2. Read all eight messages into Mailbox object from the file of "messages1.txt". The values for each line will be used to initialize each instance variable of the Message object, and then added into the Mailbox object. Important: Your must read the values in from the file using Scanner object, you are not allowed to initialize the objects manually. 3. Print out all messages using printAllMessages () method. Your output should look like Figure 2. Bluel: Terminal Window - 1 Solution Options Message list in the mailbox after loading from a file Message 1 From: Eric_A_Bially To: Zachary_B_Howard This is_test_message_1 Message 2 From: Brett_V_Bohorquez To: Marcus_R_McPartland This is_test_message_2 Message 3 From: Aron_I_Brewster To: Patrick_C_Nauman This is_test_message_3 Message 4 From: Joshua_J_Dennis To: Jacob_G_Neff This_is_test_message_4 Message 5 From: Stephen_M_Gentzler To: Kevin_A_0rlowski This is_test_message_5 Message 6 From: Adam_James_D_Geosits To: Oscar_J_Phillips This is_test_message_6 Message 7 From: Rudolf_C_Gouker To: Andy_R_Sayer This_is_test_message_7 Message 8 From: Zachary_B_Howard To: Craig_Smith This is_test_message_8 Figure 2: A screenshot of the program output 2. 4. Remove message at position 0 (index) using removeMessage (), and repeat it six times.



Hi... Please check below java code.
Mailbox.java
import java.util.List;
public class Mailbox {
private List<Message> all;
private int numOfMessages;
public List<Message> getAll() {
return all;
}
public void setAll(List<Message> all) {
this.all = all;
}
public int getNumOfMessages() {
return numOfMessages;
}
public void setNumOfMessages(int numOfMessages) {
this.numOfMessages = numOfMessages;
}
public Mailbox(List<Message> all, int numOfMessages) {
super();
this.all = all;
this.numOfMessages = numOfMessages;
}
public Message getMessage(int i){
return all.get(i-1);
}
public void removeMessages(int i){
all.remove(i-1);
}
public void printAllMessages(){
for(Message m:all){
System.out.println(m.getMessage());
}
}
}
Message.java
public class Message {
private String message;
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public Message(String message) {
super();
this.message = message;
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

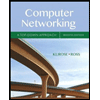
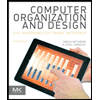
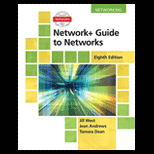
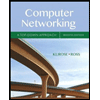
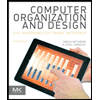
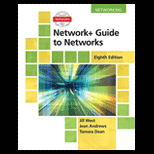
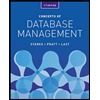
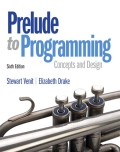
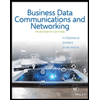