Step 1: Read your files! You should now have 3 files. Read each of these files, in the order listed below. staticarray.h-contains the class definition for StaticArray, which makes arrays behave a little more like Python lists In particular, pay attention to the private variables. Note that the array has been defined with a MAX capacity but that is not the same as having MAX elements. Which variable indicates the actual number of elements in the array? staticarray.cpp contains function definitions for StaticArray. Right now, that's just the print member function. Pay attention to the print() function. How many elements is it printing? main.cpp-client code to test your staticarray class. • Note that the multi-line comment format (/.../) has been used to comment out everything in main below the first print statement. As you proceed through the lab, you will need to move the starting comment (/") to a different location. Other than that, no changes Step 2: Default constructor The first step is to write the default constructor, which should initialize the array to empty by specifying that currently there are no elements in it. You can get away with just one line of code inside this function. On a piece of paper, write down the prototype for this function. Then highlight the black bar below to double-check, and put the prototype into the public section of the class definition in [staticarray.h In staticarray.cpp, write the function definition. Remember, just one line! Ask for help if that doesn't make sense. At this point, you should compile and run the code. The output statements in main will tell you what to expect. Step 3: Append The next step is to define an append() member function, which should do 2 things: 1. If there's room in the array (how can you determine this?), append the given value to the end of the array 2. Return true if the value was appended and false if the array was full. On a plece of paper, write down the prototype for this function. Then highlight the black bar below to double-check, and put the prototype into the public section of the class definition in staticarray.h In staticarray.cpp, write the function definition. Alas, this is more than just one line. In main.cpp, put the code that tests append (up to the declaration of indices) back into your code. Compile and check the output to make sure everything is correct so far. Step 4: At The at() member function is going to work just like the brackets notation for arrays. It gets passed an index, and should return the element at that index. In other words, arr.at(0) is like our version of arr[0]. If the index is outside the bounds of the array, it should return -11111 (an arbitrary default value). The ability to do this is a nice benefit vs. regular arrays. Write the prototype for the at function, and then check below:


It looks like you have provided a detailed set of steps for a programming task, likely related to working with a custom array class called StaticArray in C++. I'll provide you with the steps and code snippets for each of the tasks you mentioned:
Step 1: Read your files
In this step, you need to read three files: staticarray.h, staticarray.cpp and main.cpp to understand the class definition and client code.
Step 2: Default constructor
In the StaticArray class in statciarray.h, add the following prototype for the default constructor in the public section:
1class StaticArray {
2public:
3 StaticArray(); // Default constructor prototype
4 // Other member function prototypes...
5};
6
In staticarray.cpp, define the default constructor as follows:
1StaticArray::StaticArray() {
2 // Initialize the array to empty
3}
4
Step 3: Append
Add a member function prototype for append to the StaticArray class in staticarray.h:
1class StaticArray {
2public:
3 // Other member function prototypes...
4 bool append(int value); // Append prototype
5};
6
In staticarray.cpp, define the append function. This function should append the value to the array if there's room and return true, otherwise return false.
1bool StaticArray::append(int value) {
2 // Append the value to the end of the array if there's room
3 // Return true if appended, false if the array is full
4}
5
Step by step
Solved in 3 steps

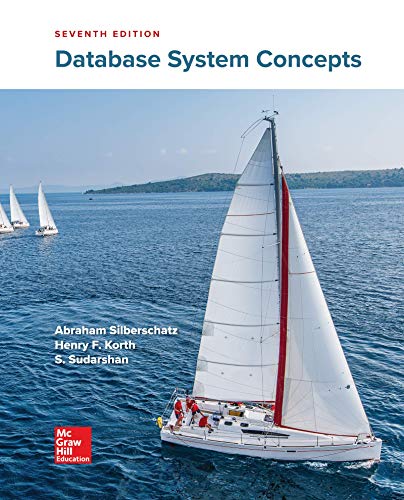
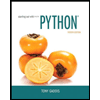
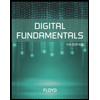
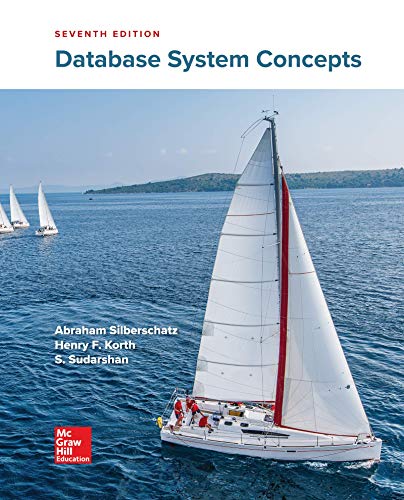
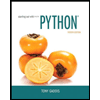
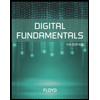
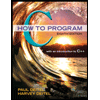
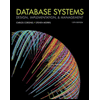
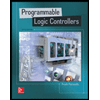