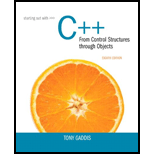
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 10, Problem 11PC
Program Plan Intro
Case Manipulator
- Include the required header files to the program.
- Declare the constant variable.
- Declare function prototypes which are used in the program.
- Define the “main()” function.
- Declare the required variables.
- Get the input C-string from the user.
- Copy the string 1 to string 2 and string 3.
- Call the “upper” function and display the result.
- Call the “lower” function and display the result.
- Call the “reserve” function and display the result.
- Define the “upper” function.
- Check the string not equal to zero.
- If the letter is not an uppercase, change the letters into uppercase using “toupper” function.
- Check the string not equal to zero.
- Define the “lower” function.
- Check the string not equal to zero.
- If the letter is not a lowercase, change the letters into lowercase using “tolower” function.
- Check the string not equal to zero.
- Define the “reverse” function.
- Check the string not equal to zero.
- If the letter is not an uppercase, change the letters into uppercase using “toupper” function.
- Otherwise, if the letter is not a lowercase, change the letters into lowercase using “tolower” function.
- Check the string not equal to zero.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
3. Problem Description: Define the Circle2D class that contains:
Two double data fields named x and y that specify the center of the circle with get
methods.
• A data field radius with a get method.
•
A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius.
•
A constructor that creates a circle with the specified x, y, and radius.
•
A method getArea() that returns the area of the circle.
•
A method getPerimeter() that returns the perimeter of the circle.
•
•
•
A method contains(double x, double y) that returns true if the specified point (x, y) is inside
this circle. See Figure (a).
A method contains(Circle2D circle) that returns true if the specified circle is inside this
circle. See Figure (b).
A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this
circle. See the figure below.
р
O
со
(a)
(b)
(c)<
Figure (a) A point is inside the circle. (b) A circle is inside another circle.
(c) A circle overlaps another…
1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.
Security in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.
Chapter 10 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 10.2 - Write a short description of each of the following...Ch. 10.2 - Prob. 10.2CPCh. 10.2 - Write an if statement that will display the word...Ch. 10.2 - What is the output of the following statement?...Ch. 10.2 - Write a loop that asks the user Do you want to...Ch. 10.4 - Write a short description of each of the following...Ch. 10.4 - Prob. 10.7CPCh. 10.4 - Prob. 10.8CPCh. 10.4 - Prob. 10.9CPCh. 10.4 - When complete, the following program skeleton will...
Ch. 10.5 - Write a short description of each of the following...Ch. 10.5 - Write a statement that will convert the string 10...Ch. 10.5 - Prob. 10.13CPCh. 10.5 - Write a statement that will convert the string...Ch. 10.5 - Prob. 10.15CPCh. 10.6 - Prob. 10.16CPCh. 10 - Prob. 1RQECh. 10 - Prob. 2RQECh. 10 - Prob. 3RQECh. 10 - Prob. 4RQECh. 10 - Prob. 5RQECh. 10 - Prob. 6RQECh. 10 - Prob. 7RQECh. 10 - Prob. 8RQECh. 10 - Prob. 9RQECh. 10 - Prob. 10RQECh. 10 - The __________ function returns true if the...Ch. 10 - Prob. 12RQECh. 10 - Prob. 13RQECh. 10 - The __________ function returns the lowercase...Ch. 10 - The _________ file must be included in a program...Ch. 10 - Prob. 16RQECh. 10 - Prob. 17RQECh. 10 - Prob. 18RQECh. 10 - Prob. 19RQECh. 10 - Prob. 20RQECh. 10 - Prob. 21RQECh. 10 - Prob. 22RQECh. 10 - Prob. 23RQECh. 10 - Prob. 24RQECh. 10 - The ________ function returns the value of a...Ch. 10 - Prob. 26RQECh. 10 - The following if statement determines whether...Ch. 10 - Assume input is a char array holding a C-string....Ch. 10 - Look at the following array definition: char...Ch. 10 - Prob. 30RQECh. 10 - Write a function that accepts a pointer to a...Ch. 10 - Prob. 32RQECh. 10 - Prob. 33RQECh. 10 - T F If touppers argument is already uppercase, it...Ch. 10 - T F If tolowers argument is already lowercase, it...Ch. 10 - T F The strlen function returns the size of the...Ch. 10 - Prob. 37RQECh. 10 - T F C-string-handling functions accept as...Ch. 10 - T F The strcat function checks to make sure the...Ch. 10 - Prob. 40RQECh. 10 - T F The strcpy function performs no bounds...Ch. 10 - T F There is no difference between 847 and 847.Ch. 10 - Prob. 43RQECh. 10 - char numeric[5]; int x = 123; numeri c = atoi(x);Ch. 10 - char string1[] = "Billy"; char string2[] = " Bob...Ch. 10 - Prob. 46RQECh. 10 - Prob. 1PCCh. 10 - Prob. 2PCCh. 10 - Prob. 3PCCh. 10 - Average Number of Letters Modify the program you...Ch. 10 - Prob. 5PCCh. 10 - Prob. 6PCCh. 10 - Name Arranger Write a program that asks for the...Ch. 10 - Prob. 8PCCh. 10 - Prob. 9PCCh. 10 - Prob. 10PCCh. 10 - Prob. 11PCCh. 10 - Password Verifier Imagine you are developing a...Ch. 10 - Prob. 13PCCh. 10 - Word Separator Write a program that accepts as...Ch. 10 - Character Analysis If you have downloaded this...Ch. 10 - Prob. 16PCCh. 10 - Prob. 18PCCh. 10 - Check Writer Write a program that displays a...
Knowledge Booster
Similar questions
- Please include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forwardObject-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forward
- When using functions in python, it allows us tto create procedural abstractioons in our programs. What are 5 major benefits of using a procedural abstraction in python?arrow_forwardFind the error, assume data is a string and all variables have been declared. for ch in data: if ch.isupper: num_upper = num_upper + 1 if ch.islower: num_lower = num_lower + 1 if ch.isdigit: num_digits = num_digits + 1 if ch.isspace: num_space = num_space + 1arrow_forwardFind the Error: date_string = input('Enter a date in the format mm/dd/yyyy: ') date_list = date_string.split('-') month_num = int(date_list[0]) day = date_list[1] year = date_list[2] month_name = month_list[month_num - 1] long_date = month_name + ' ' + day + ', ' + year print(long_date)arrow_forward
- Find the Error: full_name = input ('Enter your full name: ') name = split(full_name) for string in name: print(string[0].upper(), sep='', end='') print('.', sep=' ', end='')arrow_forwardPlease show the code for the Tikz figure of the complex plane and the curve C. Also, mark all singularities of the integrand.arrow_forward11. Go to the Webinars worksheet. DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: a. Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. b. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. c. Determine and enter the constraints based on the information provided in Table 3. d. Use Simplex LP as the solving method to find a global optimal solution. e. Save the Solver model below the Maximum weekly profit model label. f. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and…arrow_forward
- Go to the Webinars DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. Determine and enter the constraints based on the information provided in Table 3. Use Simplex LP as the solving method to find a global optimal solution. Save the Solver model below the Maximum weekly profit model label. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and Thursday…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)? When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene. Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency. To elaborate,…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
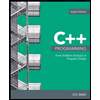
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
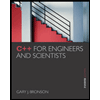
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
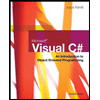
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
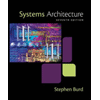
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
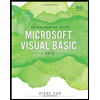
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning