Final help needed for Python Turtle, does my code have everything required, and if not can you help make the changes needed. 1. An additional option to generate another island if the user does not like the one they have (loops until they are satisfied) and have the GUIs bigger.2. Does my code have two or more functions (I dont know if the drawing only counts as one)?3. Does my code have atleast one list, dictionary, or set (if not reorganize/add one)?4. Need help exporting the finished turtle image into a file on desktop (yes/no option input by user) that can be accessed, or png (In ###'s I saved the broken code that i dont know how to fix) My code: import turtleimport randomimport tkinter as tkfrom tkinter import simpledialog### OPTIONAL IMAGE CONVERTER, REMOVE ###'S TO USE###from PIL import Image# Colors:ocean = "#000066"sand = "#ffff66"grass = "#00cc00"lake = "#000066"mountain_color = "#808080" mountain_positions = []def draw_irregular_circle(radius, line_color, fill_color, irregularity=30, spikeyness=4): my_turtle.color(line_color) my_turtle.fillcolor(fill_color) my_turtle.begin_fill() angle = 0 while angle < 360: angle += random.uniform(10, 60) if angle > 360: angle = 360 actual_radius = radius + random.uniform(irregularity * spikeyness, irregularity) * random.choice([-1, 1]) my_turtle.circle(actual_radius, angle - my_turtle.heading()) my_turtle.end_fill()def draw_triangle(size, line_color, fill_color): my_turtle.color(line_color) my_turtle.fillcolor(fill_color) my_turtle.begin_fill() for _ in range(3): my_turtle.forward(size) my_turtle.left(120) my_turtle.end_fill()def move_turtle(x, y): my_turtle.penup() my_turtle.goto(x, y) my_turtle.pendown()def draw_lake(): x = random.randint(-200, 300) y = random.randint(-50, 200) move_turtle(x, y) draw_irregular_circle(52, lake, lake, 39, 0.6) def draw_mountain(): x = random.randint(-200, 300) y = random.randint(-50, 250) mountain_positions.append((x, y))def draw_river(): start_x = random.randint(-500, -400) start_y = random.randint(-600, 200) end_x = start_x + 1000 end_y = start_y + 700 move_turtle(start_x, start_y) my_turtle.setheading(my_turtle.towards(end_x, end_y)) my_turtle.color(ocean) my_turtle.pensize(45) my_turtle.pendown() my_turtle.goto(end_x, end_y) my_turtle.penup()def get_user_input(): root = tk.Tk() root.withdraw() user_input = simpledialog.askstring("Input", "Enter the number of lakes, mountains, and rivers separated by commas (e.g., 3,2,1):") num_lakes, num_mountains, num_rivers = map(int, user_input.split(',')) root.destroy() return num_lakes, num_mountains, num_riversnum_lakes, num_mountains, num_rivers = get_user_input()screen = turtle.Screen()screen.setup(width=1200, height=800) #Increase window size (dont touch)screen.bgcolor(ocean)screen.title("Island Generator")my_turtle = turtle.Turtle()my_turtle.pensize(4)my_turtle.shape("circle")move_turtle(0, -300)draw_irregular_circle(300, sand, sand, 200, 0.4) move_turtle(0, -300)draw_irregular_circle(250, grass, grass, 150, 0.4) for _ in range(num_lakes): draw_lake()for _ in range(num_mountains): draw_mountain()for pos in mountain_positions: move_turtle(pos[0], pos[1]) draw_triangle(135, mountain_color, mountain_color) for _ in range(num_rivers): draw_river()# Save the final result as an image below (remove ### to activate)### canvas = screen.getcanvas()### canvas.postscript(file="island.ps") # Save the postscript file### img = Image.open("island.ps")### img.save("island.png", "png") # Convert to PNGturtle.done()
Final help needed for Python Turtle, does my code have everything required, and if not can you help make the changes needed.
1. An additional option to generate another island if the user does not like the one they have (loops until they are satisfied) and have the GUIs bigger.
2. Does my code have two or more functions (I dont know if the drawing only counts as one)?
3. Does my code have atleast one list, dictionary, or set (if not reorganize/add one)?
4. Need help exporting the finished turtle image into a file on desktop (yes/no option input by user) that can be accessed, or png (In ###'s I saved the broken code that i dont know how to fix)
My code:
import turtle
import random
import tkinter as tk
from tkinter import simpledialog
### OPTIONAL IMAGE CONVERTER, REMOVE ###'S TO USE
###from PIL import Image
# Colors:
ocean = "#000066"
sand = "#ffff66"
grass = "#00cc00"
lake = "#000066"
mountain_color = "#808080"
mountain_positions = []
def draw_irregular_circle(radius, line_color, fill_color, irregularity=30, spikeyness=4):
my_turtle.color(line_color)
my_turtle.fillcolor(fill_color)
my_turtle.begin_fill()
angle = 0
while angle < 360:
angle += random.uniform(10, 60)
if angle > 360:
angle = 360
actual_radius = radius + random.uniform(irregularity * spikeyness, irregularity) * random.choice([-1, 1])
my_turtle.circle(actual_radius, angle - my_turtle.heading())
my_turtle.end_fill()
def draw_triangle(size, line_color, fill_color):
my_turtle.color(line_color)
my_turtle.fillcolor(fill_color)
my_turtle.begin_fill()
for _ in range(3):
my_turtle.forward(size)
my_turtle.left(120)
my_turtle.end_fill()
def move_turtle(x, y):
my_turtle.penup()
my_turtle.goto(x, y)
my_turtle.pendown()
def draw_lake():
x = random.randint(-200, 300)
y = random.randint(-50, 200)
move_turtle(x, y)
draw_irregular_circle(52, lake, lake, 39, 0.6)
def draw_mountain():
x = random.randint(-200, 300)
y = random.randint(-50, 250)
mountain_positions.append((x, y))
def draw_river():
start_x = random.randint(-500, -400)
start_y = random.randint(-600, 200)
end_x = start_x + 1000
end_y = start_y + 700
move_turtle(start_x, start_y)
my_turtle.setheading(my_turtle.towards(end_x, end_y))
my_turtle.color(ocean)
my_turtle.pensize(45)
my_turtle.pendown()
my_turtle.goto(end_x, end_y)
my_turtle.penup()
def get_user_input():
root = tk.Tk()
root.withdraw()
user_input = simpledialog.askstring("Input", "Enter the number of lakes, mountains, and rivers separated by commas (e.g., 3,2,1):")
num_lakes, num_mountains, num_rivers = map(int, user_input.split(','))
root.destroy()
return num_lakes, num_mountains, num_rivers
num_lakes, num_mountains, num_rivers = get_user_input()
screen = turtle.Screen()
screen.setup(width=1200, height=800) #Increase window size (dont touch)
screen.bgcolor(ocean)
screen.title("Island Generator")
my_turtle = turtle.Turtle()
my_turtle.pensize(4)
my_turtle.shape("circle")
move_turtle(0, -300)
draw_irregular_circle(300, sand, sand, 200, 0.4)
move_turtle(0, -300)
draw_irregular_circle(250, grass, grass, 150, 0.4)
for _ in range(num_lakes):
draw_lake()
for _ in range(num_mountains):
draw_mountain()
for pos in mountain_positions:
move_turtle(pos[0], pos[1])
draw_triangle(135, mountain_color, mountain_color)
for _ in range(num_rivers):
draw_river()
# Save the final result as an image below (remove ### to activate)
### canvas = screen.getcanvas()
### canvas.postscript(file="island.ps") # Save the postscript file
### img = Image.open("island.ps")
### img.save("island.png", "png") # Convert to PNG
turtle.done()

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

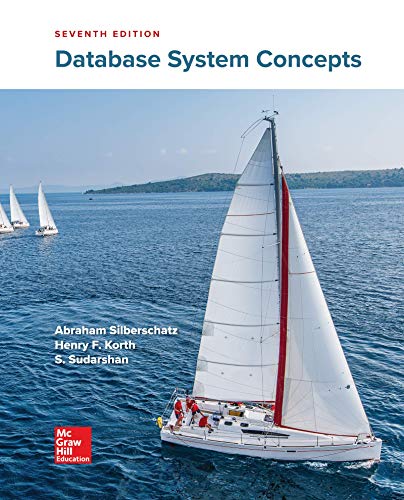
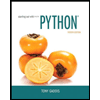
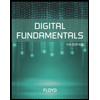
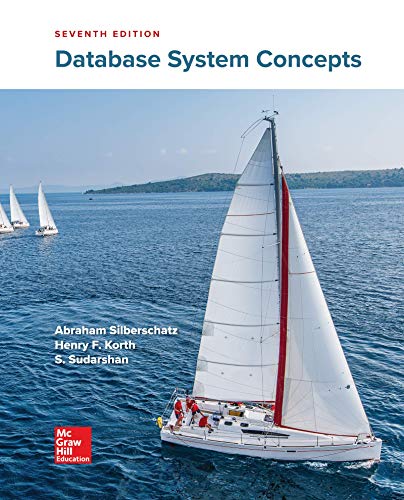
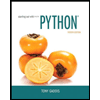
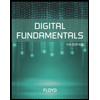
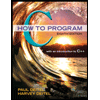
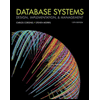
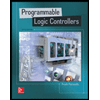