namespace
#include "Bank.h"
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
int main() {
Account** accounts = new Account * [10];
ifstream infile;
infile.open("bank2.txt");
if ( !infile )
cerr << "File cannot be opened" << endl;
else
cout << "File Opened" << endl;
string operationType;
int totalAccount = 0;
while (infile >> operationType) {
if (operationType == "Saving") {
int id;
double initialBalance, minimumBalance, annualInterestRate;
infile >> id >> initialBalance >> minimumBalance >> annualInterestRate;
Saving* savingAccount = new Saving();
savingAccount->setInterest(annualInterestRate);
savingAccount->setMinBalance(minimumBalance);
accounts[totalAccount] = savingAccount;
accounts[totalAccount]->setID(id);
accounts[totalAccount]->setBalance(initialBalance);
totalAccount++;
cout << "Saving account number " << totalAccount << " created" << endl;
cout << "with an initial balance of $" << accounts[totalAccount - 1]->getBalance() << endl;
}
else if (operationType == "Checking") {
int id;
double initialBalance, minimumBalance, monthlyFees;
infile >> id >> initialBalance >> minimumBalance >> monthlyFees;
Checking* checkingAccount = new Checking();
checkingAccount->setMonthlyFee(monthlyFees);
checkingAccount->setMinBalance(minimumBalance);
accounts[totalAccount] = checkingAccount;
accounts[totalAccount]->setID(id);
accounts[totalAccount]->setBalance(initialBalance);
totalAccount++;
cout << "Checking account number " << totalAccount << " created" << endl;
cout << "with an initial balance of $" << accounts[totalAccount - 1]->getBalance() << endl;
}
else if (operationType == "Deposit") {
int id;
double amount;
int pos;
bool isFound = false;
infile >> id >> amount;
for (int i = 0; i < totalAccount; i++) {
if (accounts[i]->getID() == id) {
isFound = true;
accounts[i]->deposit(amount);
pos = i;
}
}
if (!isFound) {
cout << "Invalid Account Number - " << id << endl;
}
else {
cout << "Deposited $" << amount << " into account #" << id << endl;
cout << "current balance is $" << accounts[pos]->getBalance() << endl;
}
}
else if (operationType == "Withdraw") {
int id;
double amount;
int pos;
infile >> id >> amount;
bool isFound = false;
for (int i = 0; i < totalAccount; i++) {
if (accounts[i]->getID() == id) {
isFound = true;
accounts[i]->withdraw(amount);
pos = i;
}
}
if (!isFound) {
cout << "Invalid Account Number - " << id << endl;
}
else {
cout << "Withdraw $" << amount << " from account #" << id << endl;
cout << "current balance is $" << accounts[pos]->getBalance() << endl;
}
}
else if (operationType == "Balance") {
int id;
infile >> id;
int pos;
bool isFound = false;
for (int i = 0; i < totalAccount; i++) {
if (accounts[i]->getID() == id) {
isFound = true;
cout << "current balance in account #" << id << " is $" << accounts[i]->getBalance() << endl;
pos = i;
}
}
if (!isFound) {
cout << "Invalid Account Number - " << id << endl;
}
}
else if (operationType == "Close") {
cout << "End of month processing complete" << endl;
for (int i = 0; i < totalAccount; i++) {
accounts[i]->closeMonth();
}
}
else if (operationType == "Report") {
for (int i = 0; i < totalAccount; i++) {
cout << accounts[i]->accountString() << endl;
}
}
else {
cout << "Unrecognised command - " << operationType << endl;
string dummyLine;
getline(infile, dummyLine);
}
}
infile.close();
}



Step by step
Solved in 2 steps

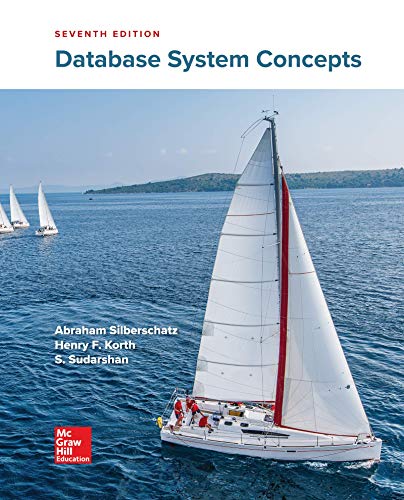
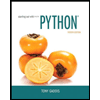
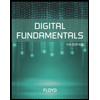
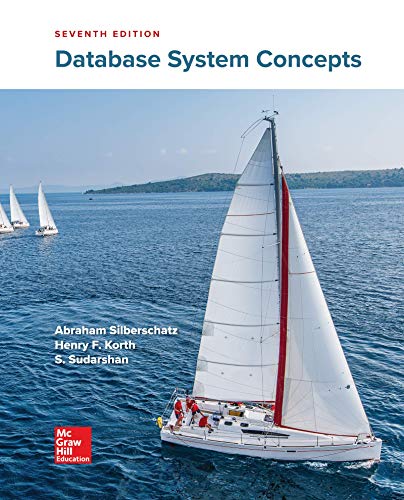
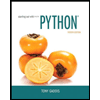
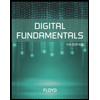
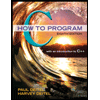
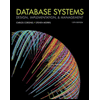
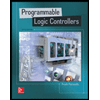