B: Artwork label (modules) Ex: If the input is: Pablo Picasso 1881 1973 Three Musicians 1921 the output is: Artist: Pablo Picasso (1881 to 1973) Title: Three Musicians, 1921 Ex: If the input is: Brice Marden 1938 -1 Distant Muses 2000 the output is:
Hello,
I am needing help with this lab. The code is correct. I am having trouble with the spacing. Please see the lab
2.15 LAB: Artwork label (modules)
Ex: If the input is:
Pablo Picasso 1881 1973 Three Musicians 1921
the output is:
Artist: Pablo Picasso (1881 to 1973) Title: Three Musicians, 1921
Ex: If the input is:
Brice Marden 1938 -1 Distant Muses 2000
the output is:
Artist: Brice Marden (1938 to present) Title: Distant Muses, 2000
Ex: If the input is:
Banksy -1 -1 Balloon Girl 2002
the output is:
Artist: Banksy (unknown) Title: Balloon Girl, 2002
Please view current attachments:
The current code that I have, is currently uploaded to make it easier. As I stated before the code is correct, it is just the spacing that I need help with to correct the spacing errors. Please attach a screen shot of the code, like I have done. It helps with spacing and less errors.
Please review the spacing errors as well.
Thank you for all of your help.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

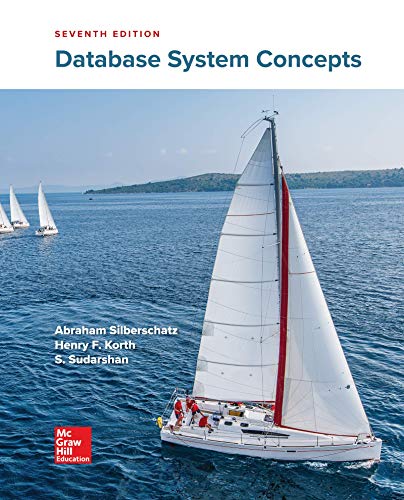
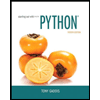
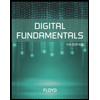
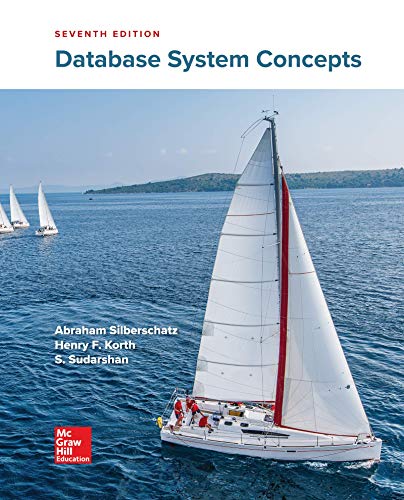
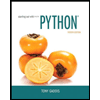
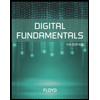
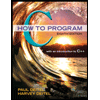
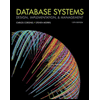
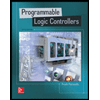