Need help with the following: Adding comments to help explain the purpose of methods, classes, constructors, etc. to help improve understanding. Implementing enhanced for loop (for each loop) to iterate over the numbers array in the calculateProduct method. Creating a separate class for the UI and a separate class for the calculator logic. Lastly, creating a constant instead of hard coding the number "5" in multiple places. Thank you for any input/knowldge you can transfer to me, I appreciate it. Source Code: package implementingRecursion; import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; public class RecursiveProductCalculator extends JFrame { private JTextField[] numberFields; private JButton calculateButton; private JLabel resultLabel; public RecursiveProductCalculator() { setTitle("Recursive Product Calculator"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLayout(new GridLayout(7, 1)); numberFields = new JTextField[5]; for (inti = 0; i < 5; i++) { JPanel panel = new JPanel(); panel.setLayout(new FlowLayout()); JLabel label = new JLabel("Number " + (i + 1) + ": "); numberFields[i] = new JTextField(10); panel.add(label); panel.add(numberFields[i]); add(panel); } calculateButton = new JButton("Calculate"); calculateButton.addActionListener(new ActionListener() { @Override publicvoid actionPerformed(ActionEvent e) { try { int[] numbers = newint[5]; for (inti = 0; i < 5; i++) { // Perform validation for each input field if (!numberFields[i].getText().matches("-?\\d+")) { JOptionPane.showMessageDialog(null, "Invalid input in Number " + (i + 1) + "."); return; } numbers[i] = Integer.parseInt(numberFields[i].getText()); } intproduct = calculateProduct(numbers, 0); resultLabel.setText("Product: " + product); } catch (NumberFormatException ex) { JOptionPane.showMessageDialog(null, "Invalid number format. Please enter valid integers."); } } }); add(calculateButton); resultLabel = new JLabel("Product: "); add(resultLabel); pack(); setVisible(true); } privateint calculateProduct(int[] numbers, intindex) { if (index == numbers.length - 1) { returnnumbers[index]; } else { returnnumbers[index] * calculateProduct(numbers, index + 1); } } publicstaticvoid main(String[] args) { SwingUtilities.invokeLater(new Runnable() { @Override publicvoid run() { new RecursiveProductCalculator(); } }); } }
Need help with the following:
- Adding comments to help explain the purpose of methods, classes, constructors, etc. to help improve understanding.
- Implementing enhanced for loop (for each loop) to iterate over the numbers array in the calculateProduct method.
- Creating a separate class for the UI and a separate class for the calculator logic.
- Lastly, creating a constant instead of hard coding the number "5" in multiple places.
Thank you for any input/knowldge you can transfer to me, I appreciate it.
Source Code:
package implementingRecursion;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class RecursiveProductCalculator extends JFrame {
private JTextField[] numberFields;
private JButton calculateButton;
private JLabel resultLabel;
public RecursiveProductCalculator() {
setTitle("Recursive Product Calculator");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new GridLayout(7, 1));
numberFields = new JTextField[5];
for (inti = 0; i < 5; i++) {
JPanel panel = new JPanel();
panel.setLayout(new FlowLayout());
JLabel label = new JLabel("Number " + (i + 1) + ": ");
numberFields[i] = new JTextField(10);
panel.add(label);
panel.add(numberFields[i]);
add(panel);
}
calculateButton = new JButton("Calculate");
calculateButton.addActionListener(new ActionListener() {
@Override
publicvoid actionPerformed(ActionEvent e) {
try {
int[] numbers = newint[5];
for (inti = 0; i < 5; i++) {
// Perform validation for each input field
if (!numberFields[i].getText().matches("-?\\d+")) {
JOptionPane.showMessageDialog(null, "Invalid input in Number " + (i + 1) + ".");
return;
}
numbers[i] = Integer.parseInt(numberFields[i].getText());
}
intproduct = calculateProduct(numbers, 0);
resultLabel.setText("Product: " + product);
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(null, "Invalid number format. Please enter valid integers.");
}
}
});
add(calculateButton);
resultLabel = new JLabel("Product: ");
add(resultLabel);
pack();
setVisible(true);
}
privateint calculateProduct(int[] numbers, intindex) {
if (index == numbers.length - 1) {
returnnumbers[index];
} else {
returnnumbers[index] * calculateProduct(numbers, index + 1);
}
}
publicstaticvoid main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
publicvoid run() {
new RecursiveProductCalculator();
}
});
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Does anyone else want to take a stab at the
Thank you
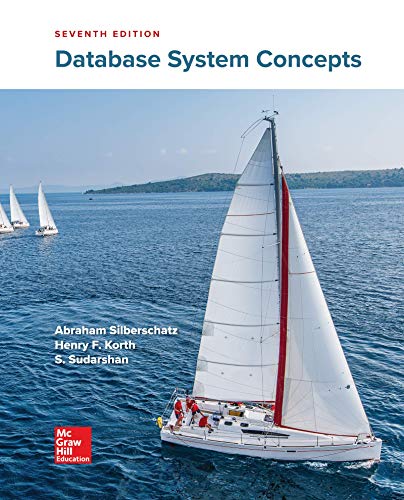
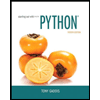
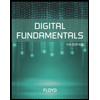
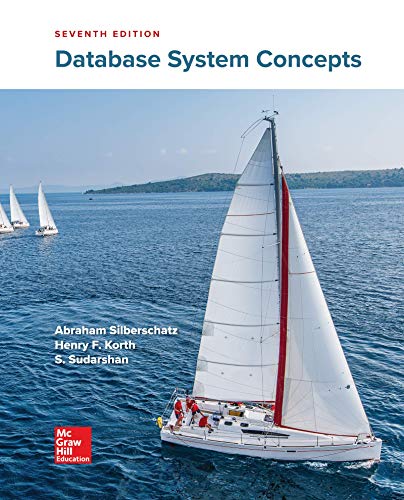
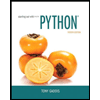
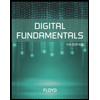
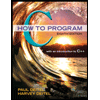
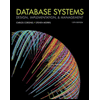
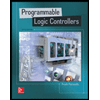