Please explain Common String Mistake in the code below/question in Bold: public class StringMistakeDemo { public static void main(String[] args) { String a = "Roux"; String b = "Roux"; String c = new String("Roux"); /* * Number 1 * Consider the output from the this block of code. * Which line causes the "false"? * Why are the last two lines different? */ System.out.println(); System.out.println("Roux" == "Roux"); //comparing two strings System.out.println(a == b); //comparing two string variables System.out.println(a == c); //comparing two other string variables System.out.println(a.equals(c));//using the string class method "equals" /* * Number 2 * "identityHashCode" provides a numeric signature that includes the * memory location for the variable. * What does the following output tell you about how Java stores strings? */ System.out.println(); System.out.println(Integer.toHexString(System.identityHashCode(a))); System.out.println(Integer.toHexString(System.identityHashCode(b))); System.out.println(Integer.toHexString(System.identityHashCode(c))); /* * Number 3 * What happens in memory when you modify the string? */ System.out.println(); a = a + b; System.out.println(a); System.out.println(Integer.toHexString(System.identityHashCode(a))); System.out.println(Integer.toHexString(System.identityHashCode(b))); System.out.println(Integer.toHexString(System.identityHashCode(c))); /* * Number 4 * When would you use the equality operator "==" and .equals? */ } }
Please explain Common String Mistake in the code below/question in Bold:
public class StringMistakeDemo
{
public static void main(String[] args) {
String a = "Roux";
String b = "Roux";
String c = new String("Roux");
/*
* Number 1
* Consider the output from the this block of code.
* Which line causes the "false"?
* Why are the last two lines different?
*/
System.out.println();
System.out.println("Roux" == "Roux"); //comparing two strings
System.out.println(a == b); //comparing two string variables
System.out.println(a == c); //comparing two other string variables
System.out.println(a.equals(c));//using the string class method "equals"
/*
* Number 2
* "identityHashCode" provides a numeric signature that includes the
* memory location for the variable.
* What does the following output tell you about how Java stores strings?
*/
System.out.println();
System.out.println(Integer.toHexString(System.identityHashCode(a)));
System.out.println(Integer.toHexString(System.identityHashCode(b)));
System.out.println(Integer.toHexString(System.identityHashCode(c)));
/*
* Number 3
* What happens in memory when you modify the string?
*/
System.out.println();
a = a + b;
System.out.println(a);
System.out.println(Integer.toHexString(System.identityHashCode(a)));
System.out.println(Integer.toHexString(System.identityHashCode(b)));
System.out.println(Integer.toHexString(System.identityHashCode(c)));
/*
* Number 4
* When would you use the equality operator "==" and .equals?
*/
}
}

String a = "Roux";
String b = "Roux";
String c = new String("Roux");
a and b variables both point to the same string constant kept in the constant string memory pool of heap data.
As c is created using new variable so c points to a completely different and new object that holds the string.
So a and b point to the same object inn heap and since == checks for reference equality so a==b gives true
"Roux" == "Roux" gives true for same reason.
a==c gives false as they are both pointing to different objects.
a.equals(c) gives true as .equals() method is overridden for String class in java and thus it compares string literals regardless of their data location.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

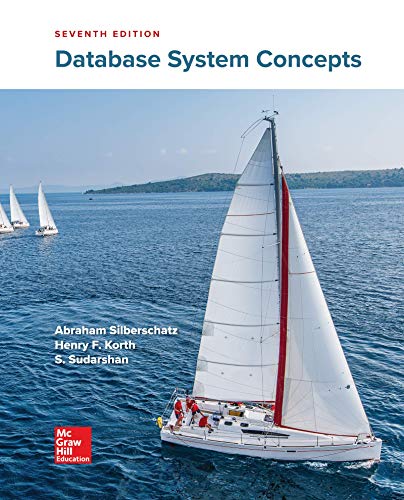
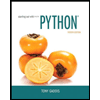
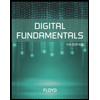
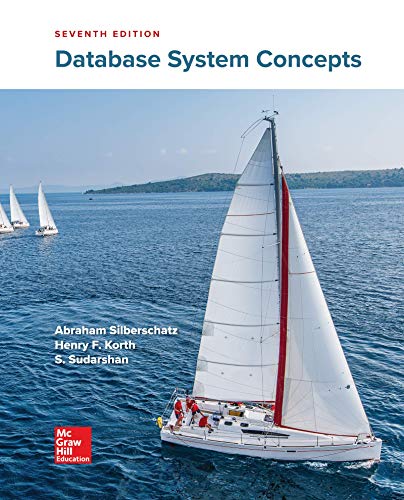
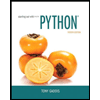
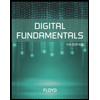
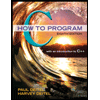
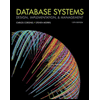
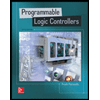