correct the syntax errors using System; class GFG { void KMPSearch(string pat, string txt) { int M = pat.Length; int N = txt.Length; int[] lps = new int[M]; int j = 0; int i = 0; while (i < N) { if (pat[j] == txt[i]) { j++; i++; } if (j == M) { Console.Write("Found pattern " j = lps[j - 1]; } else if (i < N && pat[j] != txt[i]) { if (j != 0) j = lps[j - 1]; else i = i + 1; } } } void computeLPSArray(string pat, int M, int[] lps) { int len = 0; int i = 1; lps[0] = 0; while (i < M) { if (pat[i] == pat[len]) { len++; lps[i] = len; i++; } else { if (len != 0) { len = lps[len - 1]; } else { lps[i] = len; i++; } } } } public static void Main() { string txt = "ABABDABACDABABCABAB"; new GFG().KMPSearch(pat, txt); } }
correct the syntax errors
using System;
class GFG {
void KMPSearch(string pat, string txt)
{
int M = pat.Length;
int N = txt.Length;
int[] lps = new int[M];
int j = 0;
int i = 0;
while (i < N) {
if (pat[j] == txt[i]) {
j++;
i++;
}
if (j == M) {
Console.Write("Found pattern "
j = lps[j - 1];
}
else if (i < N && pat[j] != txt[i]) {
if (j != 0)
j = lps[j - 1];
else
i = i + 1;
}
}
}
void computeLPSArray(string pat, int M, int[] lps)
{
int len = 0;
int i = 1;
lps[0] = 0;
while (i < M) {
if (pat[i] == pat[len]) {
len++;
lps[i] = len;
i++;
}
else
{
if (len != 0) {
len = lps[len - 1];
}
else
{
lps[i] = len;
i++;
}
}
}
}
public static void Main()
{
string txt = "ABABDABACDABABCABAB";
new GFG().KMPSearch(pat, txt);
}
}
![main.cpp:4:17: error: 'string' has not been declared
4 | void KMPSearch(string pat, string txt)
main.cpp:4:29: error: string' has not been declared
4 | void KMPSearch(string pat, string txt)
Aununun
main.cpp:31:25: error: string' has not been declared
31 |
void computelPSArray(string pat, int M, int[] lps)
main.cpp:31:50: error: expected
or .
before lps'
31 |
void computeLlPSArray(string pat, int M, int[] lps)
main.cpp:55:8: error: expected :' before 'static'
55 | public static void Main()
main.cpp:61:2: error: expected ;' after class definition
61 | }
|
main.cpp: In member function 'void GFG::KMPSearch(int, int)':
main.cpp:6:15: error: request for member Length' in 'pat', which is of non-class type 'int'
6 |
int M = pat.Length;
main.cpp:7:15: error: request for member Length' in txt’, which is of non-class type 'int'
int N = txt.Length;
main.cpp:8:6: warning: structured bindings only available with -std3c++17' or (-std=gnu++17'
8 |
int[] lps
new int[M];](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1a2ba6b3-393c-46f7-b189-5deedb6a552a%2F61b3dd88-5bf4-4dce-8011-ce7cedd53e8e%2Fpqwwpq_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

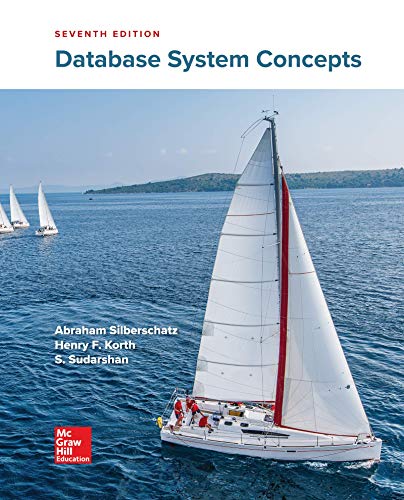
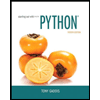
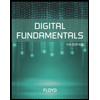
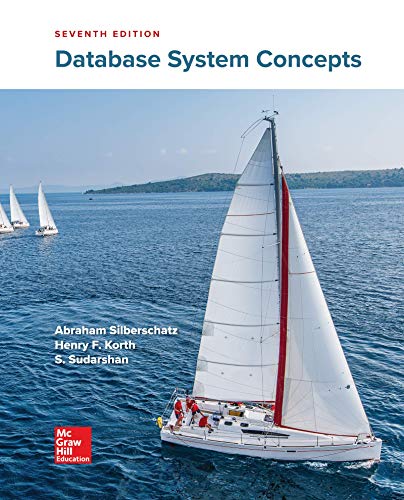
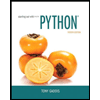
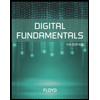
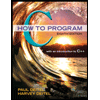
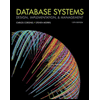
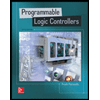