I need help coming up with more detailed comments for each section of code, separating the logic into different classes or methods to improve code modularity and readability and in the "fileMenu" ActionListener, the catch block only prints the stack trace and shows a message dialog. It would be better to provide more informative error messages to the user, indicating the cause of the error. Also the frame size is set to 400x400 pixels using the numbers directly in the code. What would constants or variables to represent these numbers look like? Thank you Source code: package creatingInterface; import javax.swing.*; import java.awt.*; import java.io.FileWriter; import java.io.IOException; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Random; public class userInterface { private static Color randomGreen; public static void main(String[] args) { // Create and set up the window. JFrame frame = new JFrame("User Interface"); // Create a new JFrame with the title "User Interface". frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Set the default close operation to exit the application when the window is closed. // Creating the MenuBar and adding components. JMenuBar menuBar = new JMenuBar(); // Create a new JMenuBar. JMenu mainMenu = new JMenu("Menu"); // Create a new JMenu with the label "Menu". menuBar.add(mainMenu); // Add the menu to the menu bar. JMenuItem dateMenu = new JMenuItem("Today's Date"); // Create a new JMenuItem with the label "Today's Date". JMenuItem fileMenu = new JMenuItem("Write to File"); // Create a new JMenuItem with the label "Write to File". JMenuItem colorMenu = new JMenuItem("Change Background Color"); // Create a new JMenuItem with the label "Change Background Color". JMenuItem exitMenu = new JMenuItem("Exit"); // Create a new JMenuItem with the label "Exit". mainMenu.add(dateMenu); // Add the menu item to the menu. mainMenu.add(fileMenu); // Add the menu item to the menu. mainMenu.add(colorMenu); // Add the menu item to the menu. mainMenu.add(exitMenu); // Add the menu item to the menu. // Text Area at the Center. JTextArea textArea = new JTextArea(); // Create a new JTextArea. textArea.setEditable(false); // Set the text area as non-editable. // Adding Action to menu item dateMenu. dateMenu.addActionListener(e -> { // When "Show Date" menu item is clicked, set the text area with the current date and time. textArea.setText(new SimpleDateFormat("yyyy.MM.dd.HH.mm.ss").format(new Date())); }); // Adding Action to menu item fileMenu. fileMenu.addActionListener(e -> { // When "Write to File" menu item is clicked, write the text area content to the file. try (FileWriter fw = new FileWriter("log.txt")) { fw.write(textArea.getText()); JOptionPane.showMessageDialog(frame, "Contents written to log.txt successfully!"); // If successful print success message. } // If unsuccessful print error message. catch (IOException ioException) { ioException.printStackTrace(); JOptionPane.showMessageDialog(frame, "Error writing to log.txt!"); } }); // Adding Action to menu item colorMenu. colorMenu.addActionListener(e -> { // When "Change Background Color" menu item is clicked, change the background color of the frame to random hue of green. if (randomGreen == null) { // Generating a random green color if it hasn't been set yet. Random green = new Random(); float r = green.nextFloat() / 2f; float g = 0.5f + green.nextFloat() / 2f; float b = green.nextFloat() / 2f; randomGreen = new Color(r, g, b); // New green } textArea.setBackground(randomGreen); // Setting green background. }); // Adding action to menu item exitMenu. exitMenu.addActionListener(e -> { // When "Exit" menu item is clicked, exit the program. JOptionPane.showMessageDialog(frame, "Exiting interface, Goodbye."); // Print exit message. System.exit(0); }); // Adding Components to the frame. frame.getContentPane().add(BorderLayout.CENTER, textArea); // Add the text area to the center of the frame. frame.getContentPane().add(BorderLayout.NORTH, menuBar); // Add the menu bar to the north of the frame. frame.setSize(400, 400); // Set the size of the frame to 400x400 pixels. frame.setVisible(true); // Make the frame visible. } }
I need help coming up with more detailed comments for each section of code, separating the logic into different classes or methods to improve code modularity and readability and in the "fileMenu" ActionListener, the catch block only prints the stack trace and shows a message dialog. It would be better to provide more informative error messages to the user, indicating the cause of the error. Also the frame size is set to 400x400 pixels using the numbers directly in the code. What would constants or variables to represent these numbers look like?
Thank you
Source code:
package creatingInterface;
import javax.swing.*;
import java.awt.*;
import java.io.FileWriter;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Random;
public class userInterface {
private static Color randomGreen;
public static void main(String[] args) {
// Create and set up the window.
JFrame frame = new JFrame("User Interface"); // Create a new JFrame with the title "User Interface".
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Set the default close operation to exit the application when the window is closed.
// Creating the MenuBar and adding components.
JMenuBar menuBar = new JMenuBar(); // Create a new JMenuBar.
JMenu mainMenu = new JMenu("Menu"); // Create a new JMenu with the label "Menu".
menuBar.add(mainMenu); // Add the menu to the menu bar.
JMenuItem dateMenu = new JMenuItem("Today's Date"); // Create a new JMenuItem with the label "Today's Date".
JMenuItem fileMenu = new JMenuItem("Write to File"); // Create a new JMenuItem with the label "Write to File".
JMenuItem colorMenu = new JMenuItem("Change Background Color"); // Create a new JMenuItem with the label "Change Background Color".
JMenuItem exitMenu = new JMenuItem("Exit"); // Create a new JMenuItem with the label "Exit".
mainMenu.add(dateMenu); // Add the menu item to the menu.
mainMenu.add(fileMenu); // Add the menu item to the menu.
mainMenu.add(colorMenu); // Add the menu item to the menu.
mainMenu.add(exitMenu); // Add the menu item to the menu.
// Text Area at the Center.
JTextArea textArea = new JTextArea(); // Create a new JTextArea.
textArea.setEditable(false); // Set the text area as non-editable.
// Adding Action to menu item dateMenu.
dateMenu.addActionListener(e -> {
// When "Show Date" menu item is clicked, set the text area with the current date and time.
textArea.setText(new SimpleDateFormat("yyyy.MM.dd.HH.mm.ss").format(new Date()));
});
// Adding Action to menu item fileMenu.
fileMenu.addActionListener(e -> {
// When "Write to File" menu item is clicked, write the text area content to the file.
try (FileWriter fw = new FileWriter("log.txt")) {
fw.write(textArea.getText());
JOptionPane.showMessageDialog(frame, "Contents written to log.txt successfully!"); // If successful print success message.
}
// If unsuccessful print error message.
catch (IOException ioException) {
ioException.printStackTrace();
JOptionPane.showMessageDialog(frame, "Error writing to log.txt!");
}
});
// Adding Action to menu item colorMenu.
colorMenu.addActionListener(e -> {
// When "Change Background Color" menu item is clicked, change the background color of the frame to random hue of green.
if (randomGreen == null) {
// Generating a random green color if it hasn't been set yet.
Random green = new Random();
float r = green.nextFloat() / 2f;
float g = 0.5f + green.nextFloat() / 2f;
float b = green.nextFloat() / 2f;
randomGreen = new Color(r, g, b); // New green
}
textArea.setBackground(randomGreen); // Setting green background.
});
// Adding action to menu item exitMenu.
exitMenu.addActionListener(e -> {
// When "Exit" menu item is clicked, exit the program.
JOptionPane.showMessageDialog(frame, "Exiting interface, Goodbye."); // Print exit message.
System.exit(0);
});
// Adding Components to the frame.
frame.getContentPane().add(BorderLayout.CENTER, textArea); // Add the text area to the center of the frame.
frame.getContentPane().add(BorderLayout.NORTH, menuBar); // Add the menu bar to the north of the frame.
frame.setSize(400, 400); // Set the size of the frame to 400x400 pixels.
frame.setVisible(true); // Make the frame visible.
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

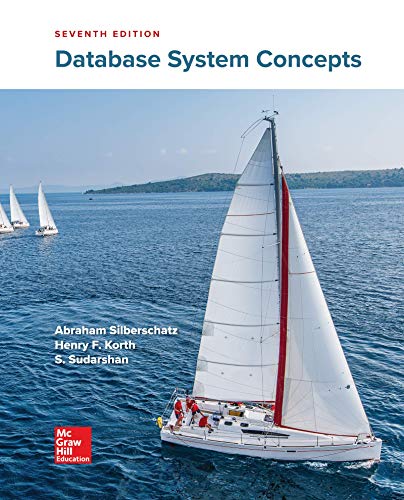
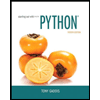
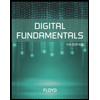
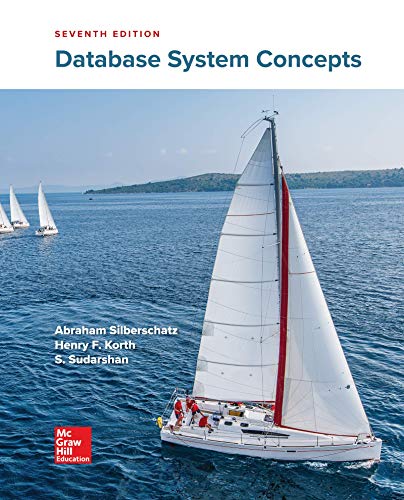
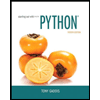
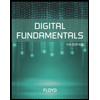
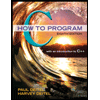
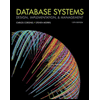
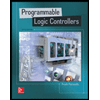