Write a Fraction class whose objects will represent fractions. #include using namespace std; class Fraction { public: int numerator; int denominator; void set(int n ,int d); void print(); Fraction multipliedBy(Fraction f); Fraction dividedBy(Fraction f); Fraction addedTo(Fraction f); Fraction subtract(Fraction f); bool isEqualTo(Fraction f); }; void Fraction::set(int n, int d) { numerator = n; denominator = d; } void Fraction::print() { cout << numerator << "/" << denominator; } Fraction Fraction::multipliedBy(Fraction f) { Fraction results; results.numerator = (this->numerator *f.denominator); results.denominator=this->denominator * f.numerator; return results; } Fraction Fraction::dividedBy(Fraction f) { Fraction results; results.numerator =(this->numerator * f.denominator); results.denominator=this->denominator * f.numerator; return results; } Fraction Fraction::addedTo(Fraction f) { Fraction results; results.numerator =(this->numerator * f.denominator) + (this->denominator * f.numerator); results.denominator=this->denominator * f.numerator; return results; } Fraction Fraction::subtract(Fraction f) { Fraction results; results.numerator = (this->numerator * f.denominator)- (this->denominator * f.numerator); results.denominator=this->denominator * f.numerator; return results; } bool Fraction::isEqualTo(Fraction f) { if ( this->numerator * f.denominator == this->denominator * f.numerator) return true; else return false; } int main() { Fraction f1; Fraction f2; Fraction result; f1.set(9, 8); f2.set(2, 3); cout << "The product of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.multipliedBy(f2); result.print(); cout << endl; cout << "The quotient of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.dividedBy(f2); result.print(); cout << endl; cout << "The sum of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.addedTo(f2); result.print(); cout << endl; cout << "The difference of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.subtract(f2); result.print(); cout << endl; if (f1.isEqualTo(f2)){ cout << "The two Fractions are equal." << endl; } else { cout << "The two Fractions are not equal." << endl; } } current output The product of 9/8 and 2/3 is 27/16 The quotient of 9/8 and 2/3 is 27/16 The sum of 9/8 and 2/3 is 43/16 The difference of 9/8 and 2/3 is 11/16 The two Fractions are not equal. Output is suppose to look like: The product of 9/8 and 2/3 is 18/24 The quotient of 9/8 and 2/3 is 27/16 The sum of 9/8 and 2/3 is 43/24 The difference of 9/8 and 2/3 is 11/24 The two Fractions are not equal. The product of 9/8 and 2/3 is 18/24 The quotient of 9/8 and 2/3 is 27/16 The sum of 9/8 and 2/3 is 43/24 The difference of 9/8 and 2/3 is 11/24 The two Fractions are not equal
Write a Fraction class whose objects will represent fractions.
#include <iostream>
using namespace std;
class Fraction
{
public:
int numerator;
int denominator;
void set(int n ,int d);
void print();
Fraction multipliedBy(Fraction f);
Fraction dividedBy(Fraction f);
Fraction addedTo(Fraction f);
Fraction subtract(Fraction f);
bool isEqualTo(Fraction f);
};
void Fraction::set(int n, int d)
{
numerator = n;
denominator = d;
}
void Fraction::print()
{
cout << numerator << "/" << denominator;
}
Fraction Fraction::multipliedBy(Fraction f)
{
Fraction results;
results.numerator = (this->numerator *f.denominator);
results.denominator=this->denominator * f.numerator;
return results;
}
Fraction Fraction::dividedBy(Fraction f)
{
Fraction results;
results.numerator =(this->numerator * f.denominator);
results.denominator=this->denominator * f.numerator;
return results;
}
Fraction Fraction::addedTo(Fraction f)
{
Fraction results;
results.numerator =(this->numerator * f.denominator) + (this->denominator * f.numerator);
results.denominator=this->denominator * f.numerator;
return results;
}
Fraction Fraction::subtract(Fraction f)
{
Fraction results;
results.numerator = (this->numerator * f.denominator)- (this->denominator * f.numerator);
results.denominator=this->denominator * f.numerator;
return results;
}
bool Fraction::isEqualTo(Fraction f)
{
if ( this->numerator * f.denominator == this->denominator * f.numerator)
return true;
else
return false;
}
int main()
{
Fraction f1;
Fraction f2;
Fraction result;
f1.set(9, 8);
f2.set(2, 3);
cout << "The product of ";
f1.print();
cout << " and ";
f2.print();
cout << " is ";
result = f1.multipliedBy(f2);
result.print();
cout << endl;
cout << "The quotient of ";
f1.print();
cout << " and ";
f2.print();
cout << " is ";
result = f1.dividedBy(f2);
result.print();
cout << endl;
cout << "The sum of ";
f1.print();
cout << " and ";
f2.print();
cout << " is ";
result = f1.addedTo(f2);
result.print();
cout << endl;
cout << "The difference of ";
f1.print();
cout << " and ";
f2.print();
cout << " is ";
result = f1.subtract(f2);
result.print();
cout << endl;
if (f1.isEqualTo(f2)){
cout << "The two Fractions are equal." << endl;
} else {
cout << "The two Fractions are not equal." << endl;
}
}
current output
The product of 9/8 and 2/3 is 27/16
The quotient of 9/8 and 2/3 is 27/16
The sum of 9/8 and 2/3 is 43/16
The difference of 9/8 and 2/3 is 11/16
The two Fractions are not equal.
Output is suppose to look like:
The product of 9/8 and 2/3 is 18/24 The quotient of 9/8 and 2/3 is 27/16 The sum of 9/8 and 2/3 is 43/24 The difference of 9/8 and 2/3 is 11/24 The two Fractions are not equal.
The product of 9/8 and 2/3 is 18/24 The quotient of 9/8 and 2/3 is 27/16 The sum of 9/8 and 2/3 is 43/24 The difference of 9/8 and 2/3 is 11/24 The two Fractions are not equal.

Step by step
Solved in 2 steps with 1 images

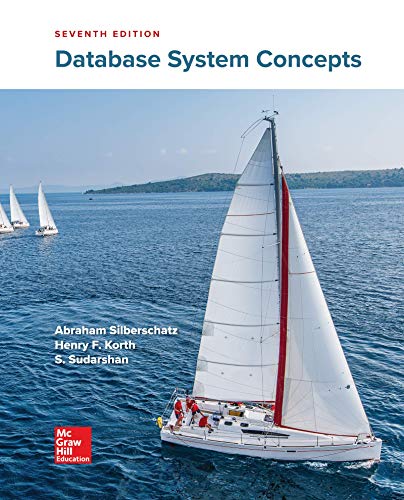
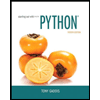
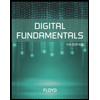
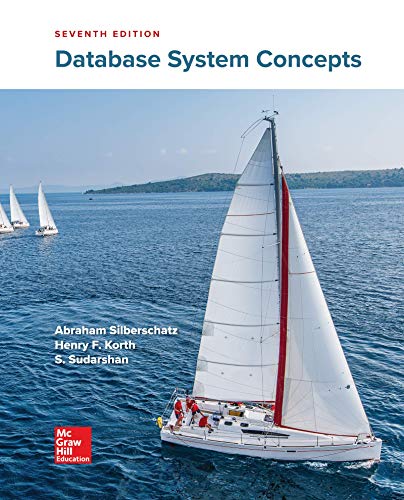
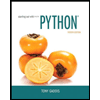
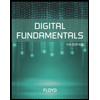
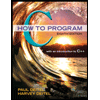
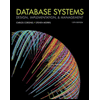
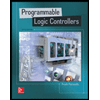