Your class Hangman has the following attributes: O A String array words to store words of the game, as follows: O O // Add any words you wish in this array String words = {"program", "java", "csc111", ...}; A char[] array hiddenWord to store the current word you want the user to guess A char[] array guessedWord to store the user guesses. guessedWord is always filled up with * at the beginning and then its content (characters) change as user guesses them. O A public Scanner object input to read the user's input. Your class has the following methods: O A helper (private) method indexOf that receives a character c then it searches for c in hidden Word. If c is found then its index į is returned, otherwise method returns -1. O A helper (private) method setCharAt that takes an index i, a character c and a char array arr as parameters. The method then stores the character c in array arr at specified index i. O A helper (private) method pickWord that generates a random index and then picks and returns a word from the array words to start a new round of the game. Use method nextInt(int bound) from class java.util.Random to generate a random index less than the length of the array words. The method returns a random int value between 0 (inclusive) and the specified value bound (exclusive). O A helper (private) method copyString ToArray that: a. Receives a String s b. Returns a new char array using the String method s.toCharArray(). O A helper (private) method printWord that is used to print the array guessedWord. O A helper (private) method is Complete that checks if the guessedWord still have * in it or not. If there are no* then it returns true (indicating that all letters have been guessed) otherwise it returns false.
Please, I want to complete the requirements in this code according to the question
import java.util.Random;
import java.util.Scanner;
public class Hangman {
static String generateAsteriskString(int n) {
String s = "";
for (int i = 0; i < n; i++) {
s += "*";
}
return s;
} static String replace(String secret, String hidden, char c) {
String s = "";
for (int i = 0; i < secret.length(); i++) {
if (secret.charAt(i) == c) {
s += c;
} else {
s += hidden.charAt(i);
} }
return s;}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String[] words = { "write", "that", "program", "java", "friend", "cool" };
Random random = new Random();
int MAX_ATTEMPTS = 8;
String ch = "y";
while (ch.equalsIgnoreCase("y")) {
String secretword = words[random.nextInt(words.length)];
int misses = 0;
String hidden = generateAsteriskString(secretword.length());
while (!hidden.equals(secretword) && misses < MAX_ATTEMPTS) {
System.out.print("(Guess) Enter a letter in word " + hidden + " > ");
char letter = scanner.next().toLowerCase().charAt(0);
if (hidden.contains("" + letter)) {
System.out.println("\t" + letter + " is already in the word");
}
else if (!secretword.contains("" + letter)) {
System.out.println("\t" + letter + " is not in the word");
misses++;
} else {
hidden = replace(secretword, hidden, letter);
}
} if (misses == MAX_ATTEMPTS) {
System.out.println("Sorry! You could not guess the word. You missed "+ MAX_ATTEMPTS + " attempts, the word was "+ secretword);
} else if (misses == 1) {
System.out.println("The word is " + secretword + ". You missed " + misses + " time");
} else {
System.out.println("The word is " + secretword + ". You missed " + misses + " times");
}
System.out.print("Do you want to guess another word? Enter y or n> ");
ch = scanner.next();
}
}
}
![Your class Hangman has the following attributes:
A String[] array words to store words of the game, as follows:
O
O
// Add any words you wish in this array
String] words = {"program", "java", "csc111", ...};
A char[] array hiddenWord to store the current word you want the user to guess
A char[] array guessedWord to store the user guesses. guessedWord is always filled up with* at the
beginning and then its content (characters) change as user guesses them.
O A public Scanner object input to read the user's input.
Your class has the following methods:
O A helper (private) method indexOf that receives a character c then it searches for c in hiddenWord.
If c is found then its index į is returned, otherwise method returns -1.
O
A helper (private) method setCharAt that takes an index i, a character c and a char array arr as
parameters. The method then stores the character c in array arrat specified index i.
O
A helper (private) method pickWord that generates a random index and then picks and returns a
word from the array words to start a new round of the game. Use method nextInt(int bound) from
class java.util.Random to generate a random index less than the length of the array words. The method
returns a random int value between 0 (inclusive) and the specified value bound (exclusive).
O
A helper (private) method copyString ToArray that:
a. Receives a String s
b.
Returns a new char array using the String method
s.to.CharArray().
O
A helper (private) method printWord that is used to print the array
guessedword.
O
A helper (private) method isComplete that checks if the guessedWord still have * in it or not. If
there are no* then it returns true (indicating that all letters have been guessed) otherwise it returns
false.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F83065055-7170-4985-b044-e96855d4403d%2Fb682960e-f233-4dc6-95cb-f9ce241b8bf8%2Feg3ge4_processed.jpeg&w=3840&q=75)
![O A helper (private) method playOneRound, that let the user plays one round of the game (does not
receive or return any values). Here is how this method (i.e. core of your program) works:
C. It starts by picking a random word from the array words.
d. Then it initializes the array hiddenWord with the picked up, stringusing
copy StringToArray
e. After that it initializes each character in array guessedWord to a *.
f. Then it starts a loop. In this loop:
i.
It checks if all letters are guessed correctly using method is complete. If so then it
prints the word, using printWord, prints number of misses and terminates.
Otherwise, loop continues.
ii.
It asks the user to enter a character.
iii.
If the character is not found then it increments number of misses.
iv. Otherwise, it starts a nested loop in which:
1. It uses indexOf to check if the character is in the
hiddenWord.
2. If it is found, then it uses method setCharAt to change the corresponding
character in array guessedWord from * to the character itself.
3. Then it uses same method to change character in
hiddenWord to $ (so that we do not find it again).
4. Then it repeats this nested loop again.
V.
Then it repeats the whole loop again.
O A public method play, where the whole game goes on. This method does not receive or return any
values. It only asks the user if he wants to play a new round of the game. If the user wants to do so then
it calls playOneRound otherwise it exits.
o
A public method getWords that returns the array words.
}
O A public method getHiddenWord that returns the array hiddenWord. The code is provided
for the last two methods:
public String[] getWords()) {
return words;
public char[] getHidden Word() {
return hidden Word;
}
Draw the UML diagram for class Hangman then implementthe class.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F83065055-7170-4985-b044-e96855d4403d%2Fb682960e-f233-4dc6-95cb-f9ce241b8bf8%2F2blsdm_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

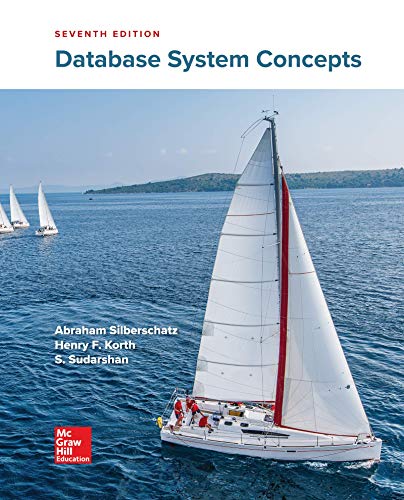
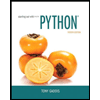
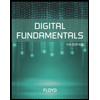
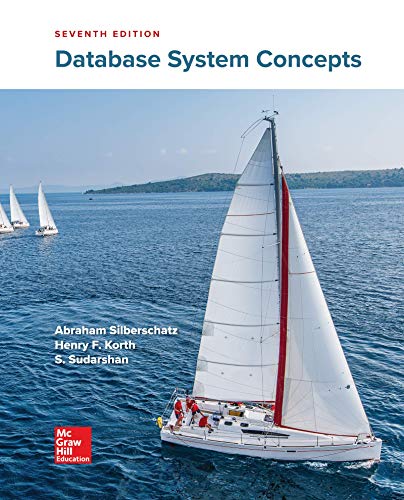
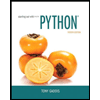
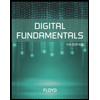
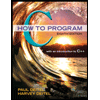
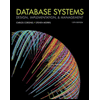
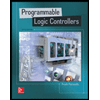