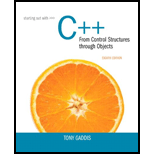
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 10.6, Problem 10.16CP
Explanation of Solution
The program is to change the every first letter “T” to “D” is as follows:
// Include the header files
#include <iostream>
using namespace std;
void mess(char[]);
// Definition of Main function
int main()
{
// Declare variable to hold the statement
char stuff[] = "Tom Talbert Tried Trains";
//Display the input statement
cout << stuff << endl;
//call the function "mess"
mess(stuff);
//Display the output statement
cout << stuff << endl;
//return statement
return 0;
}
//Definition of calling function
void mess(char str[])
{
// Declare variable to hold the value
int step = 0...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Classwork Requirements:
Developa program in C++ that:
Reads as many test scores as the user wants from the keyboard (assuming at most 50scores).
Test scores should be whole numbers.
You must validate user input; only positive numbers are valid.
Prints the scores in
original order
sorted from high to low
the highest score
the lowest score
the average of the scores
Implement the following functions using the given function prototypes:
void displayArray(int array[], int size)- Displays the content of the array
void selectionSort(int array[], int size)- sorts the array using the selection sort algorithm in descending Hint: refer to example 8-5 in the textbook.
int findMax(int array[], int size)- finds and returns the highest element of the array
int findMin(int array[], int size)- finds and returns the lowest element of the array
double findAvg(int array[], int size)- finds and returns the average of the elements of the array
#include<stdio.h>
//Standard library math.h for Mathematics functions
#include<math.h>
//function declaration
void function1(void);
void function2(void);
int main(void)
{
//calling both function
function1();
function2();
printf("\nMain function for Ceil value: %f",ceil(4.5));
return0;
}
// Defining the first function
void function1(void)
{
printf("\nFirst function for Square Root: %f",sqrt(91));
}
//Defining the second function
void function2(void)
{
printf("\nSecond function for Power: %f",pow(2,4));
}
I need to do what the picture is asking for.
#include
using namespace std;
void myfunction(int num2, int num1);
lint main() {
my function (5,2);
return 0; }
void myfunction(int num1, int num2)
{if (num1>3)
cout << "A1";
else if (num1<3)
cout<<"A2";
else
cout<<"A3";}
O A2
O A1
O A3
A1 A2 A3
Chapter 10 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 10.2 - Write a short description of each of the following...Ch. 10.2 - Prob. 10.2CPCh. 10.2 - Write an if statement that will display the word...Ch. 10.2 - What is the output of the following statement?...Ch. 10.2 - Write a loop that asks the user Do you want to...Ch. 10.4 - Write a short description of each of the following...Ch. 10.4 - Prob. 10.7CPCh. 10.4 - Prob. 10.8CPCh. 10.4 - Prob. 10.9CPCh. 10.4 - When complete, the following program skeleton will...
Ch. 10.5 - Write a short description of each of the following...Ch. 10.5 - Write a statement that will convert the string 10...Ch. 10.5 - Prob. 10.13CPCh. 10.5 - Write a statement that will convert the string...Ch. 10.5 - Prob. 10.15CPCh. 10.6 - Prob. 10.16CPCh. 10 - Prob. 1RQECh. 10 - Prob. 2RQECh. 10 - Prob. 3RQECh. 10 - Prob. 4RQECh. 10 - Prob. 5RQECh. 10 - Prob. 6RQECh. 10 - Prob. 7RQECh. 10 - Prob. 8RQECh. 10 - Prob. 9RQECh. 10 - Prob. 10RQECh. 10 - The __________ function returns true if the...Ch. 10 - Prob. 12RQECh. 10 - Prob. 13RQECh. 10 - The __________ function returns the lowercase...Ch. 10 - The _________ file must be included in a program...Ch. 10 - Prob. 16RQECh. 10 - Prob. 17RQECh. 10 - Prob. 18RQECh. 10 - Prob. 19RQECh. 10 - Prob. 20RQECh. 10 - Prob. 21RQECh. 10 - Prob. 22RQECh. 10 - Prob. 23RQECh. 10 - Prob. 24RQECh. 10 - The ________ function returns the value of a...Ch. 10 - Prob. 26RQECh. 10 - The following if statement determines whether...Ch. 10 - Assume input is a char array holding a C-string....Ch. 10 - Look at the following array definition: char...Ch. 10 - Prob. 30RQECh. 10 - Write a function that accepts a pointer to a...Ch. 10 - Prob. 32RQECh. 10 - Prob. 33RQECh. 10 - T F If touppers argument is already uppercase, it...Ch. 10 - T F If tolowers argument is already lowercase, it...Ch. 10 - T F The strlen function returns the size of the...Ch. 10 - Prob. 37RQECh. 10 - T F C-string-handling functions accept as...Ch. 10 - T F The strcat function checks to make sure the...Ch. 10 - Prob. 40RQECh. 10 - T F The strcpy function performs no bounds...Ch. 10 - T F There is no difference between 847 and 847.Ch. 10 - Prob. 43RQECh. 10 - char numeric[5]; int x = 123; numeri c = atoi(x);Ch. 10 - char string1[] = "Billy"; char string2[] = " Bob...Ch. 10 - Prob. 46RQECh. 10 - Prob. 1PCCh. 10 - Prob. 2PCCh. 10 - Prob. 3PCCh. 10 - Average Number of Letters Modify the program you...Ch. 10 - Prob. 5PCCh. 10 - Prob. 6PCCh. 10 - Name Arranger Write a program that asks for the...Ch. 10 - Prob. 8PCCh. 10 - Prob. 9PCCh. 10 - Prob. 10PCCh. 10 - Prob. 11PCCh. 10 - Password Verifier Imagine you are developing a...Ch. 10 - Prob. 13PCCh. 10 - Word Separator Write a program that accepts as...Ch. 10 - Character Analysis If you have downloaded this...Ch. 10 - Prob. 16PCCh. 10 - Prob. 18PCCh. 10 - Check Writer Write a program that displays a...
Knowledge Booster
Similar questions
- C PROGRAM Reverse + Random Formula In the code editor, there's already an initial code that contains the function declaration of the function, computeReverseNumber(int n) and an empty main() function. For this problem, you are task to implement the computeReverseNumber function. This function will take in an integer n as its parameter. This will get the reverse of the passed integer and will then compute and return the value: result = (original_number + reverse_of_the_number) / 3 In the main() function, ask the user to input the value of n, call the function computeReverseNumber and pass the inputted value of n, and print the result in the main() with two (2) decimal places. SAMPLE: Input n: 20 Output: 7.33 Input n: 123 Output: 148.00arrow_forwardExercise 5 #include using namespace std/ int squareByValue ( int ); // function prototypes void squareByReference ( int * ); // function prototype with pointer as argument int main () int x = 2, z = 4; cout << "x = " << x << " before squareByValue\n" << "Value returned by squareByValue: < squareByValue ( x ) << endl << "x = " << x << after squareByValue\n" << endl; cout << "z = " << z << " before squareByReference" <arrow_forward2.A. Is it possible to pass variable y as parameter to the functions? 2.B. Supply the missing code stated in TODO at line 17-19. 2.C Supply the missing code stated in TODO at line 21-22. 2.D. (Assuming the code is complete) What will be the display of this program?arrow_forwardC programming / Finding areaarrow_forwardUsing C++ Programming language: Assume you want a function which expects as parameters an array of doubles and the size of the array. Write the function header that accepts these parameters but is defined in such a way that the array cannot be modified in the function. You can use your own variable names for the parameters.arrow_forwardCode Should Be In C++arrow_forward/*Rewrite the given program using function overloading*/ /* Rewrite the given program using the concept of function overloading.*/#include<iostream>using namespace std;int findProductInt(int a, int b){ return (a*b);}double findProductDouble(double a, double b){ return (a*b);}int findProductThreeInt(int a, int b, int c){ return (a*b*c);}double findProductThreeDouble(double a, double b, double c){ return (a*b*c);}int main(){ int a,b,c; double x,y,z; cout<<"Enter three integers"<<endl; cin>>a>>b>>c; cout<<"Enter three double"<<endl; cin>>x>>y>>z; cout<<"Product of two integers a and b ="<<findProductInt(a,b)<<endl; cout<<"Product of three integers a,b and c ="<<findProductThreeInt(a,b,c)<<endl; cout<<"Product of two double x and y ="<<findProductDouble(x,y)<<endl; cout<<"Product of three double x,y and z…arrow_forwardC++arrow_forwardWhat will the following program display? #include <iostream> using namespace std;// Function prototype void showMe(int arg);int main() { int num = 0; showMe(num);return 0; } void showMe(int arg) { if (arg < 10) showMe(++arg); elsecout << arg << end1; }arrow_forwardNonearrow_forward3A in Python language please:arrow_forwardThe following program skeleton asks for the number of hours you’ve worked and your hourly pay rate. It then calculates and displays your wages. The function showDollars, which you are to write, formats the output of the wages. #include <iostream> #include <iomanip> using namespace std;void showDollars(double pay); // Function prototype int main(){ double payRate, hoursWorked, wages;cout << "How many hours have you worked? " cin >> hoursWorked;cout << "What is your hourly pay rate? "; cin >> payRate;wages = hoursWorked * payRate; showDollars(wages);return 0; }// Write the definition of the showDollars function here.// It should have one double parameter and display the message // "Your wages are $" followed by the value of the parameter.arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
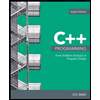
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning