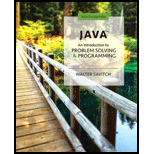
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 10, Problem 9E
Repeat the previous exercise, but write the new lines to a new binary file instead of a text file.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Read a source file name (text file) from the user (e.g, myprog.cpp) and produce a copy ofthe same. Also display the following statistics:1. Number of lines of the code?2. Total characters?
Question:
Display all the names of a given length from names.dat. This file is in binary. Each entry is in the format: name length (integer) and name (string).
There are no String reading methods for binary files. You'll have to figure out how to use the length value and readChar method to read all the names.
========================================================================
Test Case 3
Enter a length\n11(ENTER)Names of length 11\nLindi Ekins\nAdriana Bru\nLinet Greep\nRich Yakovl\nRoy Garrard\nAlex Fillon\nInge Adrain\n7 results\n
----------------------------------------------------------------------
Test Case 4
Enter a length\n12 (ENTER)Names of length 12\nSabrina Digg\nHowie Royson\nBronny Blues\nMurdoch Vase\nRyley Chmiel\nBrand Hallam\nKippy Baudet\nTate Stainer\nLindie Freke\n9 results\n
========================================================
MY code
import java.io.*;import java.util.ArrayList;import java.util.Scanner;public class TEST {public…
Suppose that you have two text files that contain sequences of integers separated by white space (blank space, tabs, and line breaks). The integers in both files appear in sorted order, with smaller values near the beginning of the file and large values closer to the end. Write a pseudocode algorithm that merges the two sequences into a single sorted sequence that is written to a third file.
Chapter 10 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 10.1 - Why would anybody write a program that sends its...Ch. 10.1 - When we discuss input, are we referring to data...Ch. 10.1 - What is the difference between a text file and a...Ch. 10.2 - Write some code that will create a stream named...Ch. 10.2 - Prob. 5STQCh. 10.2 - Prob. 6STQCh. 10.2 - Prob. 7STQCh. 10.2 - Write some code that will create a stream named...Ch. 10.2 - Suppose you run a program that writes to the text...Ch. 10.2 - Prob. 10STQ
Ch. 10.3 - Prob. 11STQCh. 10.4 - Write some Java code to create an output stream of...Ch. 10.4 - Give three statements that will write the values...Ch. 10.4 - Give a statement that will close the stream toFile...Ch. 10.4 - What import statement(s) do you use when creating...Ch. 10.4 - Prob. 16STQCh. 10.4 - Give three statements that will read three numbers...Ch. 10.4 - Give a statement that will close the stream...Ch. 10.4 - Can you use writeInt to write a number to a file...Ch. 10.4 - Can you use readUTF to read a string from a text...Ch. 10.4 - Prob. 21STQCh. 10.4 - Prob. 22STQCh. 10.4 - Does the class FileInputStream have a method named...Ch. 10.4 - Does the class FileOutputStream have a constructor...Ch. 10.4 - Does the class ObjectOutputStream have a...Ch. 10.4 - Prob. 26STQCh. 10.4 - Suppose that a binary file contains exactly three...Ch. 10.4 - The following code appears in the program in...Ch. 10.4 - Prob. 29STQCh. 10.5 - Prob. 30STQCh. 10.5 - Prob. 31STQCh. 10.5 - Prob. 32STQCh. 10.5 - Prob. 33STQCh. 10.6 - Prob. 34STQCh. 10.6 - Prob. 35STQCh. 10 - Write a program that will write the Gettysburg...Ch. 10 - Modify the program in the previous exercise so...Ch. 10 - Write some code that asks the user to enter either...Ch. 10 - Write a program that will record the purchases...Ch. 10 - Modify the class LapTimer, as described in...Ch. 10 - Write a class TelephoneNumber that will hold a...Ch. 10 - Write a class contactInfo to store contact...Ch. 10 - Write a program that reads every line in a text...Ch. 10 - Repeat the previous exercise, but write the new...Ch. 10 - Write a program that will make a copy of a text...Ch. 10 - Suppose you are given a text file that contains...Ch. 10 - Suppose that you have a binary file that contains...Ch. 10 - Suppose that we want to store digitized audio...Ch. 10 - Write a program RecoverSignal that will read the...Ch. 10 - Even though a binary file is not a text file, it...Ch. 10 - Write a program that searches a file of numbers...Ch. 10 - Write a program that reads a file of numbers of...Ch. 10 - The following is an old word puzzle: Name a common...Ch. 10 - The Social Security Administration maintains an...Ch. 10 - The following is a list of scores for a game....Ch. 10 - Write a program that checks a text file for...Ch. 10 - Prob. 5PPCh. 10 - Prob. 6PPCh. 10 - Revise the class Pet, as shown in Listing 6.1 of...Ch. 10 - Write a program that reads records of type Pet...Ch. 10 - Prob. 12PP
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Prove that the eigenvalue problem y+y=0;y(0)=0,y(1)=y(1)=0 has no negative eigenvalues. (Suggestion: Show graph...
Differential Equations: Computing and Modeling (5th Edition), Edwards, Penney & Calvis
For each of the following numeric formats, identify the format string used as the input parameter when calling ...
Starting Out With Visual Basic (8th Edition)
The following program is used in a bookstore to determine how many discount coupons a customer gets. Complete t...
Starting Out with C++: Early Objects (9th Edition)
Define each of the following terms: supertype subtype specialization entity cluster completeness constraint enh...
Modern Database Management (12th Edition)
State whether each of the following is true or false. If false, explain why. The following are all invalid vari...
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
In what year was Plankalkl designed? In what year was that design published?
Concepts of Programming Languages (11th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a program to read the file numbers.txt created in part 1 that was saved to your home directory. As you read each number, keep track of how many times each of the numbers appear in the file (use a dictionary with the number as the key and count as the value). Print the count of each number and the sum of all the numbers in the file.arrow_forwardUse Python write a program that reads a file and writes out a new file with the lines in reversed order (i.e. the first line in the old file becomes the last one in the new file.)arrow_forwardCount how often each bit is set in all the bytes of the given binary file. Open the file with the given name as a binary file. Count how often each bit is set in all the bytes of the file. A byte value returned by infile.get outside the range from 0 to 255 indicates the end of the file. Once you have a byte, you can get the bits like this: for (int i = 0; i < 8; i++) { if (byte % 2 == 1) { // The i-th bit is set } byte = byte / 2; } CODE SHOWN BELOW: #include <iostream>#include <fstream>#include <string> using namespace std; int main(){int bit_counts[8];for (int i = 0; i < 8; i++) { bit_counts[i] = 0; }fstream infile;string filename;cin >> filename; /* Your code goes here */ int largest = 0;for (int i = 0; i < 8; i++){cout << i << ": " << bit_counts[i] << endl;}return 0;}arrow_forward
- You have a huge text file with words in it. Find the smallest distance (in terms of number of words) between any two words in the file. Can you optimise your solution if the procedure will be performed several times for the same file (but different pairs of words)?arrow_forwardWrite a program to copy an existing text file from your hard disk to another file that you will call: Your Last Name.txt, e.g. if your last name was Smith, the output file name would be Smith.txt. You can create a text file and add two or three lines of text to it. You may use the attached program as your program or write your own. Please note the inclusion of <fstream> at the top of the program. Also pay attention to the open and close statements in the program. // LAB6.cpp// Kunika Saxena// File: CopyFile.cpp// Copies file InData.txt to file OutData.txt #include <cstdlib> // for the definition of EXIT_FAILURE#include <fstream> // required for external file streams#include <iostream>using namespace std; // Associate stream objects with external file names#define inFile "InData.txt"#define outFile "Saxena.txt" // Functions used ...// Copies one line of textint copyLine(ifstream&, ofstream&); int main(){ // Local data ... int…arrow_forwardCreate a flex file which recognizes if a given string is a word or a number. (Here we have 3 possibilities: word, number, other)Create a flex file which recognizes if a given string is an email address.Create a flex file which counts how many words start with a capital letter.Make calculator also run with float numbers.Create a calculator (multiplication, addition, division, extraction) for binary numbers.arrow_forward
- Use " JAVA programming" Don't provide wrong solutionarrow_forwardConsider the file inputfile.bxt which contains the following elements (numbers): 134, 325, 513, 726 Write a python program to read the numbers from the inputfile.txt, compute its reverse, and wite the result into another file, say outputfile.txt. The contents of outputfile.txt should be as follows: 431, 523, 315, 627arrow_forwardWrite a program that reads the strings from file SortedStrings. txt and report whether the strings in the files are stored in ascending order. If the strings are not sorted in the file, display the first two strings that are out of order.arrow_forward
- Write a program that reads two input files whose lines are ordered by a key data field. Your program should merge these two files, writing an output file that contains all lines from both files ordered by the same key field. As an example, if two input files contain student names and grades for a particular class ordered by name (the key field), merge the information as shown below. File 1 and file 2 are supplied. Here is an algorithm to merge the data from two files: Read a line from each data file While the end of both files has not been reached While the end of both files has not been reached Write the line from file 1 to the output file and read a new line from file 1. Else Write the line from file 2 to the output file and read a new line from file 2. Write the remaining lines (if any) from file 1 to the output file. Write the remaining lines (if any) from file 2 to the output file. See the Merging Filesslides attachedto the project in Canvasfor a visual look at this algorithm.arrow_forwardSuppose you are given a text file that contains the names of people. Every name in the file consists of a first name and last name. Unfortunately, the programmer that created the file of names did not guarantee that each name was on a single line of the file. Read this file of names and write them to a new text file sorted according to first name, one name per line. For example, if the input file contains Ed Marston Bob Jones Jeff Williams Fred Charles The output file should be Bob Jones Ed Marston Fred Charles Jeff Williams Use arrays to solve the problem.arrow_forwardWhat is the difference between a binary file and a text file? Is it possible to use a text editor to see a text or binary file?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
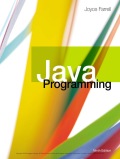
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Files & File Systems: Crash Course Computer Science #20; Author: CrashCourse;https://www.youtube.com/watch?v=KN8YgJnShPM;License: Standard YouTube License, CC-BY
UNIX Programming (Part - 10) The File System (Directories and Files Names); Author: ITUTEES;https://www.youtube.com/watch?v=K35faWBhzrw;License: Standard Youtube License