Write a superclass called Shape(as shown in the class diagram), which contains: Two instance variables color(String) and filled (boolean). Two constructors: a no-arg (no-argument) constructor that initializes the colorto "green" and filled to true, and a two-argument constructor that initializes the color and filled to the parameters values. Getter and setter for all the instance variables. By convention, the getter for a booleanvariable filled is called isFilled() (instead of getFilled() for all the other types). A toString()method that returns "A << filled/Not filled >> Shape with << green >>". Methods getArea() andgetPerimeter() both reurn 0. // We can not write the implementation of getArea() and getPerimeter() until we know the specific shape. There are two subclasses of Shapecalled Circle and Rectangle, as shown in the class diagram. Ignore the Circle class. The Rectangle class contains: Two instance variables width(double) and length (double). Three constructors as shown. The no-arg constructor initializes the widthand length to 0. All the constructors in Rectangle class should call the appropriate super-class (shape) constructors. Getter and setter for all the instance variables. Override methods getArea() -> length*widthand getPerimeter() -> 2*length + 2*width. Override the toString()method inherited, to return "A Rectangle with width=<< xxx >> and length= << zzz >>, which is a subclass of << yyy >>", where yyy is the output of the toString() method from the superclass. Write a class called Square, as a subclass of Rectangle. Square has no instance variable, but inherits the instance variables width and length from its superclass Rectangle. Note that a square is a rectangle with same width and height, therefore, whenever the length is changed, width should also be changed to the same value for maintaining the square geometry and vice versa. Provide the appropriate constructors (as shown in the class diagram). All the constructors in the Square class should call the appropriate super-class (Rectangle) constructors. Use super public Square(double side) { super(side, side); // Call superclass Rectangle(double, double) } Override the toString()method to return "A Square with side=xxx, which is a subclass of yyy", where yyy is the output of the toString() method from the superclass. Do you need to override the getArea()and getPerimeter()? Try them out. Override the setLength()and setWidth() to change both the width and length, so as to maintain the square geometry. The Test Class: Create 6 objects of rectangle and square (3 each) using each type of constructors in these classes using an array of Shape reference variables. Observe the order of constructor calling according to messages printed by your constructors. Display the state of each object via implicit call to toString. Use enhanced for to display the state of objects along with corresponding area and perimeter. Observation Experiments: Remove the no-argument constructor of shape class. Rerun your test class. Is there any error? Why? Remove all the constructors from each class, except the two-argument constructor from the shape class and no-argument constructors from the Rectangle and Square classes. Is there any error? Why? Write your observations as comments at the end of your test class of your program.
Attaching the picture of class diagram
Write a superclass called Shape(as shown in the class diagram), which contains:
- Two instance variables color(String) and filled (boolean).
- Two constructors: a no-arg (no-argument) constructor that initializes the colorto "green" and filled to true, and a two-argument constructor that initializes the color and filled to the parameters values.
- Getter and setter for all the instance variables. By convention, the getter for a booleanvariable filled is called isFilled() (instead of getFilled() for all the other types).
- A toString()method that returns "A << filled/Not filled >> Shape with << green >>".
- Methods getArea() andgetPerimeter() both reurn 0. // We can not write the implementation of getArea() and getPerimeter() until we know the specific shape.
There are two subclasses of Shapecalled Circle and Rectangle, as shown in the class diagram. Ignore the Circle class.
The Rectangle class contains:
- Two instance variables width(double) and length (double).
- Three constructors as shown. The no-arg constructor initializes the widthand length to 0. All the constructors in Rectangle class should call the appropriate super-class (shape) constructors.
- Getter and setter for all the instance variables.
- Override methods getArea() -> length*widthand getPerimeter() -> 2*length + 2*width.
- Override the toString()method inherited, to return "A Rectangle with width=<< xxx >> and length= << zzz >>, which is a subclass of << yyy >>", where yyy is the output of the toString() method from the superclass.
Write a class called Square, as a subclass of Rectangle. Square has no instance variable, but inherits the instance variables width and length from its superclass Rectangle.
Note that a square is a rectangle with same width and height, therefore, whenever the length is changed, width should also be changed to the same value for maintaining the square geometry and vice versa.
- Provide the appropriate constructors (as shown in the class diagram). All the constructors in the Square class should call the appropriate super-class (Rectangle) constructors. Use super
public Square(double side) {
super(side, side); // Call superclass Rectangle(double, double)
}
- Override the toString()method to return "A Square with side=xxx, which is a subclass of yyy", where yyy is the output of the toString() method from the superclass.
- Do you need to override the getArea()and getPerimeter()? Try them out.
- Override the setLength()and setWidth() to change both the width and length, so as to maintain the square geometry.
The Test Class:
- Create 6 objects of rectangle and square (3 each) using each type of constructors in these classes using an array of Shape reference variables.
- Observe the order of constructor calling according to messages printed by your constructors.
- Display the state of each object via implicit call to toString. Use enhanced for to display the state of objects along with corresponding area and perimeter.
Observation Experiments:
- Remove the no-argument constructor of shape class. Rerun your test class. Is there any error? Why?
- Remove all the constructors from each class, except the two-argument constructor from the shape class and no-argument constructors from the Rectangle and Square classes. Is there any error? Why?
Write your observations as comments at the end of your test class of your program.


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

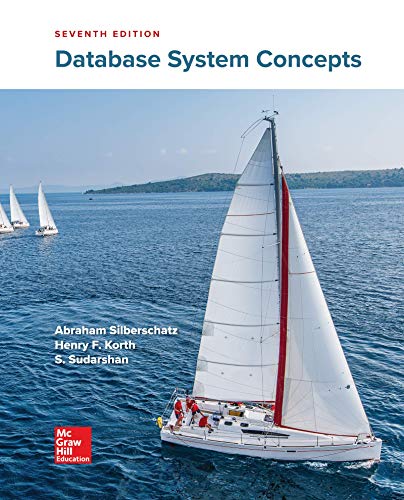
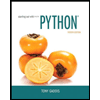
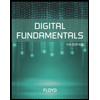
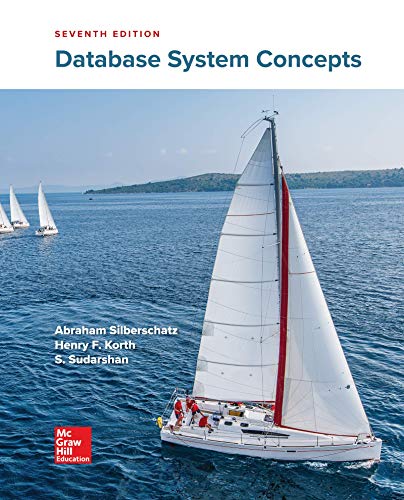
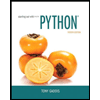
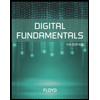
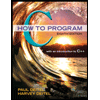
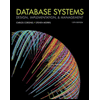
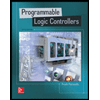