Objective: Write a Java program practicing the use of arrays in addition to the other programming practices you have learned, such as program decomposition and the use of Java class (static) methods. Monolithic solutions that do not exhibit good design practices and appropriate class methods will not receive full credit. Use arrays for this. Do not use ArrayLists. Material Provided: You will need the following file to complete this lab: info.txt (A sample input file, which is available on Carmen.) Set up You will need to create your own implementation of the Student class. It will look much like the Student class we saw in the class PowerPoint slides. You may take some shortcuts though. Knowing that the data will always be sets of four lines of text which contain a student name and three scores, you can get away with a constructor that takes the name and three scores and creates the instance of the Student object. (You will still need to get the data out of it though, so don’t forget those methods.) Also, given that you will be reading the number of students in the “class group” as the first item of data read from the file, you can allocate the array to hold the students in the program. After that you can read the data in groups of four lines, to get the complete set of data for each student, and allocate new memory for that data in your array. Hints: for loops will be helpful in this assignment. Read all input as Strings. Use Integer.parseInt(String integer_value) to get the numeric value from it. To create each new element in the array of students, use the new keyword and the Student constructor. I.e., studentList[i] = new Student(line, Integer.parseInt(line1),… Don’t worry about methods in the main program. The objective here is to write a Student class, which will have a few instance methods in it. (My solution has two for loops in it with the necessary input and output statements to read from the file and print to the screen, The Student class file will look a lot like the Student class file in the set of notes on classes and objects. Put it under the same package as the class with main in it, so they are effectively side by side. Remember that it won’t have a main (or much of anything else except the class instance variables and methods themselves.) Don’t forget to include the “throws IOException” in the proper place. Remember that the backslash is a special character, so when you use it to enter a path name (such as “C:\\info.txt”) in Eclipse, you must enter a pair of them. Otherwise Windows will drop a single backslash and you get an incorrect path. (Later versions of Eclipse actually interpret the single backslash properly, it appears.) Other programming environments may handle this differently, and the path for a Macintosh is also different from this. Macintoshes use something like “users/user_name/documents/file_name”. Description Here is a sample interaction between a user and the program you will write in the exercise. (User input is in bold.) Enter file name: c:\\info.txt Name Score1 Score2 Score3 Total ----------------------------------------------------- Andy Borders 200 250 400 850 John Smith 120 220 330 670 John Borell 250 250 500 1000 Robert Fennel 200 150 350 700 Craig Fenner 230 220 480 930 Bill Johnson 120 150 220 490 Brent Garland 220 240 350 810 ----------------------------------------------------- The total number of student in this class is: 7 The average total score of the class is: 778 John Borell got the maximum score of: 1000 Bill Johnson got the minimum score of: 490 ----------------------------------------------------- Note that the filename supplied includes the full path. Specify a full path or the IDE will not find the file. The program performs the following actions: Ask the user for the name of a file containing information about student scores and input the file name. (See “Input file format” for a description of the format of the file.) Input the student information from the file and store in an appropriate array of Student objects. See “Setup” for a description of this. Output a well-formatted table containing the name, individual scores and total score for each student. Output the number of students in the class, the average total score, the maximum total score and the minimum total score in the class, including the names of the students with the maximum and minimum scores. To tabulate the output, you can count spaces or use tab characters. A tab character can be printed by using the ’\t’ escape sequence. For example, System.out.print(”1\t2\t3”) will output something like “1 2 3” where 1, 2 and 3 are separated by tabs.
Objective: Write a Java program practicing the use of arrays in addition to the other programming practices you have learned, such as program decomposition and the use of Java class (static) methods. Monolithic solutions that do not exhibit good design practices and appropriate class methods will not receive full credit.
Use arrays for this. Do not use ArrayLists.
Material Provided: You will need the following file to complete this lab:
- info.txt (A sample input file, which is available on Carmen.)
Set up
You will need to create your own implementation of the Student class. It will look much like the Student class we saw in the class PowerPoint slides. You may take some shortcuts though. Knowing that the data will always be sets of four lines of text which contain a student name and three scores, you can get away with a constructor that takes the name and three scores and creates the instance of the Student object. (You will still need to get the data out of it though, so don’t forget those methods.)
Also, given that you will be reading the number of students in the “class group” as the first item of data read from the file, you can allocate the array to hold the students in the program. After that you can read the data in groups of four lines, to get the complete set of data for each student, and allocate new memory for that data in your array.
Hints:
- for loops will be helpful in this assignment.
- Read all input as Strings. Use Integer.parseInt(String integer_value) to get the numeric value from it.
- To create each new element in the array of students, use the new keyword and the Student constructor. I.e., studentList[i] = new Student(line, Integer.parseInt(line1),…
- Don’t worry about methods in the main program. The objective here is to write a Student class, which will have a few instance methods in it. (My solution has two for loops in it with the necessary input and output statements to read from the file and print to the screen,
- The Student class file will look a lot like the Student class file in the set of notes on classes and objects. Put it under the same package as the class with main in it, so they are effectively side by side. Remember that it won’t have a main (or much of anything else except the class instance variables and methods themselves.)
- Don’t forget to include the “throws IOException” in the proper place.
- Remember that the backslash is a special character, so when you use it to enter a path name (such as “C:\\info.txt”) in Eclipse, you must enter a pair of them. Otherwise Windows will drop a single backslash and you get an incorrect path. (Later versions of Eclipse actually interpret the single backslash properly, it appears.) Other programming environments may handle this differently, and the path for a Macintosh is also different from this. Macintoshes use something like “users/user_name/documents/file_name”.
Description
Here is a sample interaction between a user and the program you will write in the exercise. (User input is in bold.)
Enter file name: c:\\info.txt
Name Score1 Score2 Score3 Total
-----------------------------------------------------
Andy Borders 200 250 400 850
John Smith 120 220 330 670
John Borell 250 250 500 1000
Robert Fennel 200 150 350 700
Craig Fenner 230 220 480 930
Bill Johnson 120 150 220 490
Brent Garland 220 240 350 810
-----------------------------------------------------
The total number of student in this class is: 7
The average total score of the class is: 778
John Borell got the maximum score of: 1000
Bill Johnson got the minimum score of: 490
-----------------------------------------------------
Note that the filename supplied includes the full path. Specify a full path or the IDE will not find the file.
The program performs the following actions:
- Ask the user for the name of a file containing information about student scores and input the file name. (See “Input file format” for a description of the format of the file.)
- Input the student information from the file and store in an appropriate array of Student objects. See “Setup” for a description of this.
- Output a well-formatted table containing the name, individual scores and total score for each student.
- Output the number of students in the class, the average total score, the maximum total score and the minimum total score in the class, including the names of the students with the maximum and minimum scores.
To tabulate the output, you can count spaces or use tab characters. A tab character can be printed by using the ’\t’ escape sequence. For example, System.out.print(”1\t2\t3”) will output something like “1 2 3” where 1, 2 and 3 are separated by tabs.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

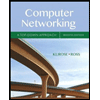
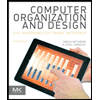
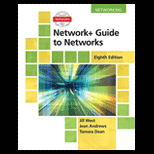
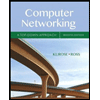
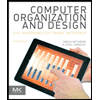
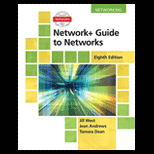
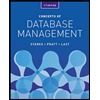
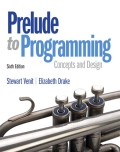
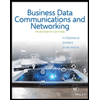