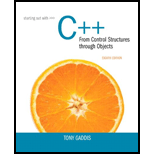
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 10, Problem 8PC
Program Plan Intro
Sum of Digits in a String
- Include the required header files to the program.
- Declare function prototype which is used in the program.
- Define the “main()” function.
- Declare the required variables.
- Get the input C-string from the user and call the function “digit” with the input C-string.
- The “for” loop is used to convert the C-string to digit
- Call the function “digit” with an argument and store it in a variable.
- Sum all that values and store in a separate variable.
- Found the highest and lowest value of the C-string using “if” condition.
- Print the result.
- Define the “digit” function.
- Declare the required variable.
- Convert the character string to integer.
- Define an array to hold an empty string value.
- Convert that string to integer using “atoi” function.
- If the input is not a digit, return 0.
- Finally return the result to the main function.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Of the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization?
Part A - Define each primary component of the information system.
Part B - Include your perspective on why your selection is most important.
Part C - Provide an example from your personal experience to support your answer.
Management Information Systems
Q2/find the transfer function C/R for the system shown in the figure
Re
ད
Chapter 10 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 10.2 - Write a short description of each of the following...Ch. 10.2 - Prob. 10.2CPCh. 10.2 - Write an if statement that will display the word...Ch. 10.2 - What is the output of the following statement?...Ch. 10.2 - Write a loop that asks the user Do you want to...Ch. 10.4 - Write a short description of each of the following...Ch. 10.4 - Prob. 10.7CPCh. 10.4 - Prob. 10.8CPCh. 10.4 - Prob. 10.9CPCh. 10.4 - When complete, the following program skeleton will...
Ch. 10.5 - Write a short description of each of the following...Ch. 10.5 - Write a statement that will convert the string 10...Ch. 10.5 - Prob. 10.13CPCh. 10.5 - Write a statement that will convert the string...Ch. 10.5 - Prob. 10.15CPCh. 10.6 - Prob. 10.16CPCh. 10 - Prob. 1RQECh. 10 - Prob. 2RQECh. 10 - Prob. 3RQECh. 10 - Prob. 4RQECh. 10 - Prob. 5RQECh. 10 - Prob. 6RQECh. 10 - Prob. 7RQECh. 10 - Prob. 8RQECh. 10 - Prob. 9RQECh. 10 - Prob. 10RQECh. 10 - The __________ function returns true if the...Ch. 10 - Prob. 12RQECh. 10 - Prob. 13RQECh. 10 - The __________ function returns the lowercase...Ch. 10 - The _________ file must be included in a program...Ch. 10 - Prob. 16RQECh. 10 - Prob. 17RQECh. 10 - Prob. 18RQECh. 10 - Prob. 19RQECh. 10 - Prob. 20RQECh. 10 - Prob. 21RQECh. 10 - Prob. 22RQECh. 10 - Prob. 23RQECh. 10 - Prob. 24RQECh. 10 - The ________ function returns the value of a...Ch. 10 - Prob. 26RQECh. 10 - The following if statement determines whether...Ch. 10 - Assume input is a char array holding a C-string....Ch. 10 - Look at the following array definition: char...Ch. 10 - Prob. 30RQECh. 10 - Write a function that accepts a pointer to a...Ch. 10 - Prob. 32RQECh. 10 - Prob. 33RQECh. 10 - T F If touppers argument is already uppercase, it...Ch. 10 - T F If tolowers argument is already lowercase, it...Ch. 10 - T F The strlen function returns the size of the...Ch. 10 - Prob. 37RQECh. 10 - T F C-string-handling functions accept as...Ch. 10 - T F The strcat function checks to make sure the...Ch. 10 - Prob. 40RQECh. 10 - T F The strcpy function performs no bounds...Ch. 10 - T F There is no difference between 847 and 847.Ch. 10 - Prob. 43RQECh. 10 - char numeric[5]; int x = 123; numeri c = atoi(x);Ch. 10 - char string1[] = "Billy"; char string2[] = " Bob...Ch. 10 - Prob. 46RQECh. 10 - Prob. 1PCCh. 10 - Prob. 2PCCh. 10 - Prob. 3PCCh. 10 - Average Number of Letters Modify the program you...Ch. 10 - Prob. 5PCCh. 10 - Prob. 6PCCh. 10 - Name Arranger Write a program that asks for the...Ch. 10 - Prob. 8PCCh. 10 - Prob. 9PCCh. 10 - Prob. 10PCCh. 10 - Prob. 11PCCh. 10 - Password Verifier Imagine you are developing a...Ch. 10 - Prob. 13PCCh. 10 - Word Separator Write a program that accepts as...Ch. 10 - Character Analysis If you have downloaded this...Ch. 10 - Prob. 16PCCh. 10 - Prob. 18PCCh. 10 - Check Writer Write a program that displays a...
Knowledge Booster
Similar questions
- Please original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forwardPlease original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forward
- What are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forwardDetermine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward
- • Solve the problem (pls refer to the inserted image)arrow_forwardWrite .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forwardWrite .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward
- • Solve the problem (pls refer to the inserted image) and create line graph.arrow_forwardwho started the world wide webarrow_forwardQuestion No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
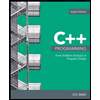
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
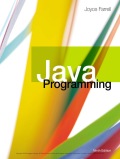
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT