Where do I put the Input.txt file for the below program //Adding the header files #include #include #include #include #include //Using namespace using namespace std; //Defining the main() int main() { //Declaring the variable string file; string name; double sc; double total; double grade; string des; //Getting input from the user cout << "Enter the input file name: "; cin >> file; //Creating file object ifstream infile; //Opening the input file infile.open(file); //Checking the file if (!infile) { cout << file << "File cannot be opened!" << endl; exit(1); } infile >> name; //Reading the file while (infile) { //Getting data from file infile >> sc; infile >> total; //Computing the grade grade = sc / total * 100; //Checking grade based on this printing the status for student if (grade > 90) des = "Excellent"; else if (grade > 80) des = "Well Done"; else if (grade > 70) des = "Good"; else if (grade >= 60) des = "Need Improvement"; else des = "Fail"; //Printing the output cout << name << " " << setprecision(0) << fixed << round(grade) << "% " << setprecision(5) << fixed << (grade * 0.01) << " " << des << endl; infile >> name; } //Closing the file infile.close(); return 0; }
Where do I put the Input.txt file for the below
//Adding the header files
#include <iostream>
#include <fstream>
#include <iomanip>
#include <string>
#include <cmath>
//Using namespace
using namespace std;
//Defining the main()
int main()
{
//Declaring the variable
string file;
string name;
double sc;
double total;
double grade;
string des;
//Getting input from the user
cout << "Enter the input file name: ";
cin >> file;
//Creating file object
ifstream infile;
//Opening the input file
infile.open(file);
//Checking the file
if (!infile)
{
cout << file << "File cannot be opened!" << endl;
exit(1);
}
infile >> name;
//Reading the file
while (infile)
{
//Getting data from file
infile >> sc;
infile >> total;
//Computing the grade
grade = sc / total * 100;
//Checking grade based on this printing the status for student
if (grade > 90)
des = "Excellent";
else if (grade > 80)
des = "Well Done";
else if (grade > 70)
des = "Good";
else if (grade >= 60)
des = "Need Improvement";
else
des = "Fail";
//Printing the output
cout << name << " " << setprecision(0) << fixed << round(grade) << "% " << setprecision(5) << fixed << (grade * 0.01) << " " << des << endl;
infile >> name;
}
//Closing the file
infile.close();
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

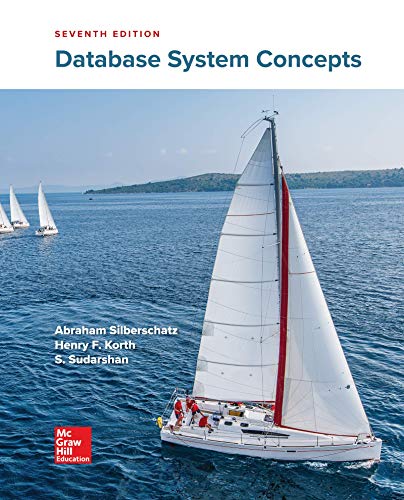
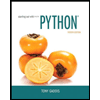
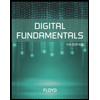
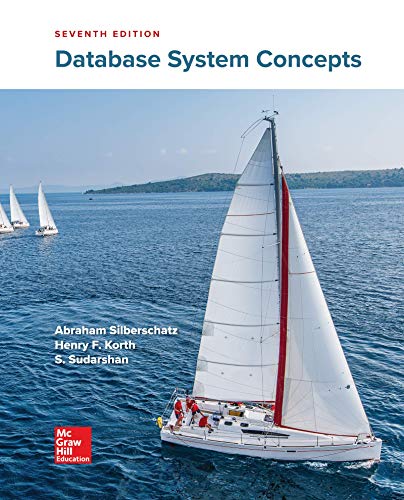
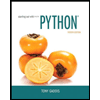
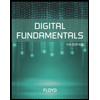
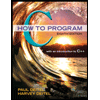
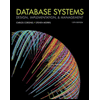
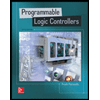