ensure that analysts are equipped to extract meaningful insights that drive informed decisions? Share examples or insights from your own experiences or studies. Please cite in text references and add weblinksarrow_forwardResearch enterprise network services commonly performed by Linux servers. Choose 3 and describe their function, as well as why they are typically set up on Linux machines.arrow_forwardThe term color tone refers to the "temperature" of a photo. Question 17Select one: True Falsearrow_forward
- You cannot add 3-dimensional effects to a shape. Question 18Select one: True Falsearrow_forwardWhich gallery shows available shapes for WordArt text? Question 10Select one: a. Transform b. Shape Styles c. Themes d. WordArt Stylesarrow_forwardWhen you press [Shift][Ctrl] while dragging a corner sizing handle on a graphic, the graphic is ____. Question 9Select one: a. resized while keeping the center position fixed and maintaining its proportions b. resized proportionally c. re-positioned diagonally d. resized diagonally while changing proportionallyarrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
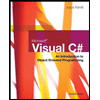
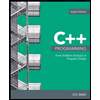
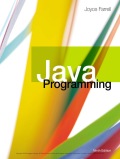
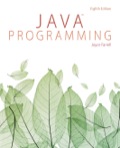