use the array built to shuffle and deal two poker hands. Change the corresponding values for all Jacks through Aces to 11, 12, 13, 14 respectively. Shuffle the deck, then deal two hands. Comment your code and submit the Python code. i wrote a code for it but its not displaying properly, can someone tell me why? (black and white is the array given/ the picture of the python app is what i wrote) its only displaying the array given and not the bottom code i put, why?
use the array built to shuffle and deal two poker hands. Change the corresponding values for all Jacks through Aces to 11, 12, 13, 14 respectively. Shuffle the deck, then deal two hands. Comment your code and submit the Python code. i wrote a code for it but its not displaying properly, can someone tell me why? (black and white is the array given/ the picture of the python app is what i wrote) its only displaying the array given and not the bottom code i put, why?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
use the array built to shuffle and deal two poker hands. Change the corresponding values for all Jacks through Aces to 11, 12, 13, 14 respectively.
Shuffle the deck, then deal two hands. Comment your code and submit the Python code.
i wrote a code for it but its not displaying properly, can someone tell me why?
(black and white is the array given/ the picture of the python app is what i wrote)
its only displaying the array given and not the bottom code i put, why?
![The code snippet is a Python program designed to construct and display a deck of 52 playing cards with each card's suit, name, and value. The steps and components of the code are explained below:
### Key Components
1. **Card Names and Values:**
- `dCardNames`: This list includes the names of the cards from '2' to '10', followed by 'J', 'Q', 'K', and 'A'.
- `dCardValues`: This parallel list assigns numerical values to each card, with face cards (J, Q, K) having a value of 10, and the Ace having a value of 11.
2. **Card Suits:**
- `dSuits`: This list contains the four suits available in a standard deck of cards: "Clubs", "Spades", "Diamonds", and "Hearts".
3. **Deck Construction:**
- `aCards`: A two-dimensional list initialized to store the card properties. It is structured with three rows intended to hold card names, suits, and values respectively for all 52 cards.
### Loop for Deck Creation
- **Outer Loop (`while i < 13`):**
- This loop iterates over the indices of card names and card values (from `0` to `12`).
- For each card name and value, it assigns these properties to the corresponding positions in `aCards` for each suit.
- **Assignments:**
- `aCards[0][i]`, `aCards[0][i + 13]`, `aCards[0][i + 26]`, `aCards[0][i + 39]` are used to assign the current card name to positions representing different suits.
- `aCards[1][i]`, `aCards[1][i + 13]`, etc., are used to assign suits to these card positions.
- `aCards[2][i]`, `aCards[2][i + 13]`, etc., assign the corresponding card values.
### Loop for Displaying Cards
- **Second Loop (`while i < 52`):**
- This loop iterates through the constructed deck of 52 cards.
- It prints out each card's name, suit, and value in a formatted string.
### Expected Output
For each card in the deck, the output will](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fac7f0f6e-6d20-42e1-81bb-7961a6d3ee59%2Feb71d177-0236-45f1-b152-a422e66fac6d%2Ft6t4sw_processed.png&w=3840&q=75)
Transcribed Image Text:The code snippet is a Python program designed to construct and display a deck of 52 playing cards with each card's suit, name, and value. The steps and components of the code are explained below:
### Key Components
1. **Card Names and Values:**
- `dCardNames`: This list includes the names of the cards from '2' to '10', followed by 'J', 'Q', 'K', and 'A'.
- `dCardValues`: This parallel list assigns numerical values to each card, with face cards (J, Q, K) having a value of 10, and the Ace having a value of 11.
2. **Card Suits:**
- `dSuits`: This list contains the four suits available in a standard deck of cards: "Clubs", "Spades", "Diamonds", and "Hearts".
3. **Deck Construction:**
- `aCards`: A two-dimensional list initialized to store the card properties. It is structured with three rows intended to hold card names, suits, and values respectively for all 52 cards.
### Loop for Deck Creation
- **Outer Loop (`while i < 13`):**
- This loop iterates over the indices of card names and card values (from `0` to `12`).
- For each card name and value, it assigns these properties to the corresponding positions in `aCards` for each suit.
- **Assignments:**
- `aCards[0][i]`, `aCards[0][i + 13]`, `aCards[0][i + 26]`, `aCards[0][i + 39]` are used to assign the current card name to positions representing different suits.
- `aCards[1][i]`, `aCards[1][i + 13]`, etc., are used to assign suits to these card positions.
- `aCards[2][i]`, `aCards[2][i + 13]`, etc., assign the corresponding card values.
### Loop for Displaying Cards
- **Second Loop (`while i < 52`):**
- This loop iterates through the constructed deck of 52 cards.
- It prints out each card's name, suit, and value in a formatted string.
### Expected Output
For each card in the deck, the output will

Transcribed Image Text:**Code Explanation and Output Visualization**
This Python script demonstrates how to create and shuffle a deck of cards. It uses lists and randomization to simulate a standard 52-card deck.
### Code Breakdown
1. **Card Initialization:**
- `aCardNames` stores the card values from 2 to 14, where 11 is Jack, 12 is Queen, 13 is King, and 14 is Ace.
- `dSuits` lists the four card suits: Clubs, Spades, Diamonds, and Hearts.
2. **Deck Creation:**
- A two-dimensional list `aCards` is created to represent each card in the deck with its suit and value.
3. **Deck Printing:**
- Before shuffling, the deck is printed with each card displayed as "Suit Value".
4. **Shuffling:**
- The deck is shuffled using a random swap algorithm from the `random` module.
5. **Post-Shuffling:**
- The shuffled deck is printed to show the new order of cards.
6. **Dealing Hands:**
- The first and second hands are printed, displaying the top two cards from the shuffled deck.
### Output Explanation
- **Initial Deck:**
- Displays each card in order, sorted by suit and then value.
- **After Shuffling:**
- Shows the deck in random order after shuffling, demonstrating the effectiveness of the randomization.
- **Dealt Hands:**
- The top two cards from the shuffled deck represent the first hand.
- The next two cards represent the second hand.
This code provides a basic foundation for understanding card deck manipulation in programming, suitable for educational purposes in an introductory programming course.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
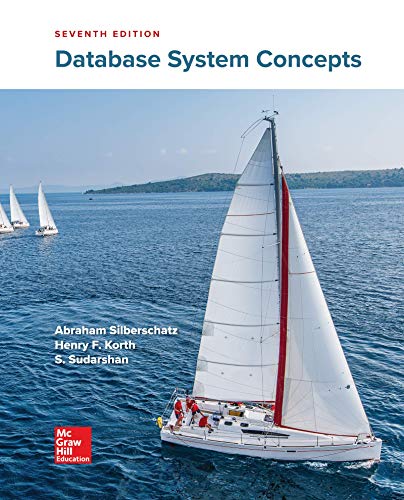
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
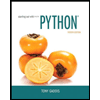
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
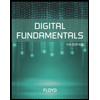
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
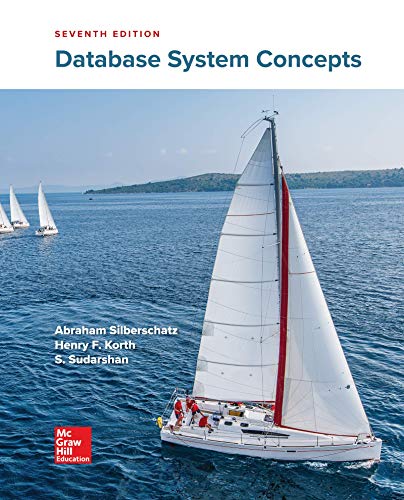
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
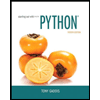
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
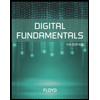
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
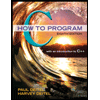
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
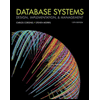
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
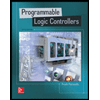
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education