read the program; it looks a lot like warm up 2, right? instead of reading data from a text file to your program, you'll be inputting numbers into an array, then copying those numbers to a file created by your .cpp program after running the program, look for (and hopefully find) the text file (should be in the same folder as your.cpp file) then, add the following to the program: o input validation, so that only valid quiz scores are entered (not less than zero nor greater than 100) • statements that will compute the average quiz score and write the average, in a sentence that says "Your average score is save and run the program; confirm that input validation works, and the average sentence appears at the end of your myData.txt file
answer in c++
![V/input values into an array
//copying that data to a text file, which is created by the
- CPP
program
//text file should be in same folder as
cpp file
#include <iostream>
#include <fstream>
using namespace std;
int main ()
{
const int QUIZZES = 5;
int scores [QUIZZES];
//array size
//declaring array with 5 elements
//loop counter variable
//output file stream object
int count = 0;
ofstream outputFile;
//store values in the array
for (count = 0; count < QUIZZES; count++)
{
cout << "Enter a quiz score: ";
cin >> scores [count];
}
//creates and opens file for output
outputFile.open ("myData.txt");
//write array contents to ouput fil
for (count = 0; count < QUIZZES; count++)
outputFile <« scores [count] <<endl;
//close the file
outputFile.close ();
//confirm data written to output file:
cout << "The data was saved to the file.\n";
return 0;
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff1944717-129f-4ffb-945b-65eb0ebca594%2Fe5e6ff23-2841-40f0-aa6e-60cc802083a5%2Fsevbjaq_processed.png&w=3840&q=75)


Solution:
In the code that is given in the question :
- input validation can be performed as follows :
// performing input validation to check if the score <=0 and score>100
while (scores[count] <= 0 || scores[count] > 100)
{
cout << "value not accepted.\n";
cin >> scores[count];
}
- The average of quiz scores can be done as follows:
sum= sum + scores[count];
avg = sum /quizzes;
// code:
#include <iostream>
#include <fstream>
using namespace std;
int main()
{ const int quizzes =5;
int scores[quizzes];
int count =0;
int sum=0;
double avg = 0.0;
ofstream outputfile;
if (scores == NULL)
return 0;
//Get the quizz score for each test
cout << "Enter the quizz scores below.\n";
for (int count = 0; count <quizzes ; count++)
{
cin >> scores[count];
// performing input validation to check if the score <=0 and score>100
while (scores[count] <= 0 || scores[count] > 100)
{
cout << "value not accepted.\n";
cin >> scores[count];
}
// calculating the average of quiz scores
sum= sum + scores[count];
avg = sum /quizzes;
}
outputfile.open("myData.txt");
for(count=0;count<quizzes;count++)
outputfile <<scores[count] << endl;
outputfile <<"your average score is " <<avg <<endl;
outputfile.close();
cout<<" The data was saved to file";
cout<< "\n your average score is"<<avg<<endl;
return 0;
}
Step by step
Solved in 3 steps with 2 images

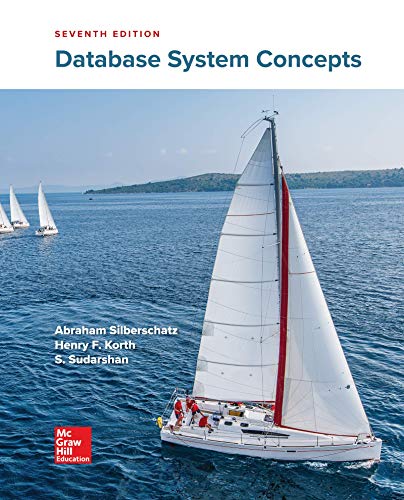
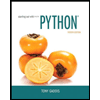
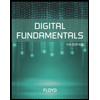
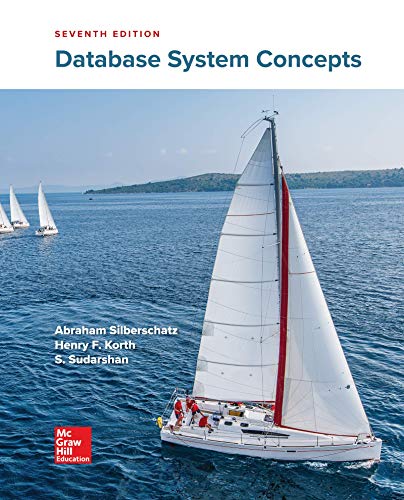
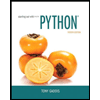
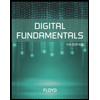
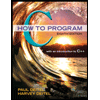
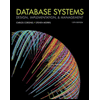
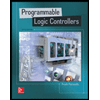