3 4 5{ 6 78 8 9 10 11 12 13 14 15 16 17 18 #include // provides size_t namespace CS3358_FA2022_A04_sequenceOf Num 19 20 21 22 23 24 25 26 27 28 29 30 31 class sequence { public: // TYPEDEFS and MEMBER SP2020 typedef double value_type; typedef size_t size_type; static const size_type CAPACITY = 10; // CONSTRUCTOR sequence(); // MODIFICATION MEMBER FUNCTIONS void start(); void end(); void advance(); }; void move_back(); void add(const value_type& entry); void remove_current(); // CONSTANT MEMBER FUNCTIONS size_type size() const; bool is item() const; value_type current() const; private: value_type data [CAPACITY]; size_type used; size_type current_index;
3 4 5{ 6 78 8 9 10 11 12 13 14 15 16 17 18 #include // provides size_t namespace CS3358_FA2022_A04_sequenceOf Num 19 20 21 22 23 24 25 26 27 28 29 30 31 class sequence { public: // TYPEDEFS and MEMBER SP2020 typedef double value_type; typedef size_t size_type; static const size_type CAPACITY = 10; // CONSTRUCTOR sequence(); // MODIFICATION MEMBER FUNCTIONS void start(); void end(); void advance(); }; void move_back(); void add(const value_type& entry); void remove_current(); // CONSTANT MEMBER FUNCTIONS size_type size() const; bool is item() const; value_type current() const; private: value_type data [CAPACITY]; size_type used; size_type current_index;
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Risking telling the obvious, you will need to make all necessary modifications to the files, including adapting the documentation and removing any parts that are irrelevant. I suggest that you pattern after the way the suggested book authors document a template class (such as for bag4) when adapting the documentation. |

Transcribed Image Text:MENSAGEM
3
4
5
6
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
#include <cstdlib> // Provides size_t
namespace main_savitch_6A
{
template <class Item>
class bag
{
public:
// TYPEDEFS and MEMBER CONSTANTS
typedef Item value_type;
typedef std::size_t size_type;
static const size_type DEFAULT_CAPACITY = 30;
// CONSTRUCTORS and DESTRUCTOR
bag(size_type initial capacity
bag(const bag& source);
~bag();
private:
// MODIFICATION MEMBER FUNCTIONS
size_type erase (const Item& target);
bool erase_one(const Item& target);
void insert (const Item& entry);
void operator =(const bag& source);
void operator +=(const bag& addend);
void reserve (size_type capacity);
// CONSTANT MEMBER FUNCTIONS
size_type count (const Item& target) const;
Item grab() const;
size_type size() const { return used; }
=
Item *data;
DEFAULT_CAPACITY);
// Pointer to partially filled dynamic array
// How much of array is being used
size_type capacity; // Current capacity of the bag
size_type used;
};
// NONMEMBER FUNCTIONS
template <class Item>
bag<Item> operator +(const bag<Item>& bl, const bag<Item>& b2);
![0-0
ANTONSEN
1
2
3
4
5
6
78
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
#ifndef SEQUENCE_H
#define SEQUENCE_H
#include <cstdlib> // provides size_t
namespace
CS3358_FA2022_A04_sequenceOfNum
{
}
class sequence
{
public:
// TYPEDEFS and MEMBER SP2020
typedef double value_type;
typedef size_t size_type;
static const size_type CAPACITY = 10;
// CONSTRUCTOR
sequence();
};
// MODIFICATION MEMBER FUNCTIONS
void start();
void end();
void advance();
void move_back();
void add(const value_type& entry);
void remove_current();
// CONSTANT MEMBER FUNCTIONS
size_type size() const;
bool is item() const;
value_type current() const;
private:
value_type data [CAPACITY];
size_type used;
size_type current_index;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2F19fb8af7-e35a-4c51-aaa2-6b9c4a647885%2F59ai4u5_processed.png&w=3840&q=75)
Transcribed Image Text:0-0
ANTONSEN
1
2
3
4
5
6
78
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
#ifndef SEQUENCE_H
#define SEQUENCE_H
#include <cstdlib> // provides size_t
namespace
CS3358_FA2022_A04_sequenceOfNum
{
}
class sequence
{
public:
// TYPEDEFS and MEMBER SP2020
typedef double value_type;
typedef size_t size_type;
static const size_type CAPACITY = 10;
// CONSTRUCTOR
sequence();
};
// MODIFICATION MEMBER FUNCTIONS
void start();
void end();
void advance();
void move_back();
void add(const value_type& entry);
void remove_current();
// CONSTANT MEMBER FUNCTIONS
size_type size() const;
bool is item() const;
value_type current() const;
private:
value_type data [CAPACITY];
size_type used;
size_type current_index;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
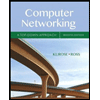
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
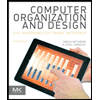
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
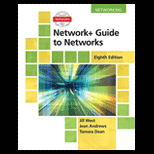
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
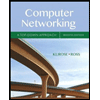
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
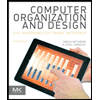
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
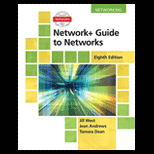
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
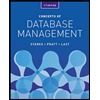
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
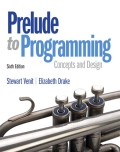
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
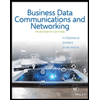
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY