I am trying to simplify this code, in python, so that I do not have to list each file separately. Is there a way that I can access the FITS files via a folder on my Desktop to produce one plot of several FITS files. import numpy as np import matplotlib.pyplot as plt from astropy.io import fits from astropy.wcs import WCS %matplotlib inline %matplotlib widget plt.figure(figsize=(10,10)) legends = [] def plot_fits_file(file_path): # used this data to test ---------- # lam = np.random.random(100) # flux = np.random.random(100) # -------------------- hdul = fits.open(file_path) data = hdul[1].data h1 = hdul[1].header flux = data[1] w = WCS(h1, naxis=1, relax=False, fix=False) lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0] plt.plot(lam, flux) plt.ylim(0, ) plt.xlabel('RV[km/s]') plt.ylabel('Normalized CCF') legends.append(file_path) spec_list = [ '~/Desktop/r.HARPN.2018-01-18T14:25:36.145_CCF_A.fits', '~/Desktop/r.HARPN.2018-01-18T14:20:10.645_CCF_A.fits', '~/Desktop/r.HARPN.2018-01-18T14:31:00.875_CCF_A.fits', '~/Desktop/r.HARPN.2018-01-18T14:36:26.373_CCF_A.fits'] for file_path in spec_list: plot_fits_file(file_path) plt.show()
I am trying to simplify this code, in python, so that I do not have to list each file separately.
Is there a way that I can access the FITS files via a folder on my Desktop to produce one plot of several FITS files.
import numpy as np
import matplotlib.pyplot as plt
from astropy.io import fits
from astropy.wcs import WCS
%matplotlib inline
%matplotlib widget
plt.figure(figsize=(10,10))
legends = []
def plot_fits_file(file_path):
# used this data to test ----------
# lam = np.random.random(100)
# flux = np.random.random(100)
# --------------------
hdul = fits.open(file_path)
data = hdul[1].data
h1 = hdul[1].header
flux = data[1]
w = WCS(h1, naxis=1, relax=False, fix=False)
lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0]
plt.plot(lam, flux)
plt.ylim(0, )
plt.xlabel('RV[km/s]')
plt.ylabel('Normalized CCF')
legends.append(file_path)
spec_list = [
'~/Desktop/r.HARPN.2018-01-18T14:25:36.145_CCF_A.fits',
'~/Desktop/r.HARPN.2018-01-18T14:20:10.645_CCF_A.fits',
'~/Desktop/r.HARPN.2018-01-18T14:31:00.875_CCF_A.fits',
'~/Desktop/r.HARPN.2018-01-18T14:36:26.373_CCF_A.fits']
for file_path in spec_list:
plot_fits_file(file_path)
plt.show()
![←
00
80000
60000
40000
20000
0 T
10
T
20
Figure 1
30
RV[km/s]
40
50](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc1c1ced3-1875-44c4-84f9-6bbf6b04c124%2F4d5547db-32cc-4ed5-b734-f9ec74d36523%2Fd2xctjg_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps

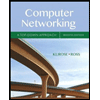
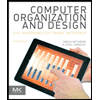
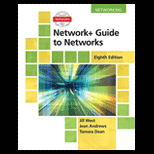
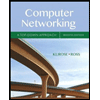
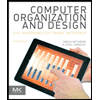
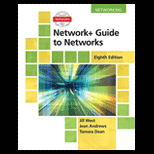
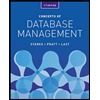
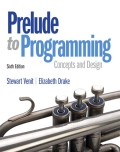
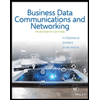