You need to design this class. It’ll hold all the FoodWastageRecord objects in memory (notice the has-a relationship between the FoodWastageTracker class and FoodWastageRecord class in the diagram above). It’ll allow the user to Add a FoodWastageRecord to the list of records in memory. IT SHOULD NOT add duplicate records. Return true if the record was added successfully, else return false. Delete a FoodWastageRecord from memory. Return true if the record was actually deleted. Return false if the record to be deleted wasn’t found. [NOTE: This is an extra credit requirement.] Get all FoodWastageRecord objects from memory Generate and return the FoodWastageReport All four of these will be the member functions of your class. food_wastage_tracker.hpp class FoodWastageTracker { public: bool AddRecord(const FoodWastageRecord &record); bool DeleteRecord(const FoodWastageRecord &record); const std::vector &GetRecords() const; FoodWastageReport GetFoodWastageReport() const; private: std::vector food_wastage_records_; }; food_wastage_tracker.cpp //what would be the implementation of the class?
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
You need to design this class.
It’ll hold all the FoodWastageRecord objects in memory (notice the has-a relationship between the FoodWastageTracker class and FoodWastageRecord class in the diagram above).
It’ll allow the user to
- Add a FoodWastageRecord to the list of records in memory. IT SHOULD NOT add duplicate records. Return true if the record was added successfully, else return false.
- Delete a FoodWastageRecord from memory. Return true if the record was actually deleted. Return false if the record to be deleted wasn’t found. [NOTE: This is an extra credit requirement.]
- Get all FoodWastageRecord objects from memory
- Generate and return the FoodWastageReport
All four of these will be the member functions of your class.
food_wastage_tracker.hpp
class FoodWastageTracker {
public:
bool AddRecord(const FoodWastageRecord &record);
bool DeleteRecord(const FoodWastageRecord &record);
const std::
FoodWastageReport GetFoodWastageReport() const;
private:
std::vector<FoodWastageRecord> food_wastage_records_;
};
food_wastage_tracker.cpp
//what would be the implementation of the class?

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

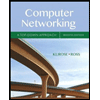
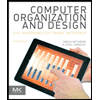
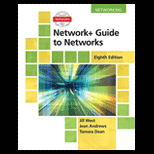
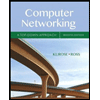
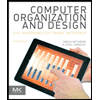
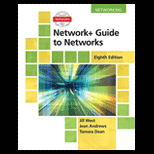
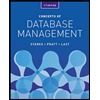
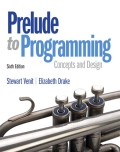
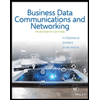