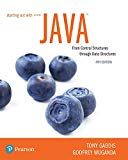
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 10, Problem 9AW
Look at the following interface:
public interface Computable
{
double compute(double x);
}
Write a statement that uses a lambda expression to create an object that implements the Computable interface. The object’s name should he half. The half object’s compute method should return the value of the x parameter divided by 2.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Question 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.
Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.
Can I get help with this case please, thank you
Chapter 10 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 10.1 - Here is the first line of a class declaration....Ch. 10.1 - Look at the following class declarations and...Ch. 10.1 - Class B extends class A. (Class A is the...Ch. 10.2 - Prob. 10.4CPCh. 10.2 - Look at the following classes: public class Ground...Ch. 10.3 - Under what circumstances would a subclass need to...Ch. 10.3 - How can a subclass method call an overridden...Ch. 10.3 - If a method in a subclass has the same signature...Ch. 10.3 - If a method in a subclass has the same name as a...Ch. 10.3 - Prob. 10.10CP
Ch. 10.4 - When a class member is declared as protected, what...Ch. 10.4 - What is the difference between private members and...Ch. 10.4 - Why should you avoid making class members...Ch. 10.4 - Prob. 10.14CPCh. 10.4 - Why is it easy to give package access to a class...Ch. 10.6 - Look at the following class definition: public...Ch. 10.6 - When you create a class, it automatically has a...Ch. 10.7 - Recall the Rectangle and Cube classes discussed...Ch. 10.8 - Prob. 10.19CPCh. 10.8 - If a subclass extends a superclass with an...Ch. 10.8 - What is the purpose of an abstract class?Ch. 10.8 - If a class is defined as abstract, what can you...Ch. 10.9 - Prob. 10.23CPCh. 10.9 - Prob. 10.24CPCh. 10.9 - Prob. 10.25CPCh. 10.9 - Prob. 10.26CPCh. 10.9 - Prob. 10.27CPCh. 10.9 - Prob. 10.28CPCh. 10 - In an inheritance relationship, this is the...Ch. 10 - In an inheritance relationship, this is the...Ch. 10 - This key word indicates that a class inherits from...Ch. 10 - A subclass does not have access to these...Ch. 10 - This key word refers to an objects superclass. a....Ch. 10 - In a subclass constructor, a call to the...Ch. 10 - The following is an explicit call to the...Ch. 10 - A method in a subclass that has the same signature...Ch. 10 - A method in a subclass having the same name as a...Ch. 10 - These superclass members are accessible to...Ch. 10 - Prob. 11MCCh. 10 - With this type of binding, the Java Virtual...Ch. 10 - This operator can be used to determine whether a...Ch. 10 - When a class implements an interface, it must...Ch. 10 - Prob. 15MCCh. 10 - Prob. 16MCCh. 10 - Abstract classes cannot ___________. a. be used as...Ch. 10 - You use the __________ operator to define an...Ch. 10 - Prob. 19MCCh. 10 - Prob. 20MCCh. 10 - You can use a lambda expression to instantiate an...Ch. 10 - True or False: Constructors are not inherited.Ch. 10 - True or False: in a subclass, a call to the...Ch. 10 - True or False: If a subclass constructor does not...Ch. 10 - True or False: An object of a superclass can...Ch. 10 - True or False: The superclass constructor always...Ch. 10 - True or False: When a method is declared with the...Ch. 10 - True or False: A superclass has a member with...Ch. 10 - True or False: A superclass reference variable can...Ch. 10 - True or False: A subclass reference variable can...Ch. 10 - True or False: When a class contains an abstract...Ch. 10 - True or False: A class may only implement one...Ch. 10 - True or False: By default all members of an...Ch. 10 - // Superclass public class Vehicle { (Member...Ch. 10 - // Superclass public class Vehicle { private...Ch. 10 - // Superclass public class Vehicle { private...Ch. 10 - // Superclass public class Vehicle { public...Ch. 10 - Write the first line of the definition for a...Ch. 10 - Look at the following code, which is the first...Ch. 10 - Write the declaration for class B. The classs...Ch. 10 - Write the statement that calls a superclass...Ch. 10 - A superclass has the following method: public void...Ch. 10 - A superclass has the following abstract method:...Ch. 10 - Prob. 7AWCh. 10 - Prob. 8AWCh. 10 - Look at the following interface: public interface...Ch. 10 - Prob. 1SACh. 10 - A program uses two classes: Animal and Dog. Which...Ch. 10 - What is the superclass and what is the subclass in...Ch. 10 - What is the difference between a protected class...Ch. 10 - Can a subclass ever directly access the private...Ch. 10 - Which constructor is called first, that of the...Ch. 10 - What is the difference between overriding a...Ch. 10 - Prob. 8SACh. 10 - Prob. 9SACh. 10 - Prob. 10SACh. 10 - What is an. abstract class?Ch. 10 - Prob. 12SACh. 10 - When you instantiate an anonymous inner class, the...Ch. 10 - Prob. 14SACh. 10 - Prob. 15SACh. 10 - Employee and ProductionWorker Classes Design a...Ch. 10 - ShiftSupervisor Class In a particular factory, a...Ch. 10 - TeamLeader Class In a particular factory, a team...Ch. 10 - Essay Class Design an Essay class that extends the...Ch. 10 - Course Grades In a course, a teacher gives the...Ch. 10 - Analyzable Interface Modify the CourseGrades class...Ch. 10 - Person and Customer Classes Design a class named...Ch. 10 - PreferredCustomer Class A retail store has a...Ch. 10 - BankAccount and SavingsAccount Classes Design an...Ch. 10 - Ship, CruiseShip, and CargoShip Classes Design a...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Specify the slope at A and the maximum deflection. Use the method of integration. El is constant.
Mechanics of Materials (10th Edition)
Distinguish among data definition commands, data manipulation commands, and data control commands.
Modern Database Management
What are the attractive features or benefits of an inverter-based power supply?
Degarmo's Materials And Processes In Manufacturing
_____ are characters or symbols that perform operations on one or more operands.
Starting Out With Visual Basic (8th Edition)
What is an is a relationship?
Starting Out with C++ from Control Structures to Objects (9th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- I need help to solve the following, thank youarrow_forwardreminder it an exercice not a grading work GETTING STARTED Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website. Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”. If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically. With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet. If cell B6 does not display your name, delete the file and download a new copy from the SAM website. Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…arrow_forwardNeed help completing this algorithm here in coding! 2arrow_forward
- In the diagram, there is a green arrow pointing from Input C (complete data) to Transformer Encoder S_B, which I don’t understand. The teacher model is trained on full data, but S_B should instead receive missing data—this arrow should not point there. Please verify and recreate the diagram to fix this issue. Additionally, the newly created diagram should meet the same clarity standards as the second diagram (Proposed MSCATN). Finally provide the output image of the diagram in image format .arrow_forwardPlease provide me with the output image of both of them . below are the diagrams code make sure to update the code and mentionned clearly each section also the digram should be clearly describe like in the attached image. please do not provide the same answer like in other question . I repost this question because it does not satisfy the requirment I need in terms of clarifty the output of both code are not very well details I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder…arrow_forwardWhy I need ?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
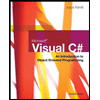
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
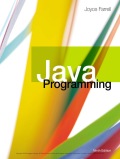
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
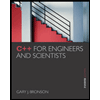
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
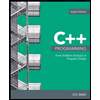
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
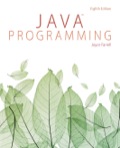
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY