you please show the input and output of the code it would help very much. There is also a text file which is named "input.txt" and it reads. Different
In c++. The instructions are in the image. Please do not change existing code below just add to what is needed. I am very confused. Can you please show the input and output of the code it would help very much. There is also a text file which is named "input.txt" and it reads. Different solutions i have seen but i keep getting errors and errors i am still confused.
Movie 1
1
Genre1
Movie 2
2
Genre2
Movie 3
3
Genre3
Movie 4
4
Genre4
Movie 5
5
Genre5
main.cpp
#include <iostream>
#include <
#include <string>
#include "functions.h"
int main()
{
vector<movie> movies;
char option;
while (true)
{
printMenu();
cin >> option;
cin.ignore();
switch (option)
{
case 'A':
{
string nm;
int year;
string genre;
cout << "Movie Name: ";
getline(cin, nm);
cout << "Year: ";
cin >> year;
cout << "Genre: ";
cin >> genre;
//call you addMovie() here
cout << "Added " << nm << " to the catalog" << endl;
break;
}
case 'R':
{
string mn;
cout << "Movie Name:";
getline(cin, mn);
bool found;
found = //call you removeMovie()here
if (found == false)
cout << "Cannot find " << mn << endl;
else
cout << "Removed " << mn << " from catalog" << endl;
break;
}
case 'O':
{
string mn;
cout << "Movie Name: ";
getline(cin, mn);
cout << endl;
//call you movieInfo function here
break;
}
case 'C':
{
cout << "There are " << movies.size() << " movies in the catalog" << endl;
// Call the printCatalog function here
break;
}
case 'F':
{
string inputFile;
bool isOpen;
cin >> inputFile;
cout << "Reading catalog info from " << inputFile << endl;
isOpen = //call you readFromFile() in here
if (isOpen == false)
cout << "File not found" << endl;
break;
}
case 'W':
{ string outputFile;
bool isOpen;
cin >> outputFile;
cout << "Writing catalog info to " << outputFile << endl;
isOpen = //call you writeToFile() in here
if (isOpen == false)
cout << "File not found" << endl;
break;
}
}
if (option == 'Q')
{
cout << "Quitting Program";
break;
}
}
}
functions.h
#ifndef FUNCTIONS_H
#define FUNCTIONS_H
#include <iostream>
#include <vector>
#include <string>
//include necessary libraries
using namespace std;
// Define the structure "movie" here
void printMenu()
{
cout << endl;
cout << "Menu:" << endl;
cout << "A - Add Movie" << endl;
cout << "R - Remove Movie" << endl;
cout << "O - Output Movie Info" << endl;
cout << "C - Output Catalog Info" << endl;
cout << "F - Read file" << endl;
cout << "W - Write file" << endl;
cout << "Q - Quit Program" << endl;
cout << "Choose an option: ";
}
void printMovieInfo(const string &mn, int yr, const string &gen)
{
cout << endl;
cout << "Name: " << mn << endl;
cout << "Year: " << yr << endl;
cout << "Genre: " << gen << endl;
}
// Write the definition and implementation of the printCatalog function here
// Write the definition and implementation of the findMovie function here
// Write the definition and implementation of the addMovie function here
// Write the definition and implementation of the removeMovie function here
// Write the definition and implementation of the movieInfo function here
// You must use the following cout statement if the movie is not in the catalog:
// cout << "Cannot find " << /*movie name variable identifier*/ << endl;
// Write the definition and implementation of the readFromFile function here
// Write the definition and implementation of the writeToFile function here
#endif


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 13 images

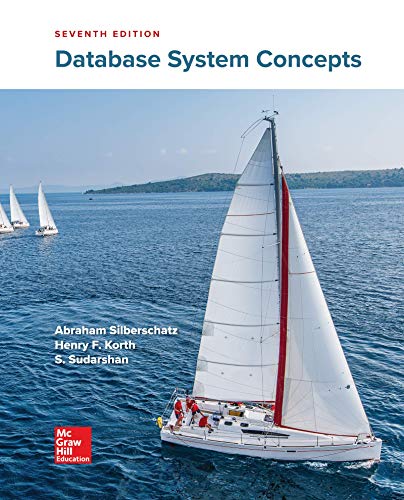
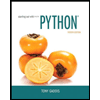
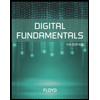
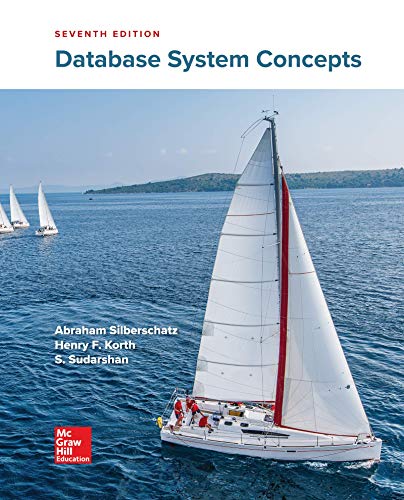
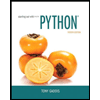
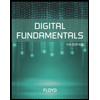
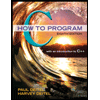
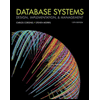
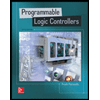